Related Blogs
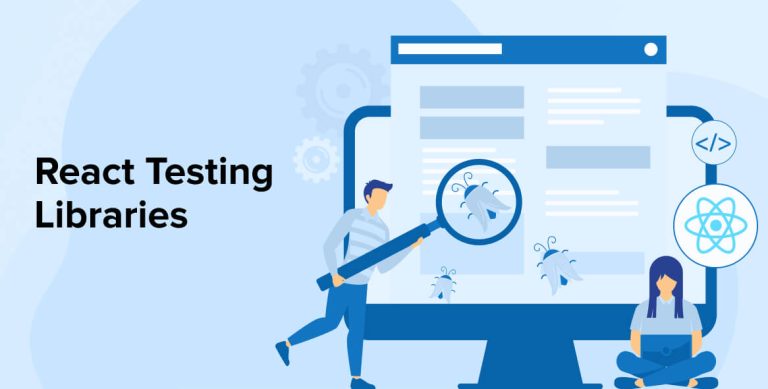
Key Takeaways
- As per Statista, React is the 2nd most used web framework in the world. There are various tools available for testing react applications. So, best tools and practices must be followed by developers.
- Jest, and React Testing Library are the most popular tools recommended by React Community to test react applications.
- Other than that, tools like Chai, Mocha, Cypress.io, Jasmine, and Enzyme are widely used for testing react apps.
- While selecting testing tools, consider iteration speed, environment required, dependencies, and flow length.
Software or application testing is one of the most important parts of software development life cycle. It helps to find out and eliminate potentially destructive bugs and ensure the quality of the final product. When it comes to testing apps, there are various React testing libraries and tools preferred by Reactjs development companies. One can test React components similar to any JavaScript code.
Now, let’s dive deeper on how you can select best-fit libraries or tools for your project.
Points to Consider before Selecting React Testing libraries and Tools:
- Iteration Speed
Many tools offer a quick feedback loop between changes done and displaying the results. But these tools may not model the browser precisely. So, tools like Jest are recommended for good iteration speed. - Requirement of Realistic Environment
Some tools use a realistic browser environment but they reduce the iteration speed. React testing Libraries like mocha work well in a realistic environment. - Component Dependencies
Some react components have dependencies for the modules that may not work best in the testing environment. So, carefully mocking these modules out with proper replacements are required. Tools and libraries like Jest support mocking modules. - Flow Length
To test a long flow, frameworks and libraries like Cypress, Playwright, and Puppeteer are used to navigate between multiple assets and routes.
Now, let’s explore a few of the best options to test React components.
1. Best React Testing Libraries and Tools
Here is a list of a few highly recommended testing libraries for React application.
1.1 Jest
Jest, a testing framework developed and supported by Facebook, is the industry standard. It has been embraced by companies like Uber and Airbnb for usage in testing React components.
While looking for a React Testing Framework, the React Community highly suggests Jest. The unit test framework is self-contained, complete with an automated test runner and assertion features.
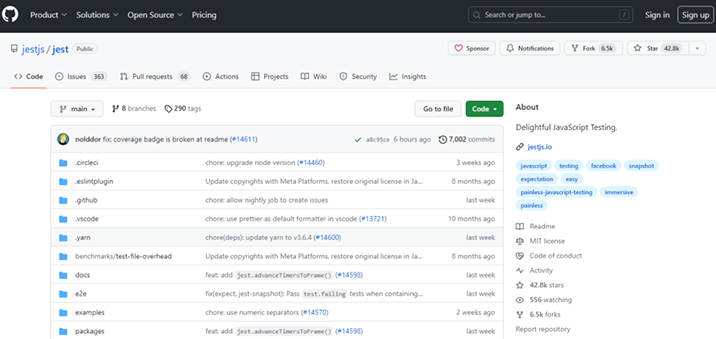
Github Stars – 42.8K
Github Forks- 6.5K
1.1.1 Features of Jest
- When it comes to JavaScript projects, Jest is meant to run without any further configuration.
- Individual test procedures are started in parallel to increase throughput.
- Quick and risk-free.
- Entire toolkit of Jest is presented at one place. It is well documented and well maintained.
- Including untested files, Jest collects code coverage information from entire projects.
