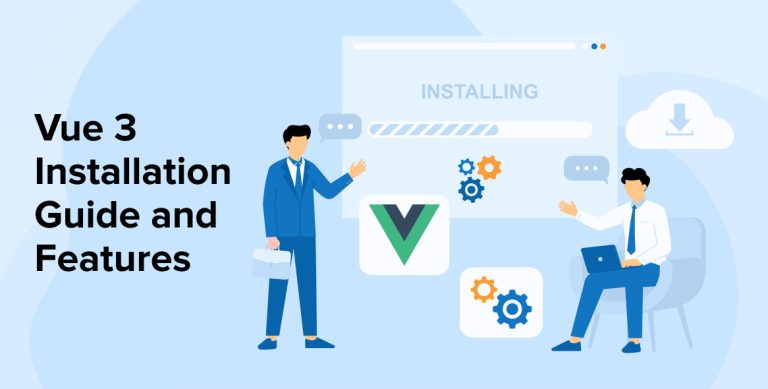
Vue.js is an open-source front-end framework for creating single-page apps and user interfaces that is rapidly rising in popularity. Vue.js has grown in prominence and gained new subscribers since its first release, because of its development-friendly language, ease of use, and well-known documentation. The new Vue version, Vue 3, was launched in September 2020. It’s more compact, easier to maintain, and when used with Vue best practices it comes with more useful functions. Its new release consists of many thoughtful breaking changes—let’s discuss them.
1. Vue 3 Installation Guidelines
Here are some steps to install vue3
1.1 Install Node.js & NPM Package
Download Node.js and NPM (Node Package Manager) on your PC as the main action.
You may determine whether they are already downloaded by navigating to the Terminal/Command prompt window on your system and executing a few instructions.
To determine the model of Node.js version,
node -v |
If it is currently downloaded, ensure that it is version 10 or higher.
To confirm the npm, execute the below code in the terminal.
npm -v |
If they are not downloaded, you will see a warning like “command not found” when performing node and npm versions test commands. Therefore, you will be required to install these.
Navigate to the Node.js portal in the internet browser; you will find two variants; It is recommended choosing LTS, the better reliable version, that is usually preferred for most visitors.
Clicking the LTS button initiates the Node.js package file installing on the PC.
To download the file, double-click the file to open the installation window. Installing Node.js is the same as installing any other software.
Along with node.js, you may also download npm since they do not need to be installed individually.
Click Continue, Continue,
Then click Agree and Install, that will request the administrator passcode. After entering the passcode, it will take a bit of time to finish the setup.
The following step involves installing Vue CLI.
1.2 Install Vue CLI
Launch Terminal and execute the following command:
npm install -g @vue/cli |
A few moments will be required to finish the installation.
If it states that users do not get authorization, rerun the command with the sudo prefix, which will prompt you to provide your administrator passcodes, and you will be good to go.
sudo npm install -g @vue/cli |
To build a vue 3 application, a vue cli version of 4.5 or higher is required.
If you’ve previously downloaded vue cli, you may use the below command to determine its version.
vue —version |
If you have a release that is less than 4.5, you may update it by using the command here.
npm upgrade —next |
The following task is to establish a Vue 3 project.
1.3 Create A Vue 3 Project
To accomplish so, use the terminal and type the following into the input field to access the computer:
cd ~/Desktop |
Then, enter:
vue create my-project-name |
You are then questioned which edition of vue you wish to be using; you may select vue 3 by using the up and down arrow keys on your computer and pressing the enter button.
This will initiate the creation of our Vue 3 project with the title of the project as the file or folder title.
The creation of the vue 3 project and installation of its standard dependencies will require a couple moments.
On the desktop, you may locate the file with the program’s name after it’s completed.
The final step is to execute the vue 3 project in a web browser.
1.4 Run The Project
Your terminal window may provide me with two instructions to execute the project.
The initial step is to navigate to the program file:
CD my-project-name |
To execute the application, use the command below.
npm run dev |
Rather than utilizing the Terminal window, you may execute the project with the help of the Visual Studio Code editor.
Go to the Applications folder → Visual Studio Code
After that, open the file and navigate the created program file.
As you are aware, the Terminal window is associated with Visual Studio, hence you may access it by selecting Terminal from the editor’s toolbar and afterwards New Terminal, which opens a new window. .
If the terminal is already in the project, then you will not need to execute the initial command.
CD my-project-name |
You only need to do the npm run server command.
