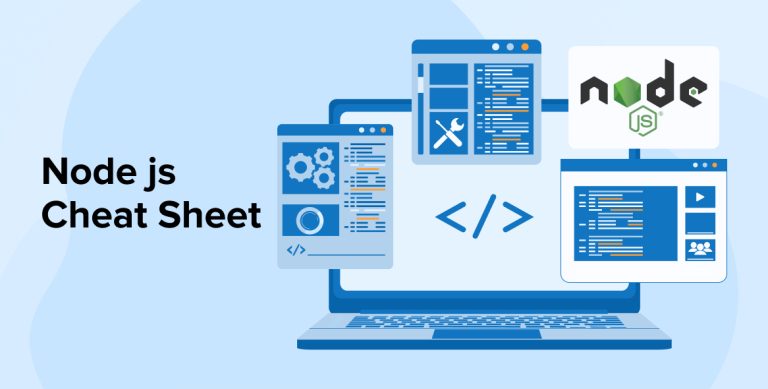
In this world of technology, the developers who want to use Node.js, a popular JS-based runtime environment for creating a scalable app, require proper knowledge to use best development practice of Nodejs and this can be done by following some references. And this is what we are going to review in this blog. We will go through Node js cheat sheet that will be helpful to both beginner and experienced developers.
1. Introduction to Node Js Cheat Sheet
Node.js, an asynchronous event-driven JS-based runtime, is designed in such a way that it can easily create scalable network applications. We will see how Node.js works in the following instance of “hello world”. Here you will be able to see that there are many different connections that can be handled concurrently. A callback is fired for each connection but if there is no task then Node.js goes to sleep.
const http = require('http'); const port = 3000; const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello World'); }); server.listen(port, () => { console.log(`Server running at port ${port}`); }); |
This is completely opposite to the concurrency model that we see today. The models nowadays have OS threads that are employed. This means that they offer thread-based networking which is inefficient and a bit difficult. On the other hand, Node.js is a technology that enables its users to be free from worries of the processes being dead-locked and this is because there are no locks. In Node.js, there are no such functions that can directly perform input/output with the use of synchronous methods. The main reason behind it is that nothing has the capability to block the scalable system.
Node.js is a system that is similar to and influenced by Python’s Twisted and Ruby’s Event Machine. But Node.js is a runtime environment that takes it to another level. This technology has the capability to present an event loop as a runtime and not as a library. Other systems than Node.js have to always start the event loop by initiating a blocking call.
Generally, in other systems, the behavior is defined via callbacks at the starting of a script and then at the end, the server will be started via EventMachine::run(), a blocking call. But in Node.js, we don’t have any start-the-event-loop call. It is a technology that can simply enter the event loop once the input script is executed. And exists the event loop after no callbacks are not left to perform.
Now after having a brief understanding of the Node and how its code works, let us go through the top node js cheat sheets of this technology.
2. Top Node Js Cheat Sheets
Here are some of the most popular cheat sheets of Node.js that every developer must-have for their quick reference.
2.1 Node.js Global Object
Node is one such technology that offers access to various global objects that are important to use with the program files of Node, a runtime environment. When a developer is writing a file that can run on the Node environment, the variables of this program files can be accessed in the global scope of the written file. This means that the developer can access the global objects by either writing in console.log(global) or global in the terminal. And if you just want to have a look at the keys, you can use Object.keys(global). And as the global is an important object, one can assign new priorities to it through global.name_of_property = ‘value_of_property’.
//Two ways to access global > console.log(global) //or > global //Adding new property to global global._ = require("lodash"); > |
2.2 Node.js Modules
The files in Node.js are known as modules. Modularity is nothing but a technique where one program comes with various parts and each of these parts offers a single piece of the overall functionality. This is just like different pieces of a puzzle getting together to complete the entire picture. For this, developers use require() function and bring smaller modules together into one.
