Related Blogs
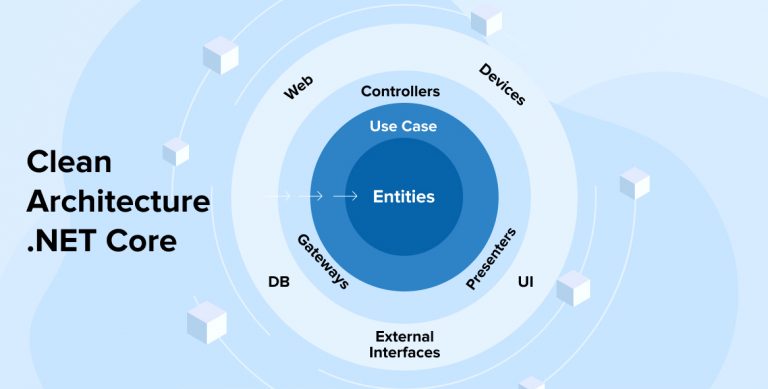
Most traditional .NET applications are deployed as a single unit. All of these applications run on the same IIS application domain and execute as web applications. It is still recommended to logically separate business applications into several layers and deploy them in this single unit. Monolithic applications are completely isolated in terms of their behavior. To perform operations, it may interact with other services and data stores. However, most applications are deployed as a single package. But in general, applications are divided into layers. One layer is dependent on the other layer. When there are dependencies among parts of an application, it can be difficult to test one part of it.
Clean Architecture is the solution to this dependency issue. In contrast to general architecture, Clean Architecture does not depend on frameworks, user interfaces, or databases. Generally, an application has 3 kinds of layers: UI layer, Business Logic Layer, and Data Access Layer but Clean architecture includes Application Core, Infrastructure and UI. It is possible to test or change a user interface or database without having to change the rest of the system.
Our team of .Net developers has helped us to draft this blog to help you to understand the importance of clean architecture and maintaining one will eliminate the loopholes coming from an erroneous app development environment. To start with, let us check the different types of architectures in ASP.NET core and then understand the clean architecture.
1. Common Architectures in ASP.NET Core
Having a good architecture is a key to building an application. Different kinds of architecture are always there to use, which have the same objectives i.e. Separation of code. That can be done by dividing an application into layers.
Two types are categorized in ASP.NET Core Common Architecture:
1.1 Traditional “N-Layer” Architecture
- It has layers such as UI, BLL (Business Logic Layer), and DAL (Data Access Layer).
- Presentation of any page will be a part of the UI Layer. In the UI layer, users can make requests. BLL interacts with these requests.
- It depends on DAL. BLL holds the logic in the application. BLL calls DAL for data access requests.
- DAL holds the data access to all the implementation details. DAL depends on the existence of the Database.
- One key disadvantage of this architecture is that the compile time dependencies run from the top to bottom.The main User Interface layer depends upon the BLL and this BLL is dependent on the DAL. This means that the BLL, which holds the most important logic in the application, depends upon the data access layer.
- So, business logic testing is much more difficult as it always requires a test database.
- As a solution, dependency inversion principle is used.
1.2 Clean Architecture
- In this type, domain and Application layers remain at the core of the design, which is called the center of the design.
- Unlike Traditional “N-Layer” architecture, the clean architecture is independent of the database, UI layer, Framework, and several other external agencies.
- The clean architecture puts business logic & application model at the center of the application.
- It has inverted dependency, the infrastructure and implementation details will depend on the application core.
Now, let’s understand Clean Architecture in detail.
2. Clean Architecture
Robert C. Martin created the clean architecture and promoted it on his blog. Clean architecture refers to software architecture. Instead of relying on data access for business logic, the application core is responsible for infrastructure and implementation details. In the application core, we can define abstractions, or interfaces, to achieve this functionality. There are various types defined at the infrastructure layer that can be used to implement clean architecture.