This command will launch the server on your PC and provide the localhost URL.
After doing so, you may receive two URLs: one is localhost and another is the Network URL, which is useful when you wish to view the program on several devices, like when testing the user interface of the program on a mobile phone.
Copy the Network URL, open the new window on the browser, then paste it into the address bar.
At this point, your Vue 3 application is setup and operating smoothly.
2. New Features in Vue 3
Here are some of the new features in Vue 3
2.1 Composition API
Composition API was first released as a plugin a few months ago, however in Vue 3, we no longer need to deploy it as a plugin. It’s now included in the latest version of the Vue package and may be used right away without any further configuration.
Implementing the Composition API has two major advantages:
- Improved organization
- The code may be shared and reused
Options API will be supported in Vue 3, therefore if you don’t require composition API, you can always utilize the standard Vue 2 techniques.
If you’re unfamiliar with the Composition API, this is how you can build code to create a component:
<template> <div class="counterMessage"> <p><b>Counter</b>: {{ counter }}</p> <p><b>Counter * 2</b>: {{ counterDouble }}</p> <button @click="inc">Increment Number</button> <button @click="dec">Decrement Number</button> <p><b>Message</b> {{ message }}</p> <button @click="changeMessage()">Change Message</button> </div> </template> <script> import { ref, computed, watch } from "vue"; export default { setup() { /* ---------------------------------------------------- */ let counter = ref(0); const counterDouble = computed(() => counter.value * 2); watch(counter, (newVal) => { console.log("counter changed", newVal); }); const inc = () => { counter.value += 1; }; const dec = () => { if (counter.value !== 0) { counter.value -= 1; } }; /* ---------------------------------------------------- */ let message = ref("Sample Text"); watch(message, (newVal) => { console.log("message changed", newVal); }); const changeMessage = () => { message.value = "New Sample Message"; }; /* ---------------------------------------------------- */ return { counter, inc, dec, counterDouble, message, changeMessage, }; }, }; </script> |
And here’s the Options API comparable code:
<template> <div class="counter"> <p><b>Counter</b>: {{ counter }}</p> <p><b>Counter * 2</b>: {{ countDouble }}</p> <button @click="inc">Increment Counter</button> <button @click="dec">Decrement Counter</button> <p><b>Message</b>: {{ message }} </p> <button @click="changeMessage()">Change Message</button> </div> </template> <script> export default { data() { return { counter: 0, message: 'Sample Message' } }, computed: { countDouble() { return this.counter*2 } }, watch: { counter(newVal) { console.log('counter changed', newVal) }, message(newVal) { console.log('message changed', newVal) } }, methods: { inc() { this.counter += 1 }, dec() { if (this.counter !== 0) { this.counter -= 1 } }, changeMessage() { this.message = "New Sample Message" } } } </script> |
We can see that utilizing Composition API helps developers to better organize the code by grouping similar features’ code (state, procedures, calculated variables, viewers, and so on), which was not feasible with the Options API.
In the Composition API instance above, the code for counting and the code for altering a message are clearly divided. As the element develops in size, code organization becomes more critical. Without investing too much time evaluating all of the program code, any software employee may quickly grasp the code.
Programmers used to be able to exchange code using Mixins. However, keeping track of data and procedures across components was difficult, and Mixins had the ability to overwrite present condition or procedures in our elements if we weren’t diligent.
Transferring code is significantly easier when you use the Composition API. As seen below, we may factor out the script for a certain functionality and reuse it in several places:
//message.js import { ref, watch } from 'vue' export function message() { let msg = ref("Sample Message") watch(msg, newValue => { console.log('message changed', newValue) }) const changeMessage = () => { msg.value = 'New Sample Message' } return { msg, changeMessage } } |
Applying the component’s shared code:
<template> <div class="counter"> <p><b>Counter</b></b>: {{ counter }}</p> <p><b>Counter * 2</b>: {{ counterDouble }}</p> <button @click="inc">Increment Counter</button> <button @click="dec">Decrement Counter</button> <p>Message: {{ msg }}</p> <button @click="changeMessage()">Change Message</button> </div> </template> <script> import {ref, computed, watch} from 'vue' import { message } from './message' export default { setup() { let counter = ref(1) const counterDouble = computed(() => counter.value * 2) watch(counter, newVal => { console.log('counter changed', newVal) }) const inc = () => { counter.value += 1 } const dec = () => { if (counter.value !== 0) { counter.value -= 1 } } let { msg, changeMessage } = message() return { counter, msg, changeMessage, inc, dec, counterDouble } } } </script> |
2.2 Suspense
Suspense is a unique component that, unless a criterion is fulfilled, presents a default content instead of your component.
