Related Blogs
Table of Content
- Java 9 Features List
- Module System (Jigsaw Project)
- JDK 9 Folder Structure
- Java SE 9 Module
- Jshell (Java 9 REPL)
- Reactive Streams
- Multi-Resolution Images
- Private Methods in Interface
- Process API
- Try-with Resources
- Diamond Operator Extension
- Unified JVM Logging
- SafeVarargs Scope Extension
- HTTP 2 client
- HTML 5 Javadoc
- Miscellaneous Java 9 features
- Java SE 9 Deprecated and Removed Features
- End of its line
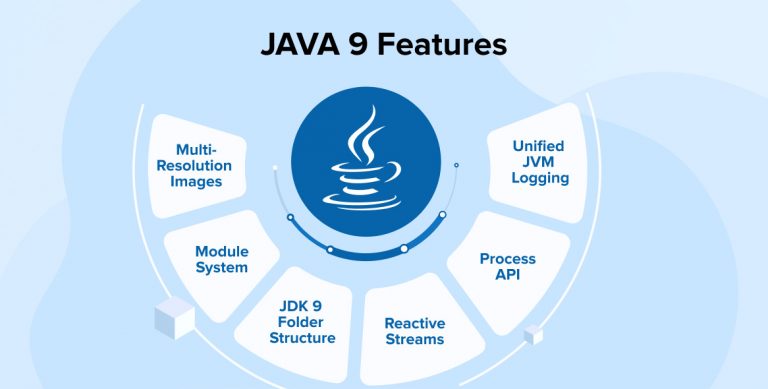
1. Java 9 Features list
After nearly 3 years of disagreements and agreements on controversial Project Jigsaw, Java 9—formally, Java Platform Standard Edition version 9 is finally here. Java SE 9 has various key architectural as well as component changes, making SE 9, the major feature release for Java Platform. The following are mentioned some of the key features and enhancements of Java 9:
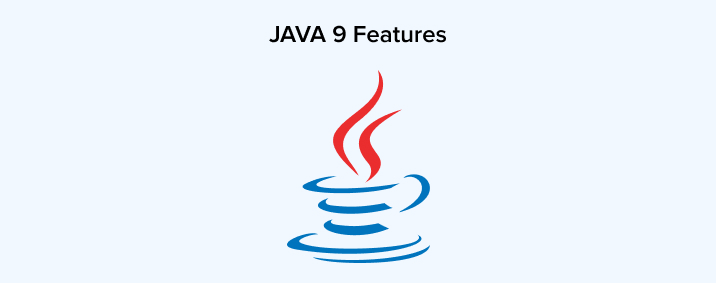
1.1 Module System (Jigsaw Project)
This is a new type of Java programing component that is useful for collecting Java code. Though the most significant feature of Java 9 is the Module System, still it is very controversial. It is released as a part of Jigsaw Project by Oracle Corporation to ease the woes of Java SE previous versions. Following features are part of the Jigsaw Project:
- Modular JDK
- Modular Java Source Code
- Modular Run-time Images
- Encapsulate Java Internal APIs
- Java Platform Module System
As in Java 9, JDK, JRE, JARs etc. are divided into smaller modules, so java developers can implement only those modules that are demanded to develop a particular application. In addition, Module system provides ease of Testing and Maintainability, support to better Performance, support for strong Encapsulation, support to less coupling between components, support to Single Responsibility Principle (SRP) and restricts access to Internal Non-critical APIs.
1.2 JDK 9 Folder Structure:
JDK 9 folder structure, unlike JDK 8 folder structure, doesn’t possess JRE folder. JRE is separated into a different folder named ‘jmods’, that contains a set of Java 9 modules and is available at ${JAVA_HOME}/jmods. ‘jmods’ which has approximately 95 modules collectively known as “JDK Modules”.
1.3 Java SE 9 Module:
Java 9 module is a self-explanatory collection of the following main components:
- One Module
- Module Name
- Module Descriptor
- Set of packages
- Set of types and resources
In Java 9, JDK jars and Java SE Specifications are separated into two sets of modules – All JDK modules start with “jdk”, while all Java SE specifications modules start with “java”. Java SE 9 has a “java.base” module commonly known as base module and acts as the heart of all Java 9 modules (all JDK modules and User-defined modules).
Developers can create their own modules as shown below:
module com.foo.bar { } |
module com.foo.bar { }
Here, ‘module’ is used to create a simple module.
