Related Blogs
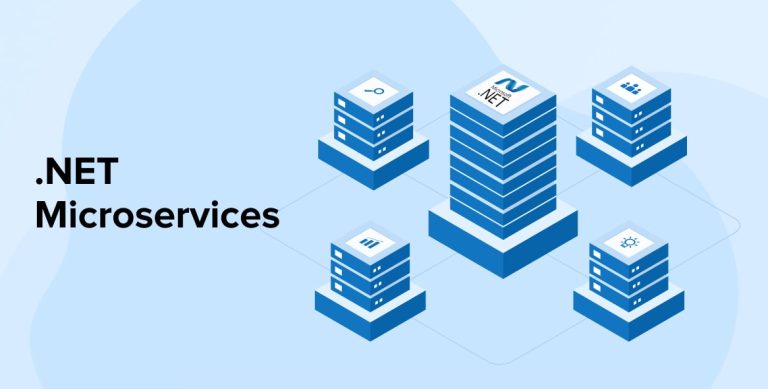
Key Takeaways on .Net Microservices
- The microservices architecture is increasingly being favoured for the large and complex applications based on the independent and individual subsystems.
- Container-based solutions offer significant cost reductions by mitigating deployment issues arising from failed dependencies in the production environment.
- With Microsoft tools, one can create containerized .NET microservices using a custom and preferred approach.
- Azure supports running Docker containers in a variety of environments, including the customer’s own datacenter, an external service provider’s infrastructure, and the cloud itself.
- An essential aspect of constructing more secure applications is establishing a robust method for exchanging information with other applications and systems.
1. Microservices – An Overview
Applications and IT infrastructure management are now being built and managed on the cloud. Today’s cloud apps require to be responsive, modular, highly scalable, and trustworthy.
Containers facilitate the fulfilment of these needs by applications. To put it another way, attempting to navigate a new location by placing an application in a container without first deciding on a design pattern is like going directionless. You could get where you’re going, but it probably won’t be the fastest way.
.NET Microservices is necessary for this purpose. With the help of a reliable .NET development company offering microservices, the software can be built and deployed in a way that meets the speed, scalability, and dependability needs of today’s cloud-based applications.
2. Key Considerations for Developing .Net Microservices
Before deciding to adopt the microservices pattern for your team, please make sure you have someone in your team who deeply understands and has experience building microservices at scale because it definitely does get more complex with time.
— CTO (@0xbyugo) August 22, 2023
When using .NET to create microservices, it’s important to remember the following points:
API Design
Since microservices depend on APIs for inter-service communication, it’s crucial to construct APIs with attention. RESTful APIs are becoming the accepted norm for developing APIs and should be taken into consideration. To prevent breaking old clients, you should plan for versioning and make sure your APIs are backward compatible.
Data Management
Because most microservices use their own databases, ensuring data consistency and maintenance can be difficult. If you’re having trouble keeping track of data across your microservices, you might want to look into utilising Entity Framework Core, a popular object-relational mapper (ORM) for .NET.
Microservices need to be tested extensively to assure their dependability and sturdiness. For unit testing, you can use xUnit or Moq, and for API testing, you can use Postman.
Monitoring and analysis are crucial for understanding the health of your microservices and fixing any problems that may develop. You might use monitoring and logging tools such as Azure Application Insights.
If you want to automate the deployment of your microservices, you should use continuous integration and continuous delivery (CI/CD) pipeline. This will assist guarantee the steady delivery and deployment of your microservices.
3. Implementation of .Net Microservices Using Docker Containers
3.1 Install .NET SDK
Let’s begin from scratch. First, install .NET 7 SDK.
Once you complete the download, install the package and then open a new command prompt and run the following command to check .NET (SDK) information:
> dotnet |
> dotnet
If the installation succeeded, you should see an output like the following in command prompt:
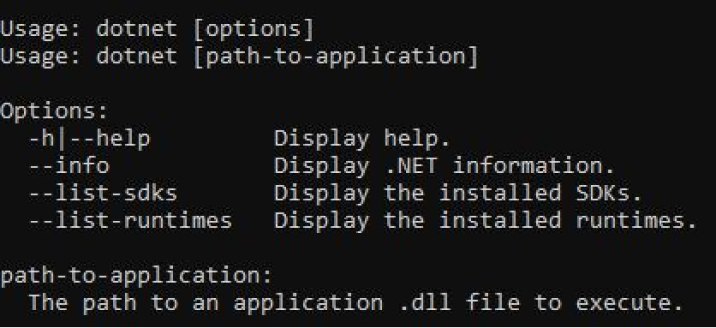
3.2 Build Your Microservice
Open command prompt on the location where you want to create a new application.