As we already know that there is no dependency on frameworks in the clean architecture. It is not mandated to have a library of feature-rich software to build this architecture. It is highly testable, we can test applications without the UI or any framework dependency. With a clean architecture, business logic and application models are placed at the center of the design. In a clean architecture, modules at the high level or their abstractions are not dependent on low level modules.
2.1 Benefits of Clean Architecture
- Clean architecture provides you with a cost-effective methodology that helps you to develop quality code with better performance and is easy to understand.
- Independent of database, frameworks & presentation layer. One can change the user interface at any time without changing the rest of the system or business logic.
- Unlike Oracle or SQL Server database, business rules are not bound to any specific database, so one can use BigTable, MongoDB, CouchDB, or something else to implement the business rules. Also, clean architecture doesn’t depend on the existence of some libraries, which are having features of laden software.
- Clean architecture is highly testable, one can check business rules without any other external elements or even without the UI, Web server, or Database.
- Clean architecture is independent of any external agency, one can say that business rules are unknown to the outside world.
2.2 Clean Architecture Layers
There are 3 layers in the Clean Architecture: The domain layer or infrastructure layer is in the center and surrounded by the application core layer and the outer layer consists of user interfaces.
Compile-time dependencies are represented by solid arrows & runtime-only dependency is represented by dashed arrows in the following diagram. It will become very easy to write automated unit tests & the reason is that the Application Core doesn’t depend on Infrastructure. The applications where UI projects, Infrastructure & Application Core runs as a single unit, are called monolithic applications.
There are interfaces defined in the application core. At the compile time the UI layer works with them. The Infrastructure layer will define implementation types, & UI layer shouldn’t know about those types. However, when the app executes, it requires these implementation types. Implementation types need to be present and must be wired up with the Application’s Core interfaces. Also, with the help of dependency injection, we shall be able to do the above mentioned things.
The given image will show how clean code works for any normal interface.
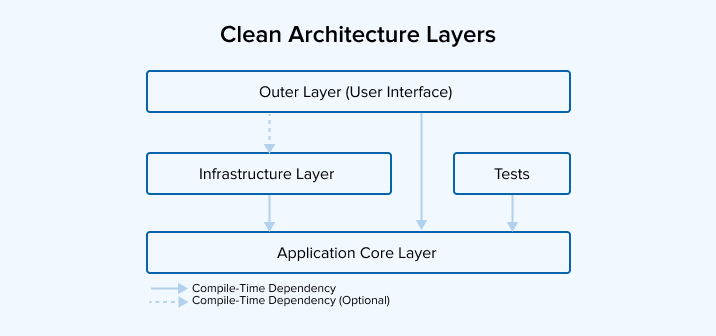
3. Clean Architecture in .NET Core
The in-built use of ASP.NET Core and dependency injection support makes this structure an ideal way to design non-monolithic applications. As the Application layer does not depend on infrastructure, automated unit tests become very easy.
There are three parts in this section: the core part, Infrastructure & Web.
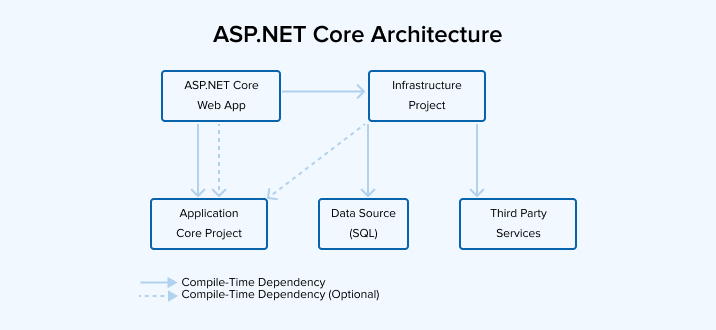
- What belongs to ASP.NET Core Web(Domain Layer)?
- One can have all of ASP.NET Core & ASP.NET Core MVC Types or some of these in addition to any DTO types such as ViewModel or API Model etc.
- ASP.NET Core Web App may include controllers, Models, ViewModels, ASP.NET Core Identity, Response Caching Filters, Model Validation Filters or several other filters, etc.
- What about Application Core?
- All of the Domain ModelTypes belong to Application Core.