Java 9 application with added component Modules can be understood from the below figure:
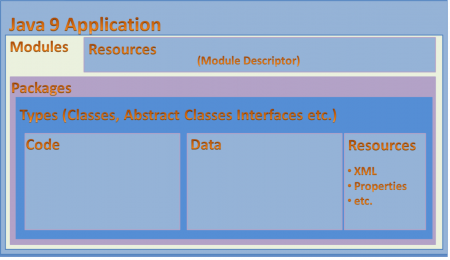
1.4 Jshell (Java 9 REPL)
Java 9 features the Read-Eval-Print-Loop (REPL) tool most commonly known as Jshell. Java 9’s REPL feature interactively evaluates declarative statements and expressions. With this, developers can receive feedback for programs before compilation, just by adding few lines of code. It does not require any IDEs or Editors to execute simple Java development services.
It is used to execute and test any Java Constructs like class, interface, enum, object, statements, etc. very efficiently and quickly.
D:\>jshell | Welcome to JShell -- Version 9-ea | For an introduction type: /help intro jshell> int a = 10 a ==> 10 jshell> System.out.println("a value = " + a ) a value = 10 |
D:\>jshell
| Welcome to JShell — Version 9-ea
| For an introduction type: /help intro
jshell> int a = 10
a ==> 10
jshell> System.out.println(“a value = ” + a )
a value = 10
1.5 Reactive Streams
Reactive programming has become very popular in application development with technologies like Scala,Play, Akka, etc. In Java 9, new Reactive Streams API are introduced to achieve similar features as of reactive programming.
Java SE 9 Reactive Streams API is a Publish/ Subscribe Framework used to implement Asynchronous, Scalable and Parallel applications easily. Java SE 9 has introduced the following APIs to develop Reactive Streams in Java-based applications:
- java.util.concurrent.Flow
- java.util.concurrent.Flow.Publisher
- java.util.concurrent.Flow.Subscriber
- java.util.concurrent.Flow.Processor
1.6 Multi-Resolution Images
A new multi-resolution image API, defined in java.awt.image package, allows a set of images with different resolutions to be wrapped up into a single multi-resolution image. Below mentioned basic operations can be performed on a multi-resolution image:
- To retrieve a resolution-specific image variant based on a given DPI metric and set of image transformations, and
- To retrieve all of the variants in the image
Apart from these operations, a multi-resolution image will behave alike an ordinary image. The java.awt.Graphics class will retrieve the necessary variant from a multi-resolution image based on the current display DPI metric and any applied transformations.
1.7 Private Methods in Interface
In Java SE 8, developers were allowed to write method implementation in interfaces by using Default and Static methods. But this feature led to code redundancy. To remove the code redundancy, one way was to extract common code in a public method which led to a threat of exposing code to other modules and clients directly.
However, we cannot perform private interface methods. From Java SE 9 onwards, we can address private and private static void display card details in an interface practicing the ‘private’ keyword.
Java SE 9 introduced a new feature to interfaces to give answer to the raised issues – Private methods in interface. Interfaces can have any of the following members:
- Constant Variables
- Abstract Methods
- Default Methods
- Static Methods
- Private Methods
- Private Static Methods
Example:-
public interface DBLogging{ String MONGO_DB_NAME = "ABC_Mongo_Datastore"; String NEO4J_DB_NAME = "ABC_Neo4J_Datastore"; String CASSANDRA_DB_NAME = "ABC_Cassandra_Datastore"; default void logInfo(String message){ log(message, "INFO") } default void logWarn(String message){ log(message, "WARN") } default void logError(String message){ log(message, "ERROR") } default void logFatal(String message){ log(message, "FATAL") } private void log(String message, String msgPrefix){ Step1: Connect to DataStore Setp2: Log Message with Prefix and styles etc. Setp3: Close the DataStore connection } // Any other abstract methods } |
public interface DBLogging{
String MONGO_DB_NAME = “ABC_Mongo_Datastore”;
String NEO4J_DB_NAME = “ABC_Neo4J_Datastore”;
String CASSANDRA_DB_NAME = “ABC_Cassandra_Datastore”;
default void logInfo(String message){
log(message, “INFO”)
}
default void logWarn(String message){
log(message, “WARN”)
}
default void logError(String message){
log(message, “ERROR”)
}
default void logFatal(String message){
log(message, “FATAL”)
}
private void log(String message, String msgPrefix){
Step1: Connect to DataStore
Setp2: Log Message with Prefix and styles etc.