1.1.2 How to Install Jest?
Download and install Jest using your preferred package manager:
npm install --save-dev jest or yarn add --dev jest |
npm install --save-dev jest or yarn add --dev jest
1.1.3 First Test Using Jest:
Now, let’s create a test for a made-up function that supposedly performs a simple arithmetic operation on two values. First, you should make a file called “add.js”
function add(x, y) { return x+y; } module.exports = add; |
function add(x, y) { return x+y; } module.exports = add;
Start by making a new file and naming it add.test.js. Here is where we’ll find the real test:
const add = require('./add'); test('adds 2 + 2 to equal 4', () => { expect(add(1, 2)).toBe(4); }); |
const add = require('./add'); test('adds 2 + 2 to equal 4', () => { expect(add(1, 2)).toBe(4); });
The preceding must be included in the package. json:
function add(x, y) { return x+y; } module.exports = add; |
function add(x, y) { return x+y; } module.exports = add;
This text will be printed by Jest when you run yarn test or npm test:
PASS ./add.test.js
✓ adds 2 + 2 to equal 4 (5ms)
The First Jest Test case is completed. This check ensured that two values were similar by comparing them using expect and toBe, respectively.
1.2 React Testing Library
A sizable developer community stands behind the React-testing-library development. You may simply test components by imitating real-world user actions without relying on the implementation details.
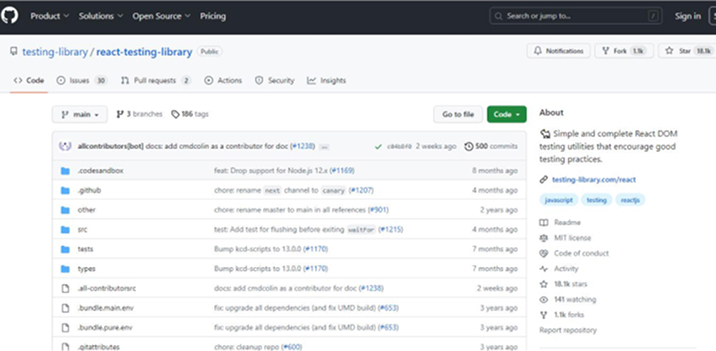
Github Stars – 18.1K
Github Forks- 1.1K
To test the React DOM, this package provides a set of tools that work together like an enzyme, mimicking real-world user interactions. You may put your React components through their paces with the help of the React testing package.
1.2.1 Features of React Testing Library
- Very light-weight
- It has inbuilt DOM testing utilities
- More accessible library
1.2.2 How to Install React Testing Library?
Node Package Manager (npm) is already setup with node, thus there’s no need to install anything else to use this component in your project.
npm install --save-dev @testing-library/react |
npm install --save-dev @testing-library/react
For yarn users, follow:
yarn add --dev @testing-library/react |
yarn add --dev @testing-library/react
The react and react-dom peerDependencies lists can be found here. To use the React Testing Library v13+, React v18 is required. Upgrade to React 12 if you’re still using an earlier edition in your project.
npm install --save-dev @testing-library/react@12 yarn add --dev @testing-library/react@12 |
npm install --save-dev @testing-library/react@12 yarn add --dev @testing-library/react@12
First Test Case:
Create firstApp.js in src directory.
firstApp.js
Write the following code to print the Hello World!
import React from "react"; export const App = () => <h1>Hello World!</h1>; |
import React from "react"; export const App = () => <h1>Hello World!</h1>;
Now, add the firstApp.test.js file in the src directory.
import React from "react"; import { render } from "@testing-library/react"; import { firstApp } from "./firstApp"; describe("App Component", function () { it("should have Hello World! string", function () { let { getByText } = render(<firstApp />); expect(getByText("Hello world!")).toMatchInlineSnapshot(` <h1> Hello World! </h1> `); }); }); |
import React from "react"; import { render } from "@testing-library/react"; import { firstApp } from "./firstApp"; describe("App Component", function () { it("should have Hello World! string", function () { let { getByText } = render(<firstApp />); expect(getByText("Hello world!")).toMatchInlineSnapshot(` <h1> Hello World! </h1> `); }); });
1.3 Chai
One of the most well-known node and browser-based BDD / TDD assertion and expectation React testing libraries is called Chai. It works well with any existing JavaScript testing framework like Mocha, Jest and Enzyme as well.