Type the following command to create a new app named “MyMicroservices”
> dotnet new webapi -o DemoMicroservice --no-https -f net7.0 |
> dotnet new webapi -o DemoMicroservice --no-https -f net7.0
Then, navigate to this new directory.
> cd DemoMicroservice |
> cd DemoMicroservice
What do these commands mean?
Command | Meaning |
dotnet | It creates a new application of type webapi (that’s a REST API endpoint). |
-o | Creates a directory where your app “DemoMicroservices” is stored. |
–no-https | Creates an app that runs without an HTTPS certificate. |
-f | Indicates that you are creating a .NET 7 application. |
3.3 Run Microservice
Type this into your command prompt:
> dotnet run |
> dotnet run
The output will look like this:
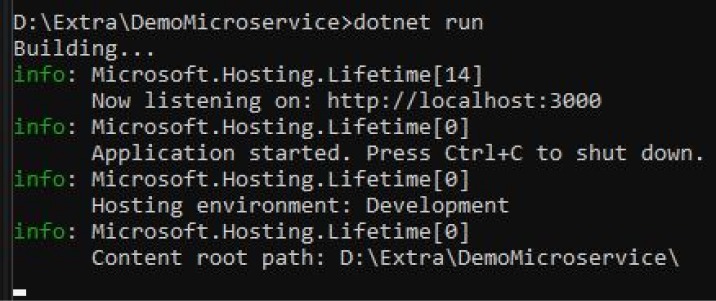
The Demo Code:
Several files were generated in the DemoMicroservices directory. It gives you a simple service which is ready to run.
The following screenshot shows the content of the WeatherForecastController.cs file. It is located in the Controller directory.
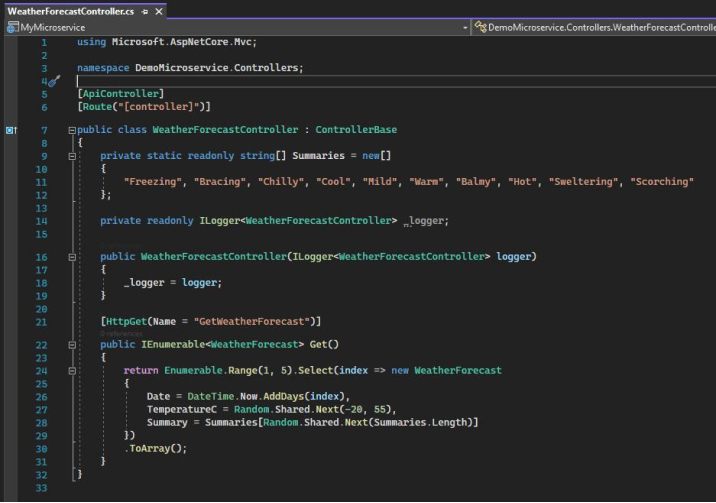
Launch a browser and enter http://localhost:<port number>/WeatherForecast once the program shows that it is monitoring that address.
In this example, It shows that it is listening on port 5056. The following image shows the output on the following url: http://localhost:5056/WeatherForecast.
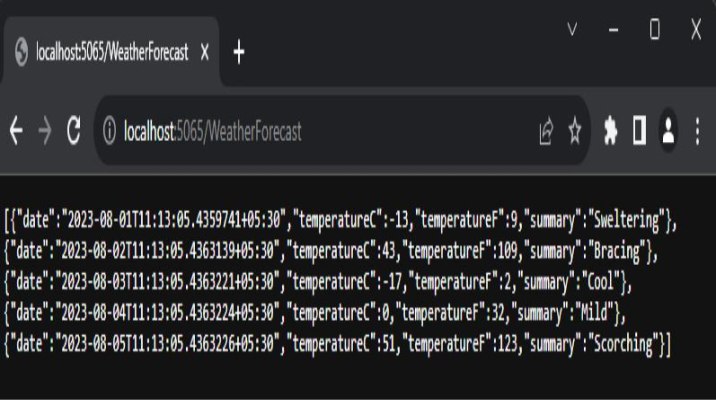
You’ve successfully launched a basic service.