- Interfaces, Business Services, Domain Events, Value Objects, POCO Entities, Application Exceptions, Aggregates, Specifications etc will be a part of Application Core Project.
- What resides in the Infrastructure?
- The communications out of the app’s process belong to Infrastructure.
- Like if someone wants to communicate out of the app, through an SMS so that can also be done through the app.
- There will be Redis Cache Service, Azure Service bus Accessor, InMemory Data Cache, EF DbContext or SMS Service, Email Service, Other API Clients in infrastructure project.
- Here in the database, we can use database link SQL to be a part Data Source.
- Third-Party Services can be GitHub API, SendGrid API, Twilio API.
Organizing code in .NET Core clean architecture
Clean architecture is layered architecture consisting Application, Infrastructure and User Interface layers. Every layer has its own responsibilities to follow. Layers contain their types which will be mentioned in detail further.
3.1 Application Layer
Application core layer contains all the business logic along with entities, domain services and interfaces. It should have minimal dependencies on databases, instead we expect it should have interface and domain model types including entities, value objects aggregates. There are domain services which will have logic in them that affects multiple entities or aggregates.
Also custom exceptions should be in the application layer. It is helpful to know when exceptions occur. Apart from that, the application layer contains domain events, event handlers and specifications which are the way to encapsulate the query into a class.
For services to pass the data to higher layers, there should be Data Transfer Objects (DTOs). There can be validators in the core project like “fluentvalidation” which is used to validate the objects which are passed in the controller. Also ”enums” can be there along with custom guard clauses.
Types of Application Layer
Application core include interfaces, domain services, specifications, custom exceptions, protection clauses, domain events, handlers, and more.
3.2 Infrastructure Layer
It depends on the Application layer for the business logic. It implements an interface from the application layer and provides functionality for accessing external systems. It includes repositories that talk to the database, the Entity Framework DbContext and necessary migration files for the communication with the database. The infrastructure layer also contains API Clients, File System, Email/SMS and System Clock. Also, there are services which implement interfaces defined in Application layer therefore, all interfaces primarily go into application core but some interfaces will go in infrastructure. So basically it works on the database and external API calls.
Types of Infrastructure Layer
Types of Infrastructure layers are
- EF Core DbContext
- Data access implementation types like Repositories, Web services, and file logger.
3.3 User Interface Layer
This layer is the access factor to the application from the user’s perspective. It contains the MVC stuff like Controller, Views or Razor Pages, ViewModels, etc. It can have custom model binders, custom filters, and custom middleware. Tag helpers are also part of these layers. There are some built-in tag helpers like Html tag helpers, image tag helpers, label tag helpers, etc. Model binding or custom model binding is also part of the UI layer.
Types of UI Layer
UI Layer has Controllers, Views/Razor Pages, ViewModels, Custom middlewares, Custom filters, and Startup class.
4. Conclusion
Clean Architecture helps to organize applications from moderate to high complexity. It separates the dependencies in a way that business logic and application’s domain keep isolated. ASP.NET Core works perfectly with the Clean Architecture method, as long as the original solution structure is set correctly. One can break down software into layers according to a distorted dependence and can have an internal system checkable. The Clean Architecture creates the application which is independent of UI Layer, Database, Framework, or any other external sources like software laden libraries, that will be easy to testify. You can also use this clean architecture solution template, a .NET core project template, available on github to ensure your application development is going on the right track.
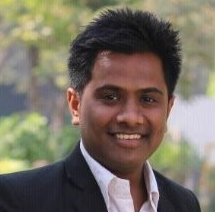
Vishal Shah
Vishal Shah has an extensive understanding of multiple application development frameworks and holds an upper hand with newer trends in order to strive and thrive in the dynamic market. He has nurtured his managerial growth in both technical and business aspects and gives his expertise through his blog posts.
Subscribe to our Newsletter
Signup for our newsletter and join 2700+ global business executives and technology experts to receive handpicked industry insights and latest news
Build your Team
Want to Hire Skilled Developers?
Comments
Leave a message...