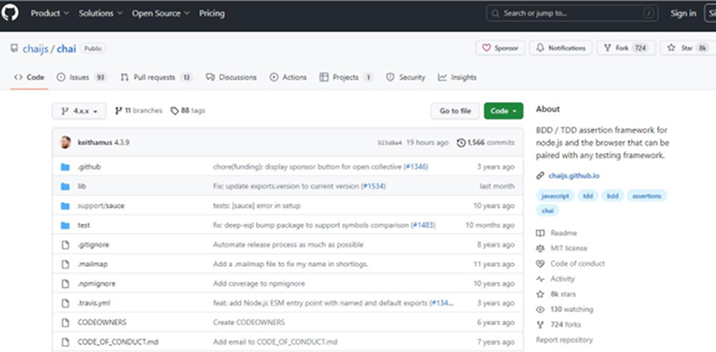
Github Stars – 8K
Github Forks- 724
To specify what should be expected in a test, you may use functionalities like expect, should, and assert. Assertions about functions are another possible use.
1.3.1 Features of Chai
- Chai allows software testers to perform various types of assertions.
- Expect
- Assert
- Should
- The Chai expect and Should styles are used to chain the natural language assertions together. The only difference is chai should style extends “should” with each property.
- The Chai assert style offers the developer with a standard assert-dot notation comparable to what is included with node.js.
- The assert style component additionally offers further tests and browser support.
1.3.2 How to Install Chai?
Chai can be downloaded through the NPM. Just enter this into your command prompt to begin the installation process.
$ npm install --save-dev chai |
$ npm install --save-dev chai
The chai.js file included in the download may also be used in the browser after it has been installed using the npm package manager. For instance:
<script src="./node_modules/chai/chai.js"></script> |
<script src="./node_modules/chai/chai.js"></script>
1.3.3 First Test Using Chai:
To begin using the library, just import it into your program and select the desired approach (either assert, expect, or should).
var chai = require('chai'); var assert = chai.assert; // Using Assert style var expect = chai.expect; // Using Expect style var should = chai.should(); // Using Should style |
var chai = require('chai'); var assert = chai.assert; // Using Assert style var expect = chai.expect; // Using Expect style var should = chai.should(); // Using Should style
app.js
function app(int a, int b){ return a*b; } |
function app(int a, int b){ return a*b; }
test.js
describe(“Multiplication”, () => { it(“Multiplies 2 and 3”, () => { chai.expect(app(2,3).to.equal(6); }); it(“Multiplies 4 and 2”, () => { chai.expect(app(4,2).to.equal(7); }); }); |
describe(“Multiplication”, () => { it(“Multiplies 2 and 3”, () => { chai.expect(app(2,3).to.equal(6); }); it(“Multiplies 4 and 2”, () => { chai.expect(app(4,2).to.equal(7); }); });
1.4 Mocha
Mocha is a popular framework to test react applications. It allows you to use any assertion library, runs tests asynchronously, generates coverage reports, and works in any web browser.
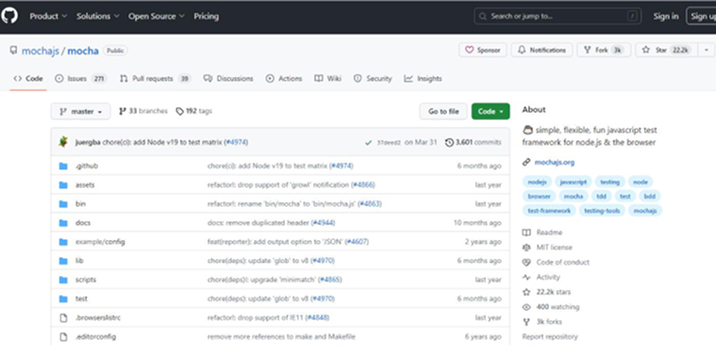
Github Stars – 22.2K
Github Forks- 3K
While putting required codes together for a test, developers use the appropriate React development tools, methodologies and approaches. Mocha is suitable with a broad variety of testing functions and packages. Mocha is a best substitute for Jest because of its simplicity in comparison with Jest in areas like mocking.
1.4.1 Features of Mocha
- Capabilities to test synchronous and asynchronous programs using a simple interface.
- Versatile and precise analysis
- The capacity to execute tests in a linear manner while monitoring for and reacting to undetected exceptions by tracing them to test cases.
- Ability to execute functions in a predetermined order and record the results to a terminal window.
- Software state is automatically cleaned up so that test cases can run separately from one another.
