Related Blogs
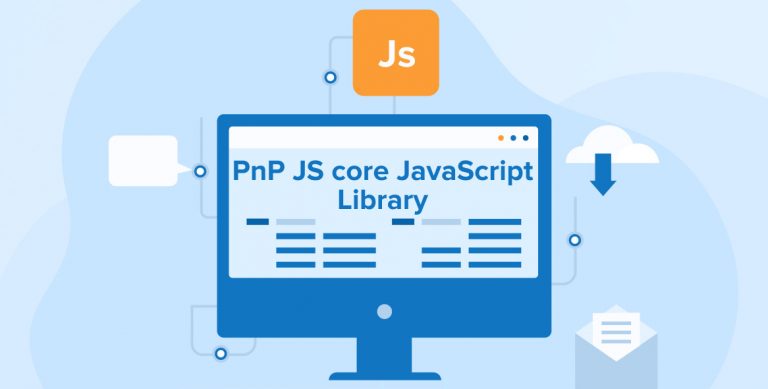
In SharePoint on-premise, to perform various basic custom operations on SharePoint lists or libraries CAML/XML-based feature is used. Later, these operations are provided using the client-side object model (CSOM), REST API, etc. In Microsoft SharePoint Online and Microsoft Office 365, various operations are served through remote provisioning technique, i.e. CSOM. Customization is provisioned in full trust code solutions or sandbox solutions.
Microsoft has introduced PnP JS to ease the above process. PnP JS stands for the “Pattern and Practices JavaScript Core Library.” This core library is fluent JavaScript API and used for the simplified common operations in SharePoint and SharePoint Framework like CRUD operations with the list, copy documents from one library to another, etc. Currently, this API is working with REST API, Utility and helper functions in a type-safe way. You can use this API in NodeJS, SharePoint Framework, and JavaScript project. This is an open source initiative API which is provided and managed by the Microsoft developer. This APIs/Projects are maintaining in the GitHub, where other developers can also share their solutions/scenarios in it.
Prerequisites
PnP JS can be used for various SharePoint operations in site. SharePoint Developers can add it by giving reference of this JS file in SharePoint site page and use it with JavaScript/jQuery code. To develop some functionality using PNP JS, it requires some prerequisites and script API’s to be added, and they are listed below:
- jQuery reference file
- e6-promise script API JS
- Fetch script API JS
- PnP reference file
CDN paths for these files are as below:
- JQuery.js: https://code.jquery.com/jquery-3.3.1.min.js
- e6-Promise.js: https://cdnjs.cloudflare.com/ajax/libs/es6-promise/4.1.1/es6-promise.js
- A lightweight library that provides tools for organizing asynchronous code.
- fetch.js: https://cdnjs.cloudflare.com/ajax/libs/fetch/3.0.0/fetch.js
- This JavaScript is a Promise-based mechanism to request web from browser programmatically.
- PnP.js: https://cdnjs.cloudflare.com/ajax/libs/sp-pnp-js/3.0.10/pnp.min.js
Note: Please add script references of above JS files in the same order as provided above.
Let’s check some basic CRUD operations with PNP JS in a SharePoint site.
Basic CRUD operations with Pattern and Practice JS
You can add this code in the Content Editor Web Part of the SharePoint site page. Please add above mentioned script files’ references in code before adding the PNP JS code chunk in the web part.
Read Operation
- Let’s start with simply Read operation with PnP JS.
Web Details
You can use below PnPJS get() function to get the current web information.
$pnp.sp.web.get().then(r => { $(".output")[0].innerText = r.Title; }); |
$pnp.sp.web.get().then(r => { $(".output")[0].innerText = r.Title; });
Script Code:
Output:
Note: This PnPJS script works like REST API in the backend.
You can also get web object of different web by using web URL with PnP JS. Please check below code for the same.
var web = new $pnp.Web(“”); web.select(“Title”).get().then(r => { $(“.output”)[0].innerText = r.Title; }) |
var web = new $pnp.Web(“”); web.select(“Title”).get().then(r => { $(“.output”)[0].innerText = r.Title; })
Get Lists Details
Below code is used to get the list count of the current web.
