Related Blogs
Table of Content
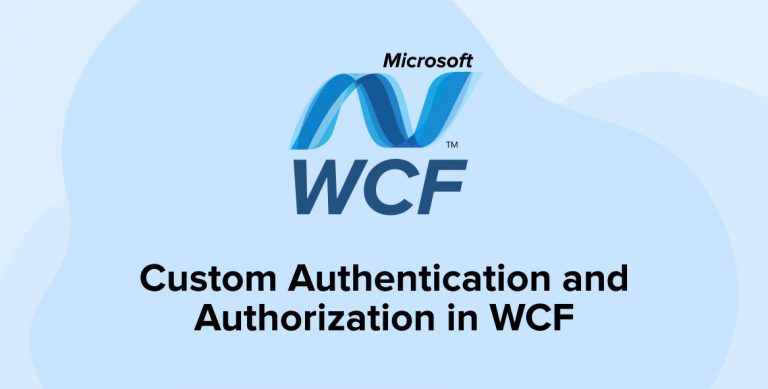
Custom Authentication in WCF
Windows Communication Foundation (WCF) is a .Net framework to build and develop service applications and also enhances to support multiple different protocols than its traditional “web service” counterpart like https, IPC, MSMQ, TCP etc.
WCF provides various security modes to create a secure communication channel when communicating with the client server.
Transfer Security Level
- Message security mode: This security mode provides end to end security which is transported through insecure communication like HTTP.
- Transport security mode: This security mode provides point to point security by transferring messages over communication protocols like TCP, IPC, Https.
- Mixed transfer security mode: This mode provides transport security for message privacy and it uses message security for security credentials.
- Both security mode: This mode is more robust than others and uses both transport and message-level security where WCF uses the secure channel with the encrypted message but it overloads the performance.
None: In this mode, the WCF service doesn’t use any authentication.
Message Security Level
- None: No client authentication is performed in this level, the only message is encrypted for security.
- Windows Authentication: Client must provide windows credential to authenticate itself along with encryption of the message.
- Certificate: This mode uses the client certificate to authenticate client along with message encryption.
- UserName: This mode uses UserName and Password to authenticate client along with message encryption.
- Issued Token: This mode uses tokens to authenticate client along with message encryption.
In this article, we’ll understand custom authentication with Message level security with wsHttpBinding and UserName security level and the custom authorization with custom attribute.
Create New WCF Service
To create new WCF service:
Open visual studio → select New Project → select WCF template → select WCF service Application
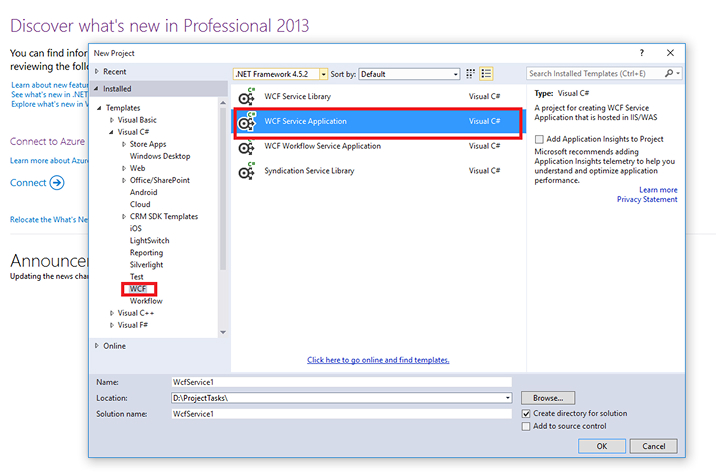
This will create an interface class and svc files automatically.
Interface class would be decorated with [ServiceContact] which describes the operations a service exposes to the client or another party.
The Methods that are decorated with [OperationContract] attribute are exposed to the client otherwise not.