1.4.2 How to Install Mocha?
Installing Mocha as a development dependency on your React project is the first step toward utilizing Mocha to test your React apps.
npm i --save-dev mocha |
npm i --save-dev mocha
The following command should be used if Yarn is being used as the package manager:
yarn add mocha |
yarn add mocha
To include Mocha to your package.json, upgrade the test script first.
{ "scripts": { "test": "mocha" } } |
{ "scripts": { "test": "mocha" } }
1.4.3 First Test Using Mocha:
// test/test.js var modal = require('modal'); describe('Array', function() { describe('#indexOf()', function() { it('should return 0 when the value is not present', function() { assert.equal([5, 4, 3, 2, 1].indexOf(6), -3); }); }); }); |
// test/test.js var modal = require('modal'); describe('Array', function() { describe('#indexOf()', function() { it('should return 0 when the value is not present', function() { assert.equal([5, 4, 3, 2, 1].indexOf(6), -3); }); }); });
The aforementioned test looked for the index “6” in the column and reported “-3” if it was not found.
1.5 Cypress.io
Cypress is a lightning-fast end-to-end test automation framework by which writing tests can become easier without the need for any extra testing library or tool. It enables testing in production environments, such as actual browsers and command prompts.
The code may be tested in the actual browser, and browser development tools can be used in tandem with it. The test results for everything may be monitored and managed from the centralized dashboard.
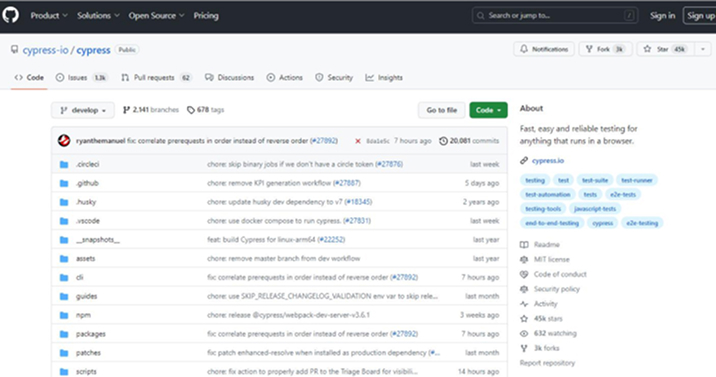
Github Stars – 45K
Github Forks- 3K
1.5.1 Features of Cypress
- Cypress records snapshots over the course of your test runs.
- Rapid debugging is possible because of the clearly shown errors and stack traces.
- Cypress will patiently wait for your instructions and statements.
- Testing that is quick, consistent, and dependable without any hiccups is desirable.
1.5.2 How to Install Cypress?
On the command line, type the preceding to initiate a React project:
npm create vite@latest my-awesome-app -- --template react |
npm create vite@latest my-awesome-app -- --template react
Open the folder and type npm install:
cd my-awesome-app npm install |
cd my-awesome-app npm install
Adding Cypress to the program is the next step.
npm install cypress -D |
npm install cypress -D
Open Cypress:
npx cypress open |
npx cypress open
Use Cypress’s Launchpad to get help setting up your work.
1.5.3 First Test Using Cypress:
Returning to the Cypress testing app’s “Create your first spec” page, select “Create from component” to get started.
A prompt will appear with a list of all the element files in the app Cypress will filter out *.config.{js,ts} and *.{cy,spec}.{js,ts,jsx,tsx} from this list. Find the Stepper component by expanding the row for Stepper.jsx.
src/component/Stepper.cy.jsx is where the following spec file was written:
src/components/Stepper.cy.jsx import React from 'react' import Stepper from './Stepper' describe('<Stepper />', () => { it('renders', () => { // Check : https://on.cypress.io/mounting-react cy.mount(<Stepper />) }) }) |
src/components/Stepper.cy.jsx import React from 'react' import Stepper from './Stepper' describe('<Stepper />', () => { it('renders', () => { // Check : https://on.cypress.io/mounting-react cy.mount(<Stepper />) }) })
The Stepper module is initially brought in. Next, we utilize describe and it to create sections for our tests within method blocks, this allows us to manage the test suite more accurately. Cypress provides these features on a global level, so you won’t need to manually import anything to utilize them.
The top-level describe block will hold all of the tests in a single file, and each one will be a separate test. The first parameter to the describe feature is the name of the test suite. The second parameter is a feature that will run the tests.