Setp3: Close the DataStore connection
}
// Any other abstract methods
}
Here, redundant code has been extracted into a common private method so that API Clients cannot see the crucial code.
1.8 Process API
In the Process API, Oracle team added a couple of new classes and methods to ease the controlling and managing of OS processes. Two new interfaces added in Process API are:
- java.lang.ProcessHandle
- java.lang.ProcessHandle.Info
Process API example
ProcessHandle currentProcess = ProcessHandle.current(); System.out.println("Current Process Id: = " + currentProcess.getPid()); |
ProcessHandle currentProcess = ProcessHandle.current();
System.out.println(“Current Process Id: = ” + currentProcess.getPid());
Some of the information, developers can now obtain from Process instance which includes:
- Whether the process supports normal termination (i.e. any of the “non-forcible” kill signals in Linux)
- The process ID (i.e. the “pid”)
- A handle to the current process
- A handle to the parent process, if one exists
- A stream of handles to the direct children of the process
- A stream of handles to the descendants (direct children, their children, and so on recursively)
- A stream of handles to all processes visible to the current process
- Process metadata such as the full command line, arguments, start instant, owning user, and total CPU duration
1.9 Try-with Resources
Java SE 9 has added a few improvements in Java SE 7’s new exception handling construct: Try-With-Resources, to overcome the flaws in Java SE 7.
Try-With-Resources Example-Java SE 7:
void testARM_Before_Java9() throws IOException{ BufferedReader reader1 = new BufferedReader(new FileReader("test.txt")); try (BufferedReader reader2 = reader1) { System.out.println(reader2.readLine()); } } |
void testARM_Before_Java9() throws IOException{
BufferedReader reader1 = new BufferedReader(new FileReader(“test.txt”));
try (BufferedReader reader2 = reader1) {
System.out.println(reader2.readLine());
}
}
Here, a utility method which creates a BufferedReader object to read the content of a file is created. If the above code snippet is observed, even though there is reader1 referring to the BufferedReader object, a duplicate “reader2” BufferedReader object has been created to use it in Try-With-Resources. It is one small bug or issue in Java SE 7 or 8 versions.
In Java SE 9 to overcome the above mentioned issue, if there is a resource already declared outside the Try-With-Resource Statement as final or effectively final, so now there is No need to declare a local variable. Previously created variable can be used within Try-With-Resource Statement without any issues as shown below:
void testARM_Java9() throws IOException{ BufferedReader reader1 = new BufferedReader(new FileReader("test.txt")); try (reader1) { System.out.println(reader1.readLine()); } } |
void testARM_Java9() throws IOException{
BufferedReader reader1 = new BufferedReader(new FileReader(“test.txt”));
try (reader1) {
System.out.println(reader1.readLine());
}
}
1.10 Diamond Operator Extension
Java SE 9 has provided extension to the Java SE 7 feature with Diamond Operators, in order to remove the limitations and issues with Anonymous Inner Classes. Following code snippet shows the limitation of diamond operator with Anonymous Inner classes in Java SE 7:
public abstract class MyHandler<t>{ //constructor, getter, setter.. abstract void handle(); } // valid code MyHandler<integer> intHandler = new MyHandler<integer>(1) { public void handle() { // handling code... } }; //Invalid in Java SE 7 & 8 MyHandler<integer> intHandler = new MyHandler<>(10) { // Anonymous Class }; MyHandler<!--?--> handler = new MyHandler<>(""One hundred") { // Anonymous Class }; </integer></integer></integer></t> |
public abstract class MyHandler<t>{
//constructor, getter, setter..
abstract void handle();
}
// valid code
MyHandler<integer> intHandler = new MyHandler<integer>(1) {
public void handle() {
// handling code…
}
};
//Invalid in Java SE 7 & 8
MyHandler<integer> intHandler = new MyHandler<>(10) { // Anonymous Class };
MyHandler<!–?–> handler = new MyHandler<>(“”One hundred”) { // Anonymous Class };
</integer></integer></integer></t>
The above code throws compilation error in Java SE 7 & 8 while it runs without compilation error in Java SE 9 due to the Diamond Operator Extension introduced.
1.11 Unified JVM Logging
Java 9 came up with a unified logging architecture (JEP 158) that pipes a lot of messages that the JVM generates through the same mechanism, which can be configured with the -Xlog option especially for complicated Java Software Development.
Java’s new version includes a common logging system and logging framework for all elements of the JVM.
