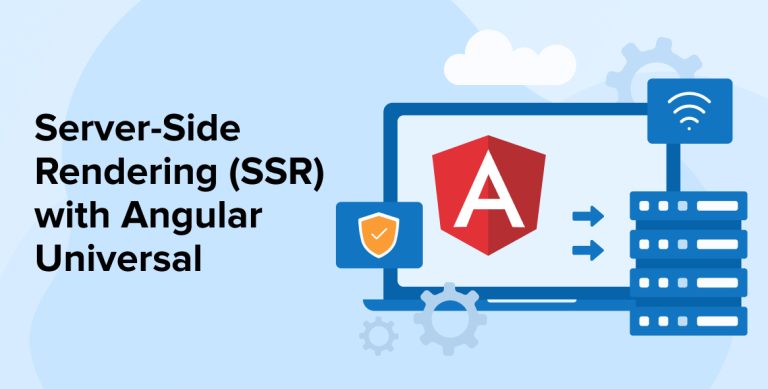
Nowadays, where everything can be done through mobile or web applications, the demand for robust applications has increased. In this competitive world, Angular is one of the most popular frameworks in the market. And applications developed by Angular app development companies are executed on the client browser by Angular server-side rendering rendering the app pages whenever a user visits the app and takes any action. For this, developers need to render the pages before or during build time on the server side for which the Angular Universal concept is utilized.
Angular Universal is an approach that enables the Angular developers to execute it on the server side by creating static application pages and then sending them later on to the client browser for the users to view them. This concept helps in rendering the app quickly which is the reason why it is a very popular approach in the tech world. To know more about Angular Universal and how it is implemented, let’s go through this blog and also have a glance at server-side and client-side rendering concepts here in the article.
1. What is Server Side Rendering?
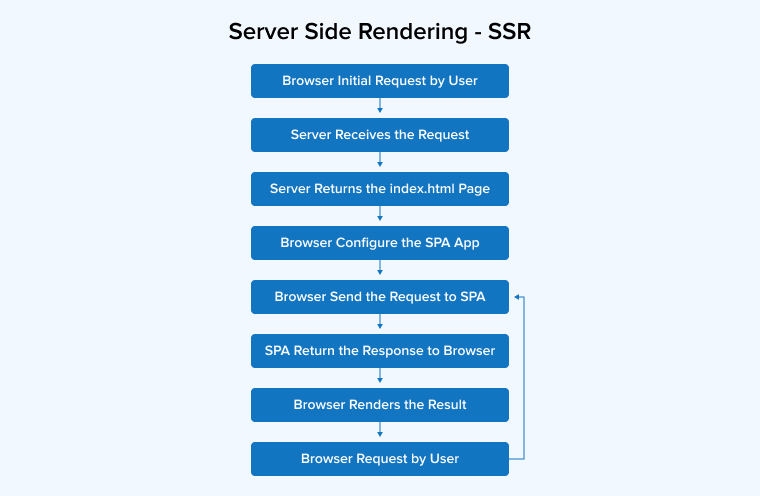
Server-Side Rendering (SSR) is a process that has been around for many years. It is a procedure that enables the conversion of HTML files into fully rendered HTML pages on the server for the client. Basically, this means that with the use of the SSR method, the server will be able to return the static HTML page, which consists of all the client-required information after compiling everything.
Here, when the client clicks something on the web application, the browser sends the request to the server, and then the server instantly responds to the HTTP request with the fully rendered web page which has also been crawled by the search engines using Search Engine Optimization process. Basically, in the server-side rendering process, the server is liable to perform the complete process since the request is sent and it has to go through the same procedure again whenever the route changes. For this, a server-side script which is written in the server-side language is used by the web app development companies in order to fetch the dynamic data from the express server.
Some of the most popular examples of SSR in JavaScript frameworks are –
- Angular Server-side Rendering
- Server-side Rendering with Express
- React Server-side Rendering
- Server-side Rendering with Nest.js
- Gatsby Server-side Rendering
- Vue Server-side Rendering
2. Advantages of SSR
Some of the major advantages of server-side rendering that make the developers use this concept are –
- Server-side rendering is a process that offers a better user experience and it helps the developers to decrease the initial page load time.
- It offers an optimum solution to users that might have issues with their internet connection.
