Related Blogs
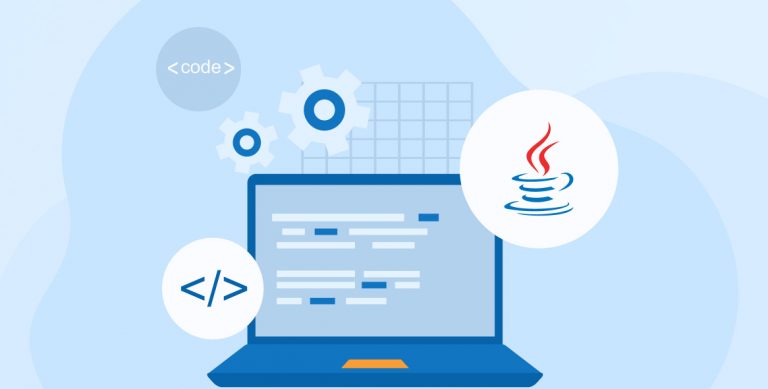
Advancement in technology occurs every single day. Lambda expression in Java is a new additional extension in Java 8. With domain-expertise and experience in Java, As Java development company we have prepared this insightful blog on the use of Lambda expressions with functional interfaces and instances that will make users well-versed with Java lambda expressions.
We do not have to define the Lambda expression. It is an anonymous method that does not execute itself. It can only be implemented if you define a functional interface and run that method. Lambda can be considered as methods that are implemented in the body section of the method.
Lambda expression was one of the most requested features which were made available since Java SE 8. A Java Lambda expression is a function that does not belong to any class. Java lambda expressions are commonly used to implement simple event listeners.
These lambda expressions are effective methods to represent one method interface using an expression. With its multi-functional collection library, it is easy to iterate, filter, and extract data and implement it.
What is Functional Interface?
An interface that contains only one abstract method called functional interface. It shows only one functionality. In the latest version of Java 8, lambda expressions are used to implement functional interfaces.
Syntax
As given in definition, a functional interface can have only one abstract method, but it can have one or more default methods as well as static methods.
Java Lambda expression syntax,
(argument-list) -> {body}. |
(argument-list) -> {body}.
There are three components in Lambda expression,
- The first component is surrounded by parentheses is an argument list, which can have none, one, or multiple arguments.
- The second component is Arrow-Token which can be used to connect the list of arguments with the body of expression.
- The third component is the method body, which comprises expressions and statements that are used for lambda expressions.
Before the Lambda expression, we were using anonymous interface implementations.
Without Lambda:
interface Square { public void size(); } public class LambdaExpressionExample { public static void main(String[] args) { int sideLength=2; Square s = new Square() { public void size() { System.out.println("All sides are "+ sideLength +" meter length."); } }; s.size(); } } |
interface Square { public void size(); } public class LambdaExpressionExample { public static void main(String[] args) { int sideLength=2; Square s = new Square() { public void size() { System.out.println("All sides are "+ sideLength +" meter length."); } }; s.size(); } }
Using Lambda Expression, we can replace some of the code in the above example as given below:
Square s = () { System.out.println("All sides are "+ sideLength +" meter length."); }; |
Square s = () { System.out.println("All sides are "+ sideLength +" meter length."); };
As you can see in the code above, we do not have to create an anonymous object for the method in the functional interface.
Lambda expressions and anonymous interface implementations, both are the same still there are a few differences. One of the differences is that anonymous interface implementation has a member variable, but lambda expressions can’t have member variables.
Lambda Parameters
Since Java lambda expressions are just methods, lambda expressions can also take parameters just like methods. These parameters must be the same count and same data type as the parameters of the method in the single method interface.
Zero Parameter
- If the matching method from the interface does not have any parameter then you can write a Lambda expression like,
() -> { System.out.println("method body"); };
() -> { System.out.println("method body"); };
- If the method body has only one line then you can also emit curly braces.