This gives uniform access to log messages from different subsystems such as class loading, threading, garbage collector, module system, or interaction with the underlying operating system.
By simply executing java -Xlog, appending -version gives the below output:
$ java -Xlog -version
# truncated a few messages
> [0.002s][info][os ] HotSpot is running with glibc 2.23, NPTL 2.23
# truncated a few messages
It shows how long the JVM has been running (2 ms), the message’s log level (info), its tags (only os), and the actual message.
1.12 SafeVarargs Scope Extension
Java 7 introduced the SafeVarargs annotation type for asserting that the bodies of annotated final or static methods, or constructors don’t perform potentially unsafe operations on their varargs (variable number of arguments) parameters. Java 9 expands this capability to also include private methods.
SafeVarargs must be used with methods that can’t be overridden because an overriding method could violate its superclass method’s @SafeVarargs annotation by performing an unsafe operation. Static, final, and private methods, and constructors can’t be overridden, so they are used with SafeVarargs.
1.13 HTTP 2 client
New HTTP 2 Client API to support HTTP/2 protocol and WebSocket features has been introduced in Java SE 9. As Legacy HTTP Client API has several issues like supporting HTTP/1.1 protocol and doesn’t help HTTP/2 protocol and WebSocket, it works only in “Blocking mode” and has a lot of performance issues. Now they have a new API HTTP 2 Client that is beneath the “java.net.http” package. This HttpURLConnection API is being replaced with new HTTP client.
This API is being introduced under the “java.net.http” package. It supports both HTTP/1.1 and HTTP/2 protocols and both Synchronous (Blocking Mode) and Asynchronous Modes. The Asynchronous Mode is supported using WebSocket API.
HTTP 2 Client Example
jshell> import java.net.http.* jshell> import static java.net.http.HttpRequest.* jshell> import static java.net.http.HttpResponse.* jshell> URI uri = new URI("http://test/2016/05/java-news.html") uri ==> http://test/2016/05/java-news.html jshell> HttpResponse response = HttpRequest.create(uri).body(noBody()).GET().response() response ==> java.net.http.HttpResponseImpl@79efed2d jshell> System.out.println("Response was " + response.body(asString())) |
1.14 HTML 5 Javadoc
Javadoc is the tool that can generate API documentation in HTML format. In the previous version of JDK, it’s HTML 4.01 – an old standard. JDK 9 Javadoc now supports generating HTML5 markup, improves search capability and Doclint.
-Xdoclint enables recommended checks for issues in Javadoc comments: bad references, lack of accessibility, missing comments, syntax error and missing HTML tags. By default, -Xdoclint is enabled. We can disable it by -Xdoclint:none.
1.15 Miscellaneous Java SE 9 features:
Some of the miscellaneous features of Java SE 9, equally important as the key features, are GC (Garbage Collector) Improvements, Stack-Walking API, Filter Incoming Serialization Data, Deprecate the Applet API, Indify String Concatenation, Enhanced Method Handles, Java Platform Logging API and Service, Compact Strings, Parser API for Nashorn, Javadoc Search, etc.
Stream API Improvements:
In Java SE 9, Oracle Corp. has combined four beneficial new methods to java.util.Stream interface. As a Stream interface, all these new execution methods are essential methods.
Java Collection Factory Methods:
Factory methods are specific kinds of static methods that are applied to produce unmodifiable instances of collections. It suggests that we can utilize these methods to build a list, set, and map of a small number of factors.
Remove Launch-Time JRE Version Selection:
Java 9 has excluded JRE (Java Runtime Environment) version choice at launch time. Now, the latest application has its active installer that additionally contains methods to handle the JRE. That’s why the JRE version choice has been eliminated.
Prepare JavaFX UI Controls and CSS APIs for Modularization:
Java involves public APIs for CSS functionality and JavaFX UI controllers. These functionalities were earlier obtainable only through internal packages, but now it is available because of the modular method.
XML Catalogs:
Standard XML catalog API is computed which helps the company for the Advancement of OASIS XML Catalogs version 1.1.
TIFF Image I/O:
TIFF (Tag Image File Format) is combined for reading and writing as a criterion. It is determined in the package javax.imageio.
Segmented Code Cache:
Code cache is split into different segments. Each segment is a selected code and advances performance and facilitates extensibility.
Filter Incoming Serialization Data:
It supports filtering the date of an incoming stream of object-serialization data to enhance both robustness and safety. Object-serialization clients implement can confirm their input more easily, and transported Remote Method Invocation (RMI) objects can prove invocation cases more easily.