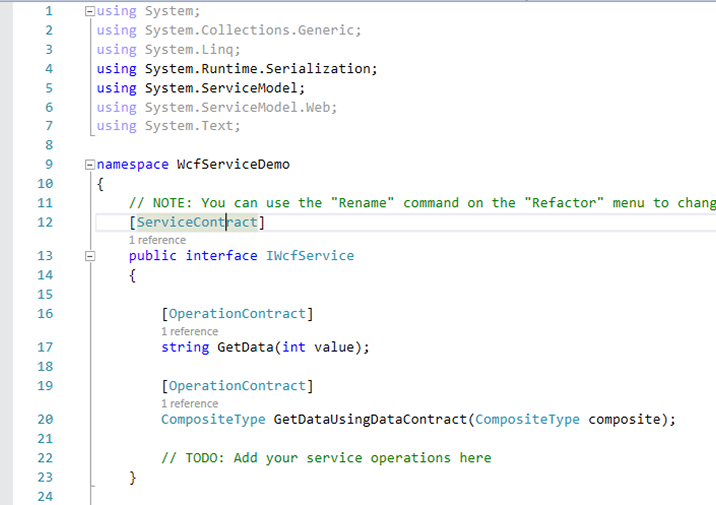
Now configure <services> under <system.servicemodel>

Set endpoint address, binding type and contract under <service> section.
- After completing all the steps try to browse service1.svc through visual studio in the browser. We will be able to access service.
- Now we would implement user authentication in our service by using an abstract class UserNamePasswordValidator.
- To implement abstract class UserNamePasswordValidator. Add reference to System.IdentityModel and create new class with suitable name, inherit UserNamePasswordValidator and override its Validate(string,string) method.
- In the Validate () method, we can write any custom logic according to our requirement to validate the user credentials passed to WCF service by the client.
- Also few changes to be done in web.config apart from overriding validate method in the service.
- Add binding to message mode security and set client credential type to UserName in <system.serviceModel> in web config.
.Net developers can choose any client credential type according to our requirement but for this example, we are using UserName client credential type.
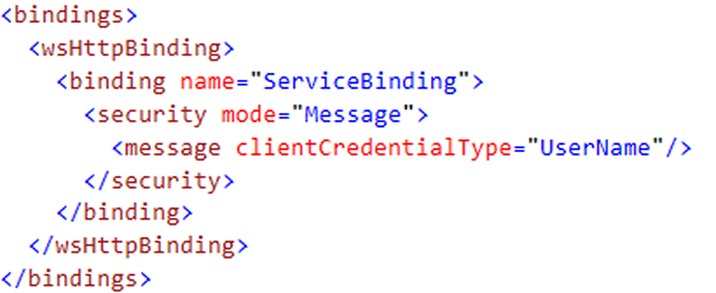
To make authentication of WCF service more secure use server certificate for authentication.
If certificate is available include it in WCF server otherwise we can also create self-signed certificate from IIS.
Follow These Steps to Create Self-Signed Certificate from IIS:-
Step 1 – Open IIS Manager select and double-click “Server Certificates” From “IIS” pane.
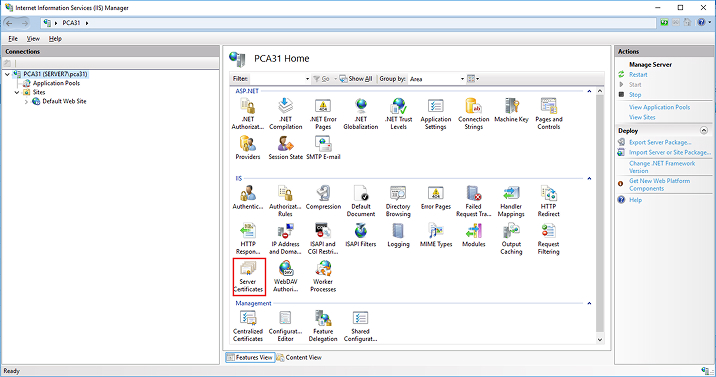
Step 2 – Select “Create Self Signed Certificate”
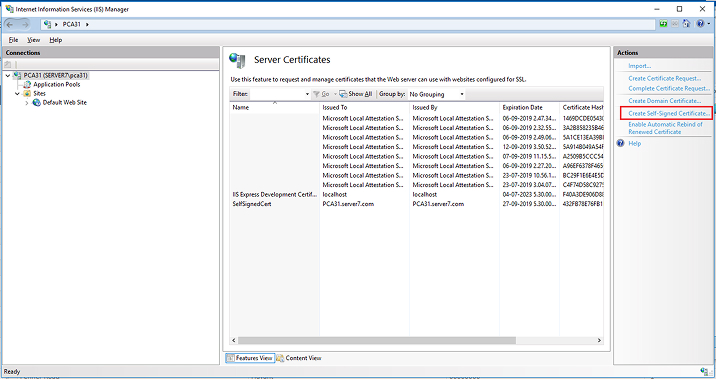
Step 3 – Provide suitable name for certificate and select certificate store for the new certificate then click “Ok” to create certificate.
