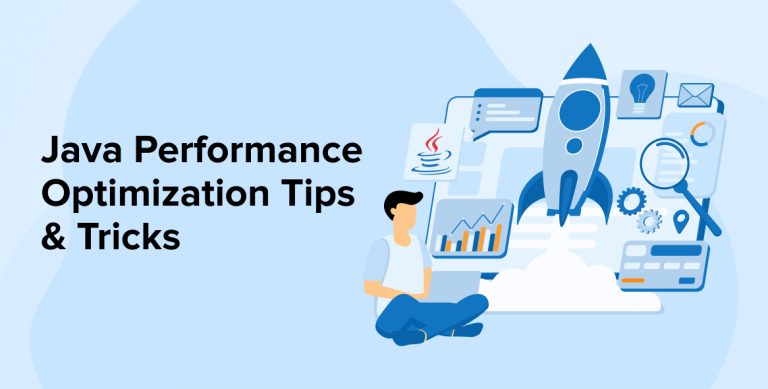
Java is a programming language widely used across various applications. While Java is highly praised for many of its qualities, it is also criticized for its limitations in application performance. However, avoiding performance pitfalls in your Java app development is easier than you might think. You just have to adhere to the best Java performance optimization practices. Implementing them while writing your code is crucial.
This article covers the most common problems that even experienced developers can face if they slip up during coding. This article will also empower you by offering information on the best code optimization tools and techniques to help optimize your Java app code for peak performance. Before diving into optimization, let’s take a look at a few performance issues.
1. Performance Issues in Java
Java performance issues are often related to concurrency, code, and memory. Here are the top five problems that you need to know about:
1.1 Memory Leaks and Out-of-Memory Errors
Every variable is assigned a certain memory in a Java program. When these variables are no longer useful, they should be flushed. But if they aren’t, they continue occupying the memory. This will cause the program to consume more and more memory, leading to memory leaks.
When this happens for a frequently run code and the memory in JVM is severely degraded, it results in OutOfMemoryError. In the worst-case scenario, your Java program will either crash or become unresponsive. Ineffective memory management is the root cause of this issue.
1.2 Thread Deadlocks
Java offers a multi-threading feature. It enables your app to work on various data requests simultaneously. Each thread is granted exclusive access to the JVM resources. This means that the specific data resource is locked when the thread accesses it. A deadlock occurs when two or more threads try to access the same data resource simultaneously.
While one thread gets exclusive access, others have to wait for their turn. This is likely to slow down the app. If the number of requests is beyond a certain limit, it may result in a crash.
1.3 Garbage Collection
If you are experiencing Java performance problems such as increased response time and CPU spikes, then garbage collection may be the reason behind it. It is a process responsible for memory management in your Java application. It automatically allocates and deallocates the memory for your Java code. If there is a Java object that is no longer in use, the garbage collector will remove it.
To do that, garbage collectors need to reclaim the memory, which can prevent threads from accessing the JVM resources. This results in increased response time. Your Java application may slow down when the garbage collector is full.
1.4 Code Level Issues
Most performance bottlenecks happen because of code-level issues. Many reasons contribute to the occurrence of such issues, including inefficient testing, insufficient server load capacity, improperly using generic templates, faulty code constructs, poor data structures, inefficient code, and poor iterations.
These mistakes can lead to a large number of complexities and problems that may impact the app’s performance. Therefore, it is recommended that all code-level issues be rectified before deploying the product.
1.5 Pool Connections
It is costly to establish a connection every time a data request is made. Therefore, Java applications have pool connections shared across the transactions to reduce the load on the database. However, if the total number of data requests exceeds the capacity of the pool connections, the additional requests are queued until the existing requests are fulfilled.
A connection is derived from the pool and, after fulfilling the data request, it is released back into the pool. But if it isn’t released back, it will lead to a leak and display errors to the users.
2. Top Tips to Optimize Your Java Application Performance
Java developers face different kinds of problems during development. So, how can one ensure that the code they are writing will deliver an optimal performance? Well, here are a few tips that could help.
2.1 Use Java Profilers
Addressing the high-impact code issues is the priority. But you have to use a profiler if you don’t know what is causing them. It helps find performance problems and other inefficiencies that exist in your code.
Gathering data from across the Java application and analyzing them allows the Java profilers to determine the performance characteristics of your app. YourKit, JProfiler, and VisualVM are some of the best Java Profilers you can use to conduct profiling for performance degradation.
2.2 Performance Testing
Testing a product in a real environment or under extreme situations to evaluate its performance is known as performance testing. BlazeMeter and Apache JMeter are ideal tools for performance testing.
Profiling is different from this. It is like examining various parts of your code up close to identify any issues. However, performance testing is about implementing your code on different types of devices, browsers, and environments to see how it performs.
2.3 Load Testing
Analyzing how your Java applications perform under varying and peak load conditions is called load testing. In this test, the behavior of the app is measured under normal conditions at first. Then the app is subjected to varying mimicked loads to observe its performance. Special tools you can use to simulate the loads for carrying out load testing are LoadRunner, WebLoad, JMeter, and more.