Java 9 Control Panel:
Java control panel is practised to manage Java applications that are installed in the browser. This control panel controls the environments that manage Java applications installed in a browser.
Anonymous Classes Improvement:
Java 9 launched a new feature that enables us to practice diamond operators with an anonymous inner class. Practiscing the diamond with anonymous classes was not supported in Java 7.
OCSP Stapling for TLS:
OCSP (Online Certificate Status Protocol) benefits the server in a TLS link to review for a revoked X.509 certificate repeal.
UTF-8 Properties Files:
The UTF-8 is a convenient method to describe non-Latin characters. The new version of java contains properties files in UTF-8 encoding. In newer versions, ISO-8859-1 encoding was practiced when loading property resource bundles.
CLDR Locale Data Enabled by Default:
CLDR (Common Locale Data Repository) describes the locale data produced by the Unicode CLDR project. It was added in JDK 8 and now default in JDK 9.
Parser API for Nashorn:
Java combined Parser API which enables users to Enable applications, in server-side framework, special IDEs. It can be practiced to parse ECMAScript code from a series, URL, or file with systems of Parser class.
Validate JVM Command-Line Flag Arguments:
Java approves cases to all numeric JVM command-line flags to evade failure. If arguments are wrong or out-of-range, it presents an appropriate error message.
BeanInfo Annotations:
The @beaninfo Javadoc tag is substituted with the explanation types JavaBean, BeanProperty, and SwingContainer. We can practice these corresponding feature attributes immediately in the Bean class.
2. Java SE 9 Deprecated and Removed Features:
The main and foremost amongst them is Applet API which is deprecated as the security-conscious browser makers have been removing support for Java browser plug-ins. Developers are now headed towards alternatives such as Java Web Start, for launching applications from a browser, or installable applications. The appletviewer tool has been deprecated as well.
Concurrent Mark Sweep (CMS) garbage collector is also deprecated with the intention to speed up the development of other garbage collectors in the HotSpot virtual machine. The low-pause G1 garbage collector is intended to be a long-term replacement for CMS.
Java SE 9 excludes Java warnings on import statements to make large code bases clean of lint warnings.
Java SE 9 removes the ability to select the JRE at launch time via the Multiple JRE feature. Also the JVM TI (Tool Interface) hprof (Heap Profiling) agent and the jhat tool are removed as well.
3. End of its line
Oracle Corporation recently revealed that the Java SE 9 is the last of its kind, in terms of the designation and the time elapsed between major Java version releases. After that, the Java developers team has planned to have a six-month release cadence, the next major version is expected to be called Java 18.3, due in March 2018, followed by Java 18.9 six months later.
JDK 9 will not be a long-term support release instead the next long-term support release would be Java 18.9.
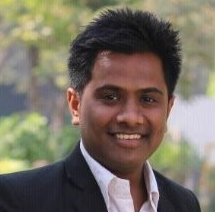
Vishal Shah
Vishal Shah has an extensive understanding of multiple application development frameworks and holds an upper hand with newer trends in order to strive and thrive in the dynamic market. He has nurtured his managerial growth in both technical and business aspects and gives his expertise through his blog posts.
Subscribe to our Newsletter
Signup for our newsletter and join 2700+ global business executives and technology experts to receive handpicked industry insights and latest news
Build your Team
Want to Hire Skilled Developers?
This is a great article about the features of Java 9. In addition to new features, the author also mentions deprecated and removed features. I agree that it is important to be aware of these changes, so that you can update your code accordingly.
This article covers all the important Java 9 features, including the module system, JShell, private methods in interfaces, reactive streams, JDK 9 folder structure, Java SE 9 module, and multi-resolution images. The description of features is clear and concise, and the examples are helpful.
This blog is an invaluable resource for those who are new to Java and want to know everything about Java 9. In this post, every Java 9 feature is explained in detail. Highly recommended!
For developers trying to understand and implement these new Java 9 features, this blog is a great resource. Thank you for sharing this detailed and informative blog!
I was eager to learn about Java 9, and this blog really helped me a lot. Some key features and enhancements of Java 9 are very well explained in this article. Thanks for sharing this insightful information!
Java 9 comes with major architectural as well as component changes. All Java 9 features, like the Module System, JDK 9 Folder Structure, Java SE 9 Module, Reactive Streams, Multi-Resolution Images, etc., are explained in detail in this article. I'll definitely recommend this excellent insights to others.