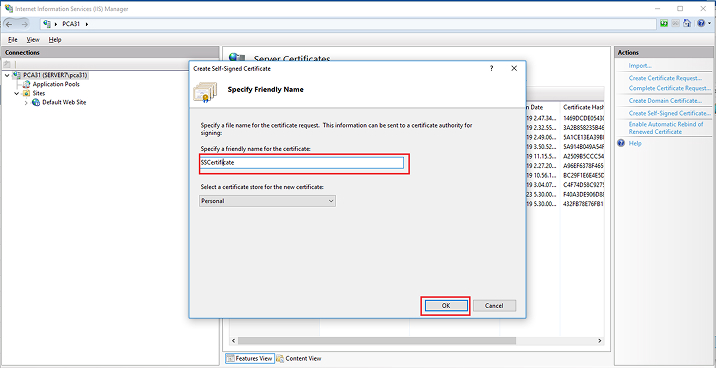
Step 4 – After clicking “OK” New certificate is created as highlighted below.
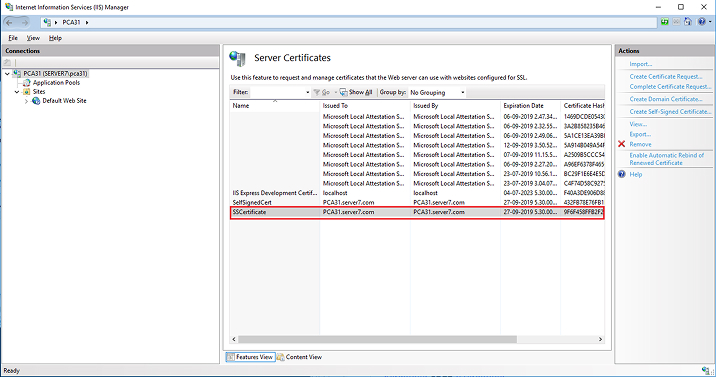
To make WCF service use our custom authentication make some changes in <behavior> section of <serviceBehaviors> in web.config.
Also, add the created self-signed certificate in “serviceCredentials” section.

As shown in above web.config file set attribute “cusomUserNamepasswordValidatorType” to WcfServiceDemo(service name).clientauthentication(class in which we have implemented our custom authentication code).WcfServiceDemo(service name).
Custom Authorization in WCF
Authorization is the process to get access and control to the WCF service by identifying clients that call the service and what operations a client can access.
After authenticating the user we should authorize the user to determine that which operations logged in user can access of WCF service.
WCF supports three ways to Authorize the user:-
- Role-based Access – In this method access to the user for operations on the service is based on the user’s role.
- Identity based Access/Claims based access – In this method access to the user is given based on its claims that are retrieved by user’s credentials when authenticating the user. This approach also used with issue token authentication.
- Resource based Access – In this method, Windows Access Control Lists (ACLs) use the identity of the caller to determine access rights to the resource.
There are multiple ways to determine the user’s role:-
- Windows Groups – In this method, we can use built-in Windows groups such as Administrators or Power Users or we can create your own Windows groups.
- Custom Roles – In this method, we can create roles that are specific to the application, like admin, users.
- ASP.NET Role Management – In this method, ASP.Net developers can use the ASP.NET role provider and user roles that have defined for a Web site.
WCF Pipeline
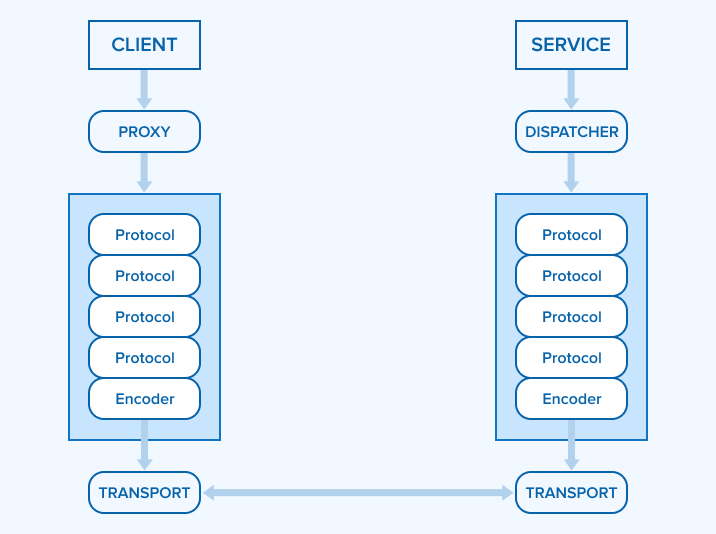
- Injecting a Parameter Inspector in the WCF pipeline at the client or service side will allow validating any of the parameters that are going to be sent to the service or are received at the service end.