2.4 Use Prepared Statement
SQL queries are implemented using the Statement in Java. However, the Statements are vulnerable to SQL injections. You can avoid that by using PreparedStatement. They are pre-compiled SQL statements. Unlike statements, prepared statements do not need to be compiled for every new query. This makes them more performant compared to statements. You can easily reuse prepared statements multiple times with different parameters.
2.5 Optimize Strings
In Java, strings are objects but behave a little differently than other objects. The literal syntax is used to create strings; afterward, they are stored in the string pool. Java checks the pool every time a request is made to create a new string. If it finds a similar string, it doesn’t create a new string object and sends the reference to the original.
However, if there is a slight change between the requested and the existing string objects, Java wouldn’t deem them similar and proceed with creating a new string. So, instead of modifying the old strings, you will always end up creating new ones, which are not optimized.
These issues occur because strings are immutable. You can use StringBuilder, a mutable sequence of characters, to modify the strings. However, its application is limited to a single thread. If you need something that is synchronized across multiple threads, you have to use String Buffer.
You can leverage StringUtils to handle strings more effectively. It offers higher-performing methods compared to native string methods. Moreover, if you need to match a pattern, use regular expressions as they are more efficient than iterative pattern matching methods.
2.6 Optimize Java Virtual Machine Garbage Collection Process
Since JVM handles the runtime environment of all Java applications, it is configured by default to meet the requirements of a large range of Java applications and hardware configurations. But when it comes to a unique application or specific environment, some optimization of the JVM may be necessary. Here’s how you can do it:
Pick a suitable Garbage Collector
In Java Development Kit 9, the G1 garbage collector is set as the default option. As the size of the heap memory varies from project to project, G1 is designed to scale to match the requirements. If you are dealing with large heaps of memory with low throughput, then Z garbage collector is an ideal option.
- For using G1 collector: -XX:+UseG1GC
- For using ZGC: -XX:+UnlockExperimentalVMOptions -XX:+UseZGC
Tuning Heap Size
Problems like excessive garbage collection and OutOfMemoryError can be solved by optimizing memory usage and setting heap size. You can utilize the -Xmx and -Xms flags to adjust heap size. The -Xmx flag will help you set the maximum heap size for JVM to allocate whereas the -Xms flag allows you to set the initial size of the heap memory for JVM to start.
Tuning Compiler Options
Some of the flags that you can use to Customize JVM’s behavior are:
- The -XX:MaxGCPauseMillis: option specifies the desired maximum pause time for garbage collection, helping to keep GC pauses within a certain time limit. e.g. -XX:MaxGCPauseMillis=200.
- The -XX:+UseAdaptiveSizePolicy: enables adaptive resizing of the heap regions, not just the tenured generation, based on the application’s memory usage patterns.
2.7 Use Recursion
During a method call, every function is allocated a stack frame in the call stack by JVM. Now, if this function calls another function, then the new function also gets a new stack frame which is placed on top of the call stack.
Recursion is used to provide a set of local variables for every stack frame. Their memory usage is more to store it all. Using recursion helps solve complex performance issues but if you are working with limited memory then it might not be an ideal solution for you.
2.8 Use of Primitives and Wrappers
Just like recursion involves a set of variables, Wrapper classes also include local variables and methods. Therefore, Wrappers consume more memory, while Primitives require less space, making them more efficient. So, if precision is not your priority then you should avoid using Bigdecimal or BigInteger classes.
2.9 Avoid Writing Long Methods
Methods need to be loaded into the stack memory during a method call. Long methods require more memory and processing power. It affects your app’s performance and maintenance.
Therefore, your method shouldn’t be very long and should focus on a single functionality. Including more than one functionality in a method not only makes it long but also complicated. Therefore, you should create small methods with appropriate logic.
2.10 Avoid Overusing Conditional Statements
Java developers should be careful of overusing conditional statements in their code. The more if-else statements you use in the code, the more JVM will have to compare the conditions. So, using multiple conditional statements can affect the Java application’s performance.
The problem worsens if this occurs within looping statements. Instead of using multiple if-else statements, you can use switch statements.
2.11 Use Stored Procedures Instead of Queries
The queries are long and complex. They need to be compiled and executed every time they are called through the app. Using Stored Procedures is much more beneficial. First of all, writing Stored procedures is simple and its execution time is considerably less than queries, even with the same business logic. This is because Stored procedures are pre-compiled and stored in the database as objects.
2.12 Don’t Use Unnecessary Log Statements and Incorrect Log Levels
Unnecessary log statements and incorrect log levels can cause poor Java performance Therefore, it is better to avoid them. It is important to refrain from logging large objects in your Java code. Restrict your logging to specific parameters that you need to observe. It is also recommended to maintain logging at higher levels like ERROR and DEBUG rather than INFO.
2.13 Select Required Columns in a Query
Queries are tasked with fetching data from the database. In this process, you have to select the columns that will be processed. Make sure that you select the necessary columns that are needed for display on the front end or for processing.