// Custom Modules const authService = require('./authService.js'); // In built Modules const express = require('os'); |
2.3 Node.js Core Modules
Node comes with several modules that come with the environment that has the capability to perform the common tasks excellently. And these modules are called core modules. These core modules of Node.js are specified within the source of this technology and are situated in the lib/ folder. Besides this, the core module is something that any developer can easily access if they correctly pass a string with the module’s name into the require() function.
const util = require('util'); |
2.4 Listing Node.js Core Modules
To list all the core modules that are available in Node.js in the REPL, the developer must use the property, builtinModules of the module. This is one of the new properties of this technology that is useful to verify if a module is handled by a third-party or Node.js.
> require('module').builtinModules |
2.5 Node.js Process Object
A process is an example of a computer program that one can execute. Node comes with some popular global processes that have useful properties. NODE_ENV is one of these properties that developers use in the statement of if/else to perform various different tasks as per the app’s development phase.
if (process.env.NODE_ENV === 'development'){ // development only code here like more verbose logging } |
2.6 The Console Module
The Node.js console module enables exporting of a global console object that offers functionalities just like the JavaScript console object. It also enables the developers to use console.log() and other similar methods to debug. And as it is a global object, there is no need to have it into a Node file.
console.log('Hello Node!'); // Logs 'Hello Node!' to the terminal |
2.7 The OS Module
The Node.js os module is used by developers to gather more data about the operating system and computer on which a program is going to run. Network interfaces, system architecture, system uptime, and the computer’s hostname are some of the best examples of data that can be retrieved with the use of this module.
var os = require('os'); const freeMemory = os.freemem(); const totalMemory = os.totalmem(); console.log("Free Memory: " + freeMemory); console.log("Total Memory: " + totalMemory); |
2.8 The Util Module
One of the last Node js cheat sheet in this blog is util module. The util Module contains utility functions that are useful for application and module developers as well.
Some of the most frequent uses of this include turning callback-based functions to promise-based functions using util.promisify().
util.promisify() |
By default Javascript follows asynchronous programming where callbacks based functions is used. But there is a limitation in callback based functions which is called Callback Hells.
promisify() function that converts callback-based functions to promise-based functions. This lets you use promise chaining and async/await with callback-based APIs.
For example, Node.js fs package uses callbacks. In order to read a file, callbacks are used:
const fs = require('fs'); fs.readFile('./package.json', 'utf8', (err, text) => { if (err) { console.log('Error', err); } else { const packageJson = JSON.parse(text); console.log(packageJson.name, packageJson.version, packageJson.description); } }); |
You can use util.promisify() to convert the fs.readFile() function to a promise based function:
const fs = require('fs'); const util = require('util'); const readFile = util.promisify(fs.readFile); readFile('./package.json', 'utf8') .then((text) => { const packageJson = JSON.parse(text); console.log(packageJson.name, packageJson.version, packageJson.description); }) .catch((err) => { console.log('Error', err);} ); |
3. Let’s Explore More About NodeJs
Node.js is one of the most popular server-side JavaScript runtime environments. It enables the developers to easily create a scalable and fast network application. There are many different companies combines in the market that have adopted Node.js and this is only because of its non-blocking I/O and event-driven model, which makes app development an efficient and lightweight process.
The node js cheat sheet enables the app developers to master some of the most useful command-line flags which are helpful in customizing the behavior of Node.js. It will also help in saving a lot of energy and time that goes behind looking up for various development tasks like scripting, debugging, and monitoring the app.
4. Conclusion
As seen in this blog, there are many different cheat sheets when it comes to a quick reference for the Node.js developers. We have tried to convey all the concept references in the simplest format possible through our blog on node js cheat sheet. For every developer, referring through a cheat sheet is an absolute path if he wants to create an amazing solution using this technology.
More Resources on Cheat Sheet:
React Cheat Sheet
Angular Cheat Sheet
This blog post is a fantastic resource for both beginner and experienced Node.js developers.It's well-written, informative, and easy to understand. Article covers a wide range of topics, from global objects to modules, core modules, the process object, console module, OS module, and the util module. These cheat sheets simplify complex concepts and provide quick access to essential information, saving developers valuable time and effort.