- Parameter Inspector intercepts the incoming and outgoing requests and after processing the requests it extracts the parameters and inspects it.
- The parameter inspector is the same for both server side and client side.
- In WCF, Parameter inspector is implemented by implementing the interface ‘IparameterInspector’.
Implementation of Custom Authorization in WCF Service using ParameterInspector
Implement custom “BusinessServiceAccess” class by deriving “System.Attribute” class which contains “CheckAccessRights” method to check the access rights of the authenticated user in the WCF service.
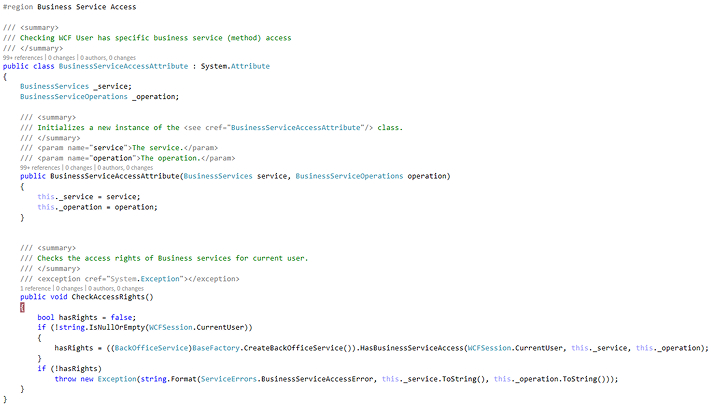
- We will use “CheckAccessRights” method to check the user authorization when it tries to call WCF service by extracting the parameters that are passed by the caller.
- For intercepting the requests made by the caller and extracting the parameters from the request we will create class “MyParameterInspector” which implements “IparameterInspector” interface.
- “MyparameterInspector” class implements two methods “BeforeCall” and “AfterCall”.
- AfterCall method executes after client calls are returned and beforservice responses are sent.
- BeforeCall method executes before client calls are sent and after service responses are returned.
In “BeforeCall” method we have executed “CheckAccessRights” method if any attribute is present in the caller request and also to determine which roles are accessible by the caller by checking attributes.
Now that we have defined our parameter inspector we need to tell our WCF service that we are going to use it. To implement this we have to inject our “MyParameterInspector” class to the server side.
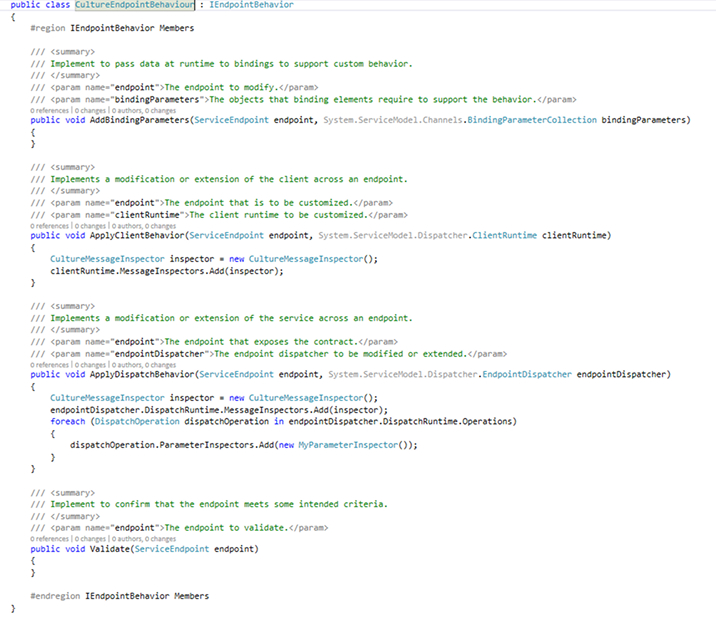
In the above code snippet we have used interface “IEndPointBehavior” which requires to implement the following four methods:-
- AddBindingParameters: This method is used to pass custom data at runtime to enable bindings to support custom behavior.