Here’s the demo video from Cypress – Test Replay Product Demo
1.6 Jasmine
Jasmine is an excellent open-source BDD framework and test runner for evaluating a wide variety of JavaScript software. The user interface is put through its paces across a variety of devices and screen sizes, from smartphones to TVs. To ensure their code is bug-free, many Angular CLI programmers also consider Jasmine to be an indispensable tool. Developers typically employ it alongside Babel and Enzyme when testing React applications. The handy util package provides more information about testing your React code.
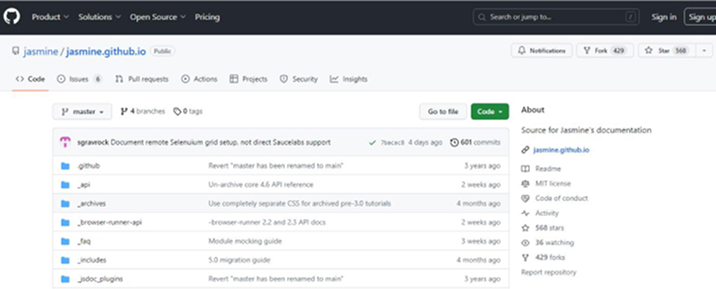
Github Stars – 568
Github Forks- 429
1.6.1 Features of Jasmine
- It’s quick, has little overhead, and doesn’t rely on anything except itself.
- It provides almost every functionalities and features that one requires to test the code.
- You may use it with the browser or Node.
- It’s compatible with Python and Ruby also.
- In other words, the DOM is not necessary.
- It has a simple syntax and an extensive API that is easy to use.
- The tests and their outcomes can be described in ordinary terms.
1.6.2 How to Install Jasmine?
Installing Jasmine as part of the setup is recommended because developers frequently use it in conjunction with Enzyme.
npm install - - save - dev @babel/core \ @babel/register \ babel-preset-react-app \ cross-env \ jsdom \ jasmine OR yarn add - - dev babel-cli \ @babel/register \ babel-preset-react-app \ cross-env \ enzyme \ enzyme-adapter-react-16 \ jasmine-enzyme \ jsdom \ jasmine |
npm install - - save - dev @babel/core \ @babel/register \ babel-preset-react-app \ cross-env \ jsdom \ jasmine OR yarn add - - dev babel-cli \ @babel/register \ babel-preset-react-app \ cross-env \ enzyme \ enzyme-adapter-react-16 \ jasmine-enzyme \ jsdom \ jasmine
Follow this command to start Jasmine:
// For NPM npm jasmine init // For Yarn yarn run jasmine init |
// For NPM npm jasmine init // For Yarn yarn run jasmine init
1.6.3 First Test Using Jasmine
Jasmine expects all configuration files, such as those for Babel, Enzyme, and JSDOM, to be placed in a spec folder.
// babel.js require('@babel/register'); // for typescript require('@babel/register')({ "extensions": [".ts", ".tsx", ".js", ".jsx"] }); describe("A suite is a function", function() { // For grouping related specs, there is a describe function. // Typically each test file has one describe function at the top level. let x; it("and so is a spec", function() { // Specs are defined by calling the global Jasmine function it. x = true; expect(x).toBe(true); }); }); describe("The 'toBe' compares with ===", function() { it("and has a negative case", function() { expect(false).not.toBe(true); }); it("and has a positive case", function() { expect(true).toBe(true); }); }); |
// babel.js require('@babel/register'); // for typescript require('@babel/register')({ "extensions": [".ts", ".tsx", ".js", ".jsx"] }); describe("A suite is a function", function() { // For grouping related specs, there is a describe function. // Typically each test file has one describe function at the top level. let x; it("and so is a spec", function() { // Specs are defined by calling the global Jasmine function it. x = true; expect(x).toBe(true); }); }); describe("The 'toBe' compares with ===", function() { it("and has a negative case", function() { expect(false).not.toBe(true); }); it("and has a positive case", function() { expect(true).toBe(true); }); });
1.7 Enzyme
When it comes to testing React components, developers may rely on Enzyme, a testing tool created to make the process easier. One of Airbnb’s most popular React testing libraries is called Enzyme. To fully test the React application, developers often pair it with another framework like Jest, Chai, or Mocha.