- When server-side rendering is used in any application, it makes the app load faster and this is what benefits businesses ranking higher on the search engine.
- When a page link is copied and sent to others, one can see a pleasant preview of that page. Besides this, when the page link is shared with others or is posted on social media sites, specifying the web page title, description, and image is mandatory. And all these things can be done smoothly when the page is server-side rendered.
3. Angular Universal
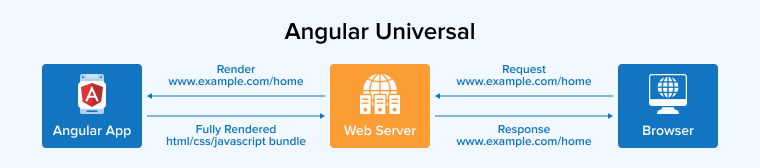
After understanding the concept of server-side rendering and client-side rendering, let’s go through the details that explain the Angular Universal so that later on understanding server-side rendering and client-side application rendering in an Angular application becomes easier.
Angular Universal is one of the most popular pre-rendering solutions for any Angular project. Let us go through an example to understand this concept easily. When there is a normal single-page application, the developers generally develop it in such an easy that the data is brought to the client, and then the HTML is created which will represent the data on the client side. But there might come situations where the Angular app developers might want to render the application ahead of time, for instance, when the application is in its build time. And this is what Angular Universal is used for.
Angular Universal is a concept where the developers initially render HTML and CSS that is shown to the users ahead of time. And this can be done during the on-the-fly approach on the server when the request is sent by the users on the web page and during the application build time. Angular Universal enables the HTML and CSS code to be initially served to the users so that they can see something on the screen after they send a request.
Besides this, the server-side rendering is not the complete thing as with the server-side rendered HTML, a simple client-side Angular app shipment to the web browser is also required. And by doing so, the developers will enable the Angular application to take over the web page which will make the application work like a normal single-page app. This means that all the runtime rendering can now occur directly on the server and returns to the client side as usual.
Now, after understanding Angular Universal, before we jump towards the steps that can help you implement server-side rendering with Angular Universal, let’s first go through the benefits of Angular Universal.
4. Benefits of Angular Universal
Here are some of the major advantages of Angular Universal –
- Angular Universal is a concept that helps in improving an app’s user experience as it enables the developers to create applications using a server-side rendering approach which allows the applications to get rendered in the initial view on the server and because of this the loading time of the app in the initial stage is improved.
- It is also good for code portability and sharing which enables the developers to share the application’s code between the client and the server side.
- With the help of the Angular Universal approach, developers can proceed with server-side rendering to help businesses protect their applications against various types of attacks like cross-site scripting (XSS).
- This approach is used by developers to render the applications in a way that web browsers can easily crawl the websites which eventually helps in improving the application’s visibility in search results.
- Angular Universal also benefits when it comes to improving server-side capabilities as it enables the use of Angular applications on the server.
Now, after understanding the Angular Universal benefits, let us go through the steps of implementing server-side rendering with this approach.
5. Steps to implement Server-Side Rendering (SSR) with Angular Universal
Steps to implement Server-Side Rendering (SSR) with Angular Universal
Now, after understanding Angular Universal and server-side rendering, we will see how Angular developers can implement server-side rendering with Angular Universal.
In the following example, we’ll create an Angular application with property binding and implement Server Side rendering with the help of Angular Universal.
Step 1. Install Angular CLI
Before stepping ahead, make sure that you have Angular CLI installed with following command in terminal.
npm install -g @angular/cli |
Step 2. Create New Angular App
Here’s SSR-Angular is used.
ng new SSR-Angular |
Enter the project directory:
cd SSR-Angular |
Step 3. Generate a New Component
For property binding, create a component. Call it example.
ng generate component example |
Step 4. Add Property Binding
The newly created example component, add a property to bind to.
src/app/example/example.component.ts
export class ExampleComponent { message = "Angular SSR using Angular Universal"; } |
In the component’s template, use the property binding syntax.
src/app/example/example.component.html
<p>{{ message }}</p> <!-- This is interpolation, which is a kind of property binding --> |
Step 5. Use the Component in App Component
Add example component.
src/app/app.component.html
<app-example></app-example> |
Step 6. Serve the Application
Run the application.
ng serve |
After running, open the application in the browser at http://localhost:4200/ to check your component displaying bound property.