You probably recall all the components in vue 2 you’ve built with v-if=”!isLoading” on the root element and a v-else on the secondary one to present data after it is loaded , including an API call or other concurrent query.
This is the purpose of suspense. The sample syntax in the template below is much better than the v-if one.
<Suspense> <template #default> <MyComponent /> </template> <template #fallback> <div>Loading...</div> </template> </Suspense> |
The mechanism used to determine if your component should be loaded or not. It is included with the Composition API and the below-mentioned setup function. Suspense takes over when the setup function is defined as async, and the fallback is presented after you return from setup.
A snippet example has been given below that may interrupt the fallback:
export default { async setup () { const data = await getMyData() return { data } } } |
This feature minimizes the boilerplate code necessary to perform external calls quite dramatically — and cleanly.
2.3 Multiple V-models
Many firms utilize v-model for two-way binding in Vue.js, and the most popular method to use it is with form elements. You can only use one v-model in a single component in Vue 2, but in Vue 3 one can use multiple v-models. Let’s see how it looks for both below:
Vue 2
<template> <div id="app"> <exampleForm v-model="person" /> </div> </template> <script> export default { data() { return { person: { age: 23 , name: "John " } }; } }; </script> |
Vue 3
<template> <div id="app"> <exampleForm v-model:age="person.age" v-model:name="person.name" /> </div> </template> <script> export default { data() { return { person: { age: 23 , name: "John " } }; } }; </script> |
2.4 Portals
Portal is a feature that allows the display of a portion of code from one component into another component in a separate DOM tree. In Vue 2, there was a third-party plugin named portal-vue that did this.
Portal will be incorporated into Vue 3 and is very simple to utilize. Vue 3 will contain a custom tag called <Teleport> that will allow any code encased within it to be transported to any location.
Let’s look at an example:
<teleport to="#modal-layer"> <div class="modal"> Hello World! </div> </teleport> |
Any code included in <Portal></Portal> will be shown at the specified target location.
<body> <div id="app"></div> <div id="modal-layer"></div> </body> |
2.5 Full TypeScript Support
Vue 3 is now entirely written in TypeScript. TypeScript is a superset of JavaScript. Vue type descriptions enhance TypeScript compatibility because they are automatically created, tested and kept up-to-date. It’s worth noting that TypeScript will still be completely optional in Vue projects. That’s fantastic!
The Vue Core Team also highlighted that after incorporating TypeScript into the Vue core, the system architecture has been greatly reduced and is a lot easier to manage.
2.6 Fragments
Fragments could be referred to as “Multiple Root Nodes,” as well and is one of the React features. In Vue 2, every component is restricted to a single root element. Fragments resolve this issue by enabling the use of many root nodes inside each of them. The notion of multiple root nodes is as simple as it feels. The sole exception is that if you wish to use property inheritance, you must indicate which root components will receive any attributes supplied to the parent component:
<template> <ComponentOne /> <ComponentTwo v-bind="$attrs"/> <ComponentThree /> </template> |
As you may notice in the above code snippet, Vue 3 allows you to use many root nodes immediately without any further work.
2.7 Global Mounting
You’ll find something unusual when you access main.js in the project. To load plugins and additional libraries, developers no longer need to utilize the Global Vue instance unlike previous versions.
However, have a look at the createApp method:
import { createApp } from 'vue' import App from './App.vue' const myApp = createApp(App) myApp.use(/* plugin name */) myApp.use(/* plugin name */) myApp.use(/* plugin name */) myApp.mount('#app') |
The benefit of this functionality is that it shields the Vue app from third-party libraries/plugins that may override or modify the global instance – most commonly via Mixins.
Now, instead of installing those plugins on the global material, you may use the createApp function to install them on a specific instance and it will work properly.
3. Conclusion
If you’re starting a new project, you could still use Vue 2 with a Composition API plugin and then transition to Vue 3 later because there won’t be any major updates other from the loss of filters.
Vue 3 will have a slew of new and exciting features. The unified composition will have a huge influence on future app development by offering a simple method to organize and exchange code, as well as excellent TypeScript support.
The effectiveness will be perfectly alright, and the package capacity will be lowered even further in the next release. Suspense, v-models, and other features will make programming easier than earlier.
Comments
Leave a message...