() -> System.out.println("method body");
() -> System.out.println("method body");
Single Parameter
- If interface method has one parameter then Lambda expression will be like this,
(param) -> {System.out.println("One parameter: " + param);};
(param) -> {System.out.println("One parameter: " + param);};
- For one parameter, you can also omit parentheses,
param -> System.out.println("One parameter: " + param);
param -> System.out.println("One parameter: " + param);
Multiple Parameter
- If the method you match with your Java lambda expression takes multiple parameters, the parameters need to be listed in parentheses. Here is how that looks in Java code:
(p1, p2) { System.out.println("Multiple parameters: " + p1 + ", " + p2); };
(p1, p2) { System.out.println("Multiple parameters: " + p1 + ", " + p2); };
Accessible Variables
A Java lambda expression is capable of accessing variables declared outside the lambda function body under certain circumstances.
Java lambda expression can access the following types of variables:
- Local variables
- Instance variables
- Static variables
Local Variables
- A Lambda expression can access the value of a local variable declared outside the lambda body if and only if the variable is “effectively final” or declared as final.
- If you try to change the value of that variable the compiler would give you an error about the reference to it from inside the lambda body.
Effectively Final
- It means that the variable is not declared as final but its value has never been changed once it is assigned.
Instance Variables
- A Lambda expression can also access an instance variable. Java Developer can change the value of the instance variable even after its defined and the value will be changed inside the lambda as well.
Static Variables
- A Lambda expression can also use static variables. Because a static variable is accessible from everywhere in a Java application. Static variable can be changed once it is used in Lamda expression by the Java developer.
Method References
As you saw until now, lambda expression calls another method using the parameters that passed to the lambda expression. There is a shorter way to express the method call.
For example,
MyInterface myInterface = (str) -> { System.out.println(str); }; |
MyInterface myInterface = (str) -> { System.out.println(str); };
- You have seen a short way to represent this is,
MyInterface myInterface = str -> System.out.println(str);<br>
MyInterface myInterface = str -> System.out.println(str);<br>
- Now, you have a shorter way to represent method call in Lambda like this,
MyInterface myInterface = System.out<strong>::</strong>println;<br>
MyInterface myInterface = System.out<strong>::</strong>println;<br>
- Here the double colon (::) is a signal to the Java compiler that this is a method reference. The method defined after the double colon is referred to as a method reference.
- Whatever class or object that has the referenced method defines before the double colon.
Conclusion
Undoubtedly, there are many advantages of Lambda expressions that we came across in this elaborate blog such as it reduces lines of code, sequential and parallel execution support, passing behavior into methods, and higher efficiency.
In this article, we have learned about functional interfaces, using it, how one can write lambda expressions, and different types of ways to define lambda expressions using different parameters like zero, one, two, or more parameters. Then, we also learned various variables that are accessible from a local lambda expression, instance, and static variables. After this, we also found a shorter way to express lambda expression using the double colon (::) which is called as a method reference.
Overall, now we are well-versed with using lambda expressions. We can reduce code lines without creating anonymous objects. Using Lambda, we can also define methods in the functional interface that will reduce memory consumption and accelerate productivity.
More Post on Java:
Is AWS Lamda is server side component?
Microservices Implementation in Java
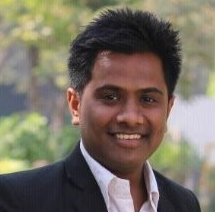
Vishal Shah
Vishal Shah has an extensive understanding of multiple application development frameworks and holds an upper hand with newer trends in order to strive and thrive in the dynamic market. He has nurtured his managerial growth in both technical and business aspects and gives his expertise through his blog posts.
Subscribe to our Newsletter
Signup for our newsletter and join 2700+ global business executives and technology experts to receive handpicked industry insights and latest news
Build your Team
Want to Hire Skilled Developers?
Comments
Leave a message...