Here’s the browser output:
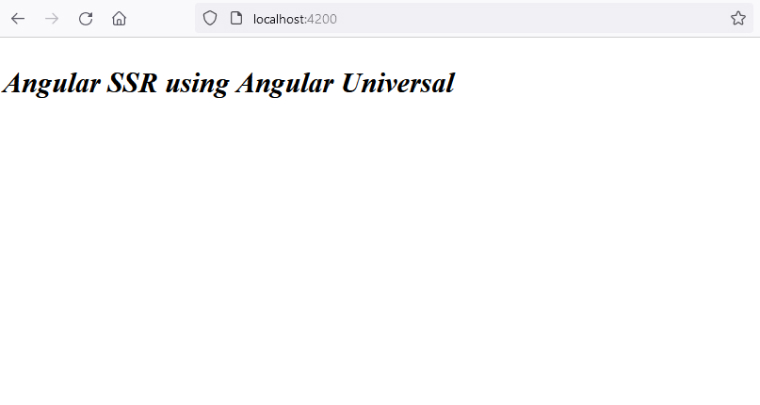
Angular App Without SSR
Here’s the source code of the output.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <title>SSRAngular</title> <base href="/" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <link rel="icon" type="image/x-icon" href="favicon.ico" /> <link rel="stylesheet" href="styles.css" /> </head> <body> <app-root></app-root> <script src="runtime.js" type="module"></script> <script src="polyfills.js" type="module"></script> <script src="styles.js" defer></script> <script src="vendor.js" type="module"></script> <script src="main.js" type="module"></script> </body> </html> |
Step 7. Implement SSR Using Angular Universal
Angular app with property binding is running and displaying. Let’s proceed to implement SSR using Angular Universal. Enter the following command.
ng add @nguniversal/express-engine |
The command will then execute many steps and create necessary configuration.
Adding Node.js Express server code.
Creating necessary configuration for SSR.
Adding scripts to package.json for building and serving the SSR app.
Step 8. Build the App for SSR
Add the following command in terminal to build the app.
npm run build:ssr |
Step 9. Serve the SSR Version of the App
Serve the SSR version to test it locally.
npm run serve:ssr |
App can be opened in browser at http://localhost:4000/. Now, after configuring Angular Universal, the source code has been modified and the updated source code added below.
<!DOCTYPE html> <html lang="en" data-critters-container> <head> <meta charset="utf-8" /> <title>SSRAngular</title> <base href="/" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <link rel="icon" type="image/x-icon" href="favicon.ico" /> <link rel="stylesheet" href="styles.ef46db3751d8e999.css" /> <style ng-app-id="ng"> [_nghost-ng-c536301577] { font-style: italic; font-weight: 700; font-size: 30px; } </style> </head> <body> <app-root _nghost-ng-c1933515739 ng-version="16.2.12" ng-server-context="ssr"> <app-example _ngcontent-ng-c1933515739 _nghost-ng-c536301577><p _ngcontent-ng-c536301577>Angular SSR using Angular Universal</p></app-example> </app-root> <script src="runtime.f6a9aa164cb6b0ac.js" type="module"></script> <script src="polyfills.385fd9000572a4bb.js" type="module"></script> <script src="main.216c6d0f0f761e33.js" type="module"></script> </body> </html> |
After reviewing the source code before and after implementing Angular Universal, you’ll notice significant differences. Previously hidden within <app-root>
, the source code now displays proper HTML that is accessible to search crawlers.
6. Conclusion
In this blog, we learned about server-side and client-side rendering and how it affects app development and process. Besides, we also had a brief discussion about Angular Universal which is a popular approach for rendering applications. It is known as a pre-render builder that helps Angular developers to render the apps on the server side. This process takes place when the user hits the website for the first time. Applying server-side rendering with the Angular Universal approach offers great app performance, accessibility, and also benefits in SEO.
Nice article! This article perfectly explains server side rendering using angular universal. Most important point in this article is it discusses how to implement SSR with Angular Universal with examples.
I really enjoyed reading this article. It covers every essential detail about server side rendering. I liked how effectively the article presented all points.
This article gives a complete explanation of server side rendering. It is a nice resource for developers who want to study about SSR. Article explains how you can use angular universal to create static application pages and send them to the client browser using SSR.