To stop the service from running locally using the dotnet run command, type CTRL+C at the command prompt.
3.4 Role of Containers
In software development, containerization is an approach in which a service or application, its dependencies, and configurations (in deployment manifest files) are packaged together as a container image.
The containerized application may be tested as a whole and then deployed to the host OS in the form of a container image instance.
Software containers are like cardboard boxes in which they are a standardised unit of software deployment that can hold a wide variety of programs and dependencies, and they can be moved from location to location.
This method of software containerization allows developers and IT professionals to easily deploy applications to many environments with few code changes.
If this seems like a scenario where containerizing an application may be useful, it’s because it is. The advantages of containers are nearly identical to the advantages of microservices.
The deployment of microservices is not limited to the containerization of applications. Microservices may be deployed via a variety of mechanisms, such as Azure App Service, virtual machines, or anything else.
Containerization’s flexibility is an additional perk. Creating additional containers for temporary jobs allows you to swiftly scale up. The act of instantiating an image (by making a container) is, from the perspective of the application, quite similar to the method of implementing a service or a web application.
In a nutshell, containers improve the whole application lifecycle by providing separation, mobility, responsiveness, versatility, and control.
All of the microservices you create in this course will be deployed to a container for execution; more specifically, a Docker container.
3.5 Docker Installation
3.5.1. What is Docker?
Docker is a free and set of platform as a service products that use OS level virtualization for automating the deployment of applications as portable, self-sufficient containers that can run on cloud or on-premises. Docker also has premium tiers for premium features.
Azure supports running Docker containers in a variety of environments, including the customer’s own datacenter, an external service provider’s infrastructure, and the cloud itself. Docker images may be executed in a container format on both Linux and Windows.
3.5.2. Installation Steps
Docker is a platform for building containers, which are groups of an app, its dependencies, and configurations. Follow the steps mentioned below to install the docker:
- First download the .exe file from docker website.
- Docker’s default configuration for Windows employs Linux Containers. When asked by the installation, just accept the default settings.
- You may be prompted to sign out of the system after installing Docker.
- Make sure Docker is up and running.
- Verify that Docker is at least version 20.10 if you currently have it installed.
Once the setup is complete, launch a new command prompt and enter:
> docker --version |
> docker --version
If the command executes and some version data is displayed, then Docker has been set up properly.
3.6 Add Docker Metadata
A Docker image can only be created by following the directions provided in a text file called a Dockerfile. If you want to deploy your program in the form of a Docker container, you’ll need a Docker image.
Get back to the app directory
Since the preceding step included opening a new command prompt, you will now need to navigate back to the directory in which you first established your service.
> cd DemoMicroservice |
> cd DemoMicroservice
Add a DockerFile
Create a file named “Dockerfile” with this command:
> fsutil file createnew Dockerfile 0 |
> fsutil file createnew Dockerfile 0
To open the docker file, execute the following command.
> start Dockerfile. |
> start Dockerfile.
In the text editor, update the following with the Dockerfile’s current content:
FROM mcr.microsoft.com/dotnet/sdk:7.0 AS build WORKDIR /src COPY DemoMicroservice.csproj . RUN dotnet restore COPY . . RUN dotnet publish -c release -o /app FROM mcr.microsoft.com/dotnet/aspnet:7.0 WORKDIR /app COPY --from=build /app . ENTRYPOINT ["dotnet", "DemoMicroservice.dll"] |
FROM mcr.microsoft.com/dotnet/sdk:7.0 AS build WORKDIR /src COPY DemoMicroservice.csproj . RUN dotnet restore COPY . . RUN dotnet publish -c release -o /app FROM mcr.microsoft.com/dotnet/aspnet:7.0 WORKDIR /app COPY --from=build /app . ENTRYPOINT ["dotnet", "DemoMicroservice.dll"]
Note: Keep in mind that the file needs to be named as Dockerfile and not Dockerfile.txt or anything else.
