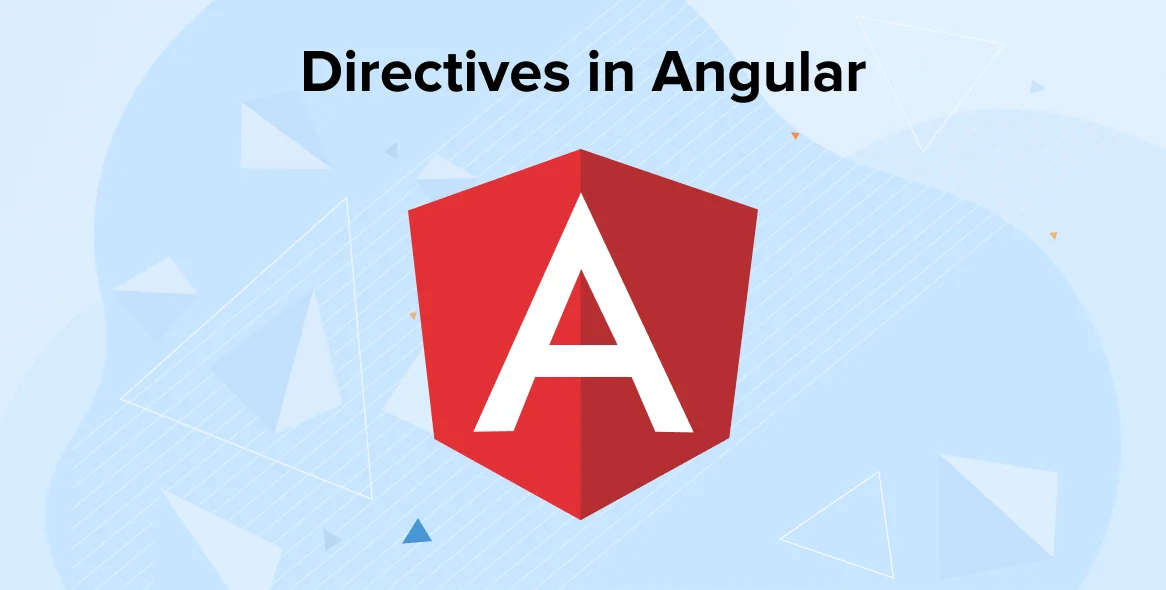
In this post, we’re going to know what are Angular directives and their examples in detail for a better understanding. Later moving ahead, we will discuss the types of Directives in Angular and focus on writing custom directives in Angular.
Before we proceed ahead, let us first understand what are directives in Angular.
1. What are Directives in Angular?
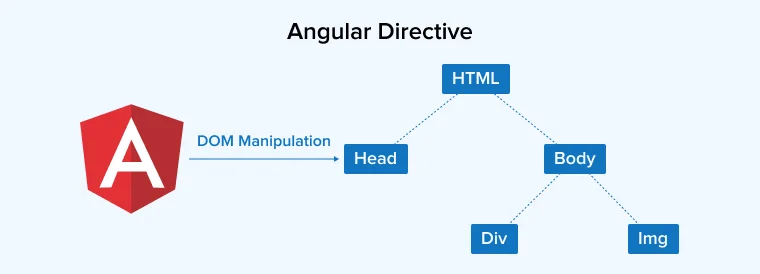
Directives in Angular are functions that will automatically execute whenever the Angular compiler finds them which enhances the capability of HTML elements by binding custom behaviors to the DOM. Basically, developers use Angular directives to manipulate the DOM, for instance, by using Directives Angular development company can easily add or delete an HTML element from the DOM or change the appearance of the DOM host elements.
From the core concept, directives in Angular are categorized into three main categories.
- Components
- Structural directives
- Attribute directives
Apart from this, if you’re planning to build an Angular application that ensures optimal implementation, provides better performance, and has enhanced security, then make sure to follow the best security practices for Angular.
So let us now discuss the three main types of directives in Angular in detail with example for better understanding.