Avoid selecting columns that don’t need processing because it may cause a delay in the query execution in the database. Selecting too many columns also increases network traffic from the database to the application, which can harm the Java application performance.
2.14 Fetch the Data Using Joins
Developers often use subqueries to gather data from multiple tables, which can take time for execution. Instead, use Joins for data collection as their execution time is less than that of subqueries. But you have to use Joins properly as well as normalize the tables. Otherwise, it will slow down the implementation and your Java application performance will be affected negatively.
You can create an index on the table’s columns, as it frequently helps reduce the app latency and improve performance by decreasing the execution time.
2.15 Use Caching and Memoization
Caching methods like LRU cache or memoization help save the results of resource-intensive operations. This method avoids repeating expensive calculations. Caching and memoization also enable you to reuse these saved results. This significantly improves your application’s performance optimization.
2.16 Use Lazy Initialization
The process of creating an object only when its requirement arises is known as lazy initialization. This technique is useful in cases where an object is not always needed or when its creation is very expensive. Lazy Initialization helps improve Java performance. However, it may face synchronization issues in a multithreading environment.
3. Best Tools For Java Performance Tuning
The optimization techniques discussed above have given you some clarity on how to optimize your Java code. But to implement those techniques, you need some robust and reliable tools. Therefore, here is a small list of the top tools that can help you with Java performance tuning.
3.1 Apache Maven
Maven is a Java project management tool. It is a one-stop storage solution to save all your documents, reports, and even the project‘s build. Built by Apache, Maven is an opinionated tool that gives clear definitions of how to build Java projects. Unless your project has a complicated build structure, Maven is very easy to use.
3.2 Mockito
Mockito is a framework used by developers for creating mock objects in unit tests. Mock objects help create simulated interactions and behavior. Using these dummy objects will give you an idea of how your app might behave when interacting with real-world objects.
3.3 Gradle
Gradle is a build automation tool. It also helps you out with dependency management. You can easily integrate it with various plugins. Because it is fast, Gradle is largely used for complex data structures.
Moreover, it uses domain-specific language over XML. So, both the clutter and the size of the configuration files are reduced. Gradle is also known for providing advanced analysis and debugging features.
3.4 Groovy
Groovy comes with robust syntax and expressive language features that help increase the productivity of the developers. It supports metaprogramming, scripting, and testing. Because it is open-source, Groovy enjoys the support of a large and active community. It is a high-impact language that provides great interoperability with Java.
3.5 JMeter
JMeter is useful for load testing. Developers can leverage this open-source tool to measure the functional load and web performance on different types of servers. JMeter also allows you to conduct functional and automated testing of your application.
3.6 JUnit
JUnit is a Java-based unit testing tool. It enables developers to write test cases to check if their code works as per expectations. It analyzes the code for potential errors and helps in solving them quickly.
3.7 EhCache
Ehcache is an open-source, and feature-rich caching solution. It can seamlessly integrate with Jira, GitHub, Spring, and Hibernate. This Java-based solution provides effective caching capabilities for building lightweight apps. Ehcache has an extensive architecture that allows for easy customization. It is open source but has minimal dependencies, which helps reduce various deployment and maintenance complexities.
3.8 Eclipse
Eclipse is one of the best Java IDEs. developers prefer this open-source tool for JEE projects. It provides very detailed reports on code optimization. You can smoothly integrate Eclipse with JUnit.
4. Conclusion
Being a feature-rich programming language, Java is used for developing various types of software applications. However, developers have to be wary of certain issues while writing Java programs as it can have a negative impact on your app performance.
In this article, we explored the common problems that developers should avoid and discussed the Java performance optimization tips. From using Java Profilers to Lazy initialization, each practice is very helpful for improving the overall performance of your code. We also take a look at some of the best Java coding tools that can help you develop and deliver a robust and reliable application.
It is important to remember that code optimization is a continuous process. You can get the best results by continuously analyzing and modifying your Java programs.
FAQs:
How to optimize performance in Java?
Here are a few dos and don’ts you need to consider for optimizing the performance of your Java application.
Dos:
- Use Caching and memoization
- Use Java profilers
- Use Lazy Initialization
Don’ts:
- Don’t overuse conditional statements
- Don’t write long methods
- Don’t use incorrect log levels and unnecessary log statements
What is Java optimization?
The process of improving the speed and efficiency of Java applications is known as Java optimization. It includes identifying and fixing the underlying issues in the code and architecture of the application. Java optimization ensures that your app performs efficiently and meets user expectations by providing an enhanced user experience.
What is the code optimization tool in Java?
A tool designed to improve Java code performance is known as a code optimization tool in Java. it helps ensure effective memory management, optimization, and quick implementation of the code without altering its behavior.
How to code in Java efficiently?
Adhering to the best programming practices can help you code efficiently in Java. Optimizing JVM garbage collection and Strings, using PreparedStatement, recursion, and Stored procedures are some of the best tips to optimize your Java code for enhanced efficiency and performance.
Comments
Leave a message...