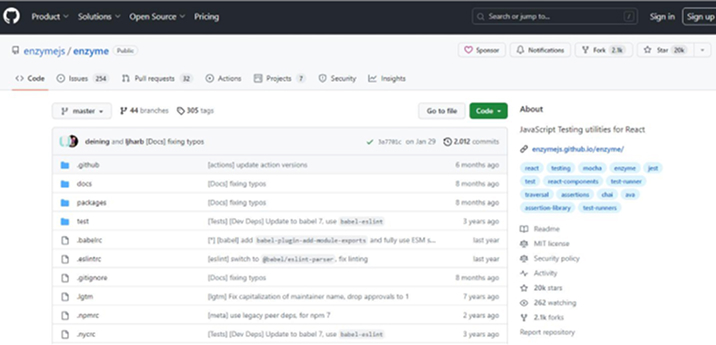
Github Stars – 20K
Github Forks- 2.1K
The sole purpose of the enzyme is to render components, retrieve resources, identify elements, link components, and simulate events. It may make use of assertions written in either Chai or Jest. Testing is simplified by abstracting the rendering of components in React so that you may test their results.
1.7.1 Features of Enzyme
- Enzyme’s API aims to be user-friendly and adaptable by modeling itself after jQuery’s API for DOM manipulation and traversal.
- The Enzyme Application Programming Interface allows for the Inspection of React Elements.
- It provides shallow rendering.
- Provides access to enterprise implementations of your component.
- Provides execution of a complete DOM rendering.
- There are cases where using react-hooks in shallow rendering is appropriate.
1.7.2 How to Install Enzyme?
For installation using npm:
npm - - save - dev enzyme enzyme-adapter-react-16 |
npm - - save - dev enzyme enzyme-adapter-react-16
7.3 First Test Using Enzyme
To install Enzyme, write the following command in npm.
npm install –save-dev enzyme |
npm install –save-dev enzyme
Now, create an app.tsx file in the src folder with the following code.
import React, { Component } from 'react'; import './App.scss'; class App extends React.Component<any, any> { constructor(props: any) { super(props); this.state = { }; } render() { return ( <div> <button id="ClickHere" className="click-here">Click Here</button> </div> ) } }; export default App; |
import React, { Component } from 'react'; import './App.scss'; class App extends React.Component<any, any> { constructor(props: any) { super(props); this.state = { }; } render() { return ( <div> <button id="ClickHere" className="click-here">Click Here</button> </div> ) } }; export default App;
Now, create an app.test.tsx file in the same folder and write the following code.
import React from 'react' import Enzyme, { shallow } from 'enzyme' import Adapter from 'enzyme-adapter-react-16' import App from './App' Enzyme.configure({ adapter: new Adapter() }) describe('First Test Case', () => { it('it should render button', () => { const wrapper = shallow(<App />) const buttonElement = wrapper.find('#ClickHere'); expect(buttonElement).toHaveLength(1); expect(buttonElement.text()).toEqual('Click Here'); }) }) |
import React from 'react' import Enzyme, { shallow } from 'enzyme' import Adapter from 'enzyme-adapter-react-16' import App from './App' Enzyme.configure({ adapter: new Adapter() }) describe('First Test Case', () => { it('it should render button', () => { const wrapper = shallow(<App />) const buttonElement = wrapper.find('#ClickHere'); expect(buttonElement).toHaveLength(1); expect(buttonElement.text()).toEqual('Click Here'); }) })
Then use the “npm test” command to test the code.
2. Conclusion
Because of React’s modular design, TDD (Test Driven Development) is improved. Finding the right technology may facilitate the implementation of this idea and help you to harvest its benefits, from testing specific parts to testing the complete system.
Combining the proper testing framework (such as Jest, chai, enzyme, etc.) with the necessary assertion/manipulation libraries is the secret to establishing a flexible approach. Using virtual isolation, you can take scalability and TDD to an entirely higher platform by separating components from their tasks (like Bit etc).
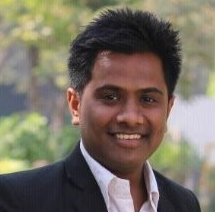
Vishal Shah
Vishal Shah has an extensive understanding of multiple application development frameworks and holds an upper hand with newer trends in order to strive and thrive in the dynamic market. He has nurtured his managerial growth in both technical and business aspects and gives his expertise through his blog posts.
Subscribe to our Newsletter
Signup for our newsletter and join 2700+ global business executives and technology experts to receive handpicked industry insights and latest news
Build your Team
Want to Hire Skilled Developers?
Comments
Leave a message...