2. Types of Directives in Angular
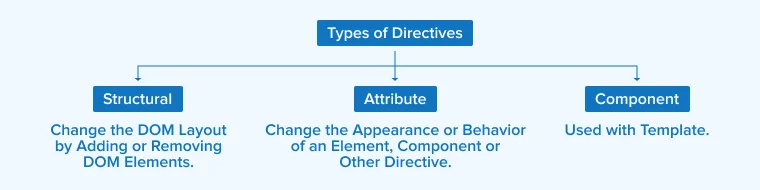
2.1 Component Directives
n Angular, components are directives with templates. Component directives have templates whereas Structural and Attribute directives don’t have templates. Instead, they’re tailored to DOM manipulation. The component directive comes with template or template URLs that represent something in DOM. So we can say that the component directive is a cleaner version of the Directive as it comes with a template, which is easier to use.
In component directives, you’ll find three main parameters which include:
- (i) selector: It represents the template tag which specifies the starting and end of the Component.
- (ii) templateUrl: It defines which particular template is for the component.
- (iii) styleUrls: It includes all the types of fashion formats for the actual component.
For better understanding, let’s take an example: change-user. directive
import { Directive } from '@angular/core'; @Directive({ selector: '[changeUser]' }) export class ChangeUserDirective { constructor() { } } |
app.component.html
<div style="text-align:center"> <span changeuser="">User: {{title}} is Created.</span> </div> |
change-user.directive.ts
import { Directive, ElementRef} from '@angular/core'; @Directive({ selector: '[changeUser]' }) export class ChangeUserDirective { constructor(Element: ElementRef) { console.log(Element); Element.nativeElement.innerText="Username is changed by changeUser Directive. "; } } |
You can also check the output in the console.log.
2.2 Structural Directives
This is the type of directive that is responsible for making a change to the structure of the DOM or we can say editing the layout of the DOM. You can add or delete the elements from the DOM to change or alter the element’s behavior or appearance.
One can easily identify the difference between Attribute and structural directive just by looking at the syntax. The Attribute directive doesn’t contain any prefix whereas when it comes to Structural Directive, it always starts with an asterisk. In short, we can say that Structural directives are specially designed to build and destroy DOM elements. If you have ever worked with Angular and come across directives, then you must be knowing that the three most commonly used built-in structural directives in Angular are:
- NgIf
- NgFor
- NgSwitch
Still confused? Don’t worry, we have an example for you.
Here is the example for Nglf.
<div *ngif="”product”">{{product.name}}</div> <div template="”ngIf" product”="">{{product.name}}</div> <ng-template [ngif]="”product”"> <div>{{product.name}}</div> </ng-template> |
Example for NgFor.
<div *ngfor="let product of products">{{product.name}}</div> <div template="ngFor let product of products">{{product.name}}</div> <ng-template ngfor="" let-product="" [ngfor]="products">{{product.name}} </ng-template> |
And example for NgSwitch.
<div class="row" [ngswitch]="selectedValue"> <div *ngswitchcase="'One'">One is Pressed</div> <div *ngswitchcase="'Two'">Two is Selected</div> <div *ngswitchdefault="">Default Option</div> </div> |
2.3 Attribute Directives
Lastly, we have Attribute directives. Such directives manipulate the appearance and behavior of DOM elements. Developers can use Attribute directives when they want to change or alter the style of DOM elements and show or remove elements according to a changing property. You might know that Angular offers various built-in Attribute Directives like NgClass, NgStyle, and much more.
To change the style of any DOM element based on certain conditions, you need to execute.
<p [ngstyle]="{'background': isRed ? 'red' : 'blue'}"> This is an Attribute Directive</p> |
Still not sure? Here is the example where you want to use a new hoverDirective, make sure to add a new paragraph element to the template and then apply the directive as an attribute.
src/app/app.component.html
<p>content_copy<hoverme> hover this!</p> |
Now run the application.
3. Creating Custom Attribute Directive
If you’re already familiar with Angular components and know how to create them then you can easily create a custom directive where you need to replace the @Component decorator with the @Directive decorator. Attribute directive aims to change the color of the text whenever the point glides over it.
You’ll be surprised to know that creating your own attribute directive is similar to creating the Angular component. But before this, you must
- Create a class with @Directive
- After creating a class, create an Attribute directive having Element Ref
- Listen to the Hover event with
- HostListener
Make use of handlers, the element is referenced, and the text color is changed.
import{Directive, ElementRef, HostListener} from '@angular/core'