Optional: Add a .dockerignore file
If you have a .dockerignore file, it will limit the number of files that are read during the ‘docker build’ process. Reduce the number of files to compile faster.
If you’re acquainted with .gitignore files, the following command will create a .dockerignore file for you:
> fsutil file createnew .dockerignore 0 |
> fsutil file createnew .dockerignore 0
You can then open it in your favorite text editor manually or with this command:
> start .dockerignore |
> start .dockerignore
Then, either manually or with the following command, load it in your preferred text editor:
Dockerfile [b|B]in [O|o]bj |
Dockerfile [b|B]in [O|o]bj
3.7 Create Docker Image
Start the process with this command:
> > docker build -t demomicroservice |
> > docker build -t demomicroservice
Docker images may be created with the use of the Dockerfile and the docker build command.
The following command will display a catalogue of all images on your system, especially the one you just made.
> docker images |
> docker images
3.8 Run Docker image
Here’s the command you use to launch your program within a container:
> docker run -it --rm -p 3000:80 --name demomicroservicecontainer demomicroservice |
> docker run -it --rm -p 3000:80 --name demomicroservicecontainer demomicroservice
To connect to a containerized application, go to the following address: http://localhost:3000/WeatherForecast
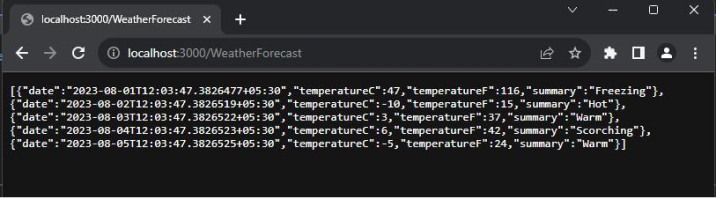
Optionally, The subsequent command allows you to observe your container in a different command prompt:
> docker ps |
> docker ps

To cancel the docker run command that is managing the containerized service, enter CTRL+C at the prompt.
Well done! A tiny, self-contained service that can be easily deployed and scaled with Docker containers has been developed by you.
These elements provide the foundation of a microservice.
4. Conclusion
The .NET Framework, from its inception with .NET Core to the present day, was designed from the ground up to run natively on the cloud. Its cross-platform compatibility means your .NET code will execute regardless of the operating system as your Docker image is built on. .NET is incredibly quick, with the ASP.NET Kestrel web server consistently surpassing its competitors. Its remarkable presence leaves no doubt for disappointment and should be incorporated in your projects.
5. FAQs
Why is .NET core good for microservices?
.NET enables the developers to break down the monolithic application into smaller parts and deploy services separately which can not only help businesses get more time to market the product but also benefit in adapting to the changes quickly and with flexibility. Because of this reason, the .NET core is considered a powerful platform to create and deploy microservices. Besides this, some other major reasons behind it being a good option for microservices are –
- Easier maintenance as with .NET core, microservices can be tested, updated, and deployed independently.
- Better scalability is offered by the .NET core. It scales each service independently to meet the traffic demands.
What is the main role of Docker in microservices?
When it comes to a microservices architecture, the .Net app developers can create applications that are independent of the host environment. This can be done by encapsulating each of the microservices in Docker containers. Docker is a concept that enables the developers to package the applications they create into containers and here each container has a standard executable component and operating system library to run the microservices in any platform.
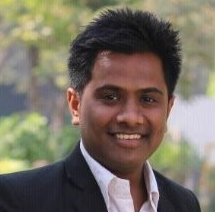
Vishal Shah
Vishal Shah has an extensive understanding of multiple application development frameworks and holds an upper hand with newer trends in order to strive and thrive in the dynamic market. He has nurtured his managerial growth in both technical and business aspects and gives his expertise through his blog posts.
Subscribe to our Newsletter
Signup for our newsletter and join 2700+ global business executives and technology experts to receive handpicked industry insights and latest news
Build your Team
Want to Hire Skilled Developers?
Comments
Leave a message...