$pnp.sp.web.lists.get().then(r => { $(".output")[0].innerText = r.length; }); |
$pnp.sp.web.lists.get().then(r => { $(".output")[0].innerText = r.length; });
Script Code:
OutPut:
Get List Items
You can use the below script to read the list items using list name:
$pnp.sp.web.lists.getByTitle("Test 1").items.get().then(r => { $(".output")[0].innerText = r.length; }); |
$pnp.sp.web.lists.getByTitle("Test 1").items.get().then(r => { $(".output")[0].innerText = r.length; });
Script Code:
OutPut:
You can also get item by ID using below script:
$pnp.sp.web.lists.getByTitle("Test 1").items.getById(1).get().then(r => { $(".output")[0].innerText = r.Title; }); |
$pnp.sp.web.lists.getByTitle("Test 1").items.getById(1).get().then(r => { $(".output")[0].innerText = r.Title; });
Add Operation
You can use below script to add an item in a custom list or library:
Without Attachment in list:
First, let’s add new item in custom list with title. Below is the script to add the list item:
$pnp.sp.web.lists.getByTitle("Test1").items.add({ Title: "PnP Item 1" }).then(r => { $(".output")[0].innerText = "Item Added successfully"; }); |
$pnp.sp.web.lists.getByTitle("Test1").items.add({ Title: "PnP Item 1" }).then(r => { $(".output")[0].innerText = "Item Added successfully"; });
Script Code:
Output:
List Output:
With Attachment in list:
Let’s add item with attachment in the custom list.
$pnp.sp.web.lists.getByTitle("Test1").items.add({ Title: "PnP Item 2" }).then(r => { $(".output")[0].innerText = "Item Added successfully"; r.item.attachmentFiles.add("file.txt", "Here is some file content."); }); |
$pnp.sp.web.lists.getByTitle("Test1").items.add({ Title: "PnP Item 2" }).then(r => { $(".output")[0].innerText = "Item Added successfully"; r.item.attachmentFiles.add("file.txt", "Here is some file content."); });
Script Code:
OutPut:
List Output:
Update Operation:
Let’s try to update the existing item using its item ID:
$pnp.sp.web.lists.getByTitle("Test1").items.getById(2).update({ Title: "PnP Updated Title" }).then(r => { $(".output")[0].innerText = "Item Updated Successfully"; }); |
$pnp.sp.web.lists.getByTitle("Test1").items.getById(2).update({ Title: "PnP Updated Title" }).then(r => { $(".output")[0].innerText = "Item Updated Successfully"; });
Script Code:
OutPut:
List OutPut:
Delete Operation
You can delete the item from a list using its item ID:
$pnp.sp.web.lists.getByTitle("Test1").items.getById(3).delete().then(r => { $(".output")[0].innerText = "Item Deleted"; }); |
$pnp.sp.web.lists.getByTitle("Test1").items.getById(3).delete().then(r => { $(".output")[0].innerText = "Item Deleted"; });
Script Code:
Output:
Exception handling in Pattern and Practice JS
You can handle the exception using catch function in PnP JS. Below is the example to handle the exception in PnP JS. In below example, catch block handles the Exception of the PnP JS execution:
$pnp.sp.web.lists.getByTitle("Test 1").items.getById(1).get().then(r => { $(".output")[0].innerText = r.Title; }).catch(function (data) { console.log(data); }); |
$pnp.sp.web.lists.getByTitle("Test 1").items.getById(1).get().then(r => { $(".output")[0].innerText = r.Title; }).catch(function (data) { console.log(data); });
You are now familiar with PNP JS and its basic operations. It is very easy to perform various tedious operations using PNP JS.
Conclusion
Without any solution package, one can perform the CRUD operations on list/library with the beauty of PnP JS. PnP JS Core library can be used in SharePoint Online and On-premises to ease the access of SharePoint data. The complexity of REST API operations on the SharePoint content is simplified by PnP JS. It can be used with Script Editor Web Part, SharePoint Framework and SharePoint Add-ins based implementations.
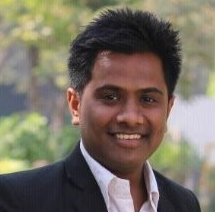
Vishal Shah
Vishal Shah has an extensive understanding of multiple application development frameworks and holds an upper hand with newer trends in order to strive and thrive in the dynamic market. He has nurtured his managerial growth in both technical and business aspects and gives his expertise through his blog posts.
Subscribe to our Newsletter
Signup for our newsletter and join 2700+ global business executives and technology experts to receive handpicked industry insights and latest news
Build your Team
Want to Hire Skilled Developers?
Comments
Leave a message...