- ApplyClientBehavior: This method is used modify, examine or insert extensions to a client dispatcher in a client application.
- ApplyDispatchBehavior: This method is used to modify, examine or insert extensions to operation-wide execution in a service application.
- Validate: This method is used to confirm that an OperationDescription meets specific requirements. This can be used to ensure that an operation has a certain configuration setting enabled, supports a particular feature and other requirements.
In the method “ApplyDispatchBehavior” we have injected our parameter inspector in “dispatchOperation.ParameterInspectors”.
To extend the behavior of the class “CultureEndpointBehavior” shown in above code snippet we need to implement new class which is derived from abstract class “BehaviorExtensionElement”.
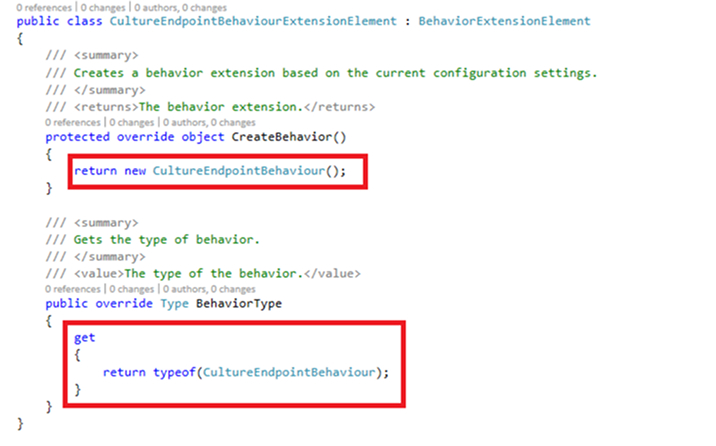
In the above class two methods are overridden of abstract class.
- “CreateBehavior” method – Creates the instance of the extended behavior. As shown in above code snippet we have returned object of “CultureEndPointBehavior” class. The class that we have created above to inject parameterinspector to our WCF Service. Refer to fig (III).
- “BehaviorType” property returns the type of extended behavior. As shown in above code snippet we have returned type of (CultureEndPointBehavior).
- Now to add the extension in WCF service add section “<extension></extension>” in web.config file

Our custom “parameterinspector” is now ready for use and service can authorize the caller using the Attribute.
The only thing that remains is to decorate one or more of the exposed operation on the service contract with the newly created “BusinessServiceAccess” attribute.
Please note here that we are passing two parameters in BusinessServiceAccess attribute and this is the advantage over the traditional Authorize attribute approach where we could pass additional parameters which we could use at the time of checking rights. Refer CheckAccessRights function in BusinessServiceAccessAttribute class.
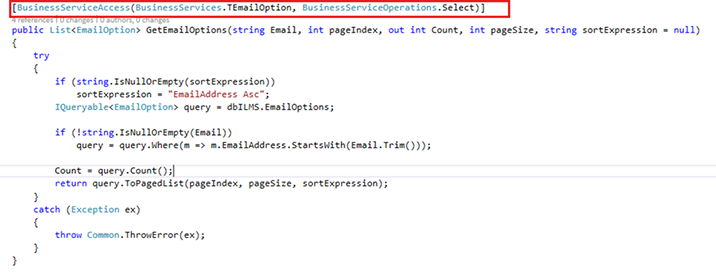
Summary
In this article we understand how to implement custom authentication and authorization in WCF service. In first part we understood about the authentication and also implemented custom authentication in WCF. In later part we have discussed about the authorization in WCF and also implemented custom authorization using Parameter Inspector.
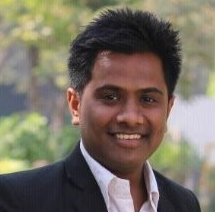
Vishal Shah
Vishal Shah has an extensive understanding of multiple application development frameworks and holds an upper hand with newer trends in order to strive and thrive in the dynamic market. He has nurtured his managerial growth in both technical and business aspects and gives his expertise through his blog posts.
Subscribe to our Newsletter
Signup for our newsletter and join 2700+ global business executives and technology experts to receive handpicked industry insights and latest news
Build your Team
Want to Hire Skilled Developers?
Fantastic article on authentication and authorization in WCF! I was struggling to create a new WCF service, but this article cleared up all of my doubts. The given example and code are really useful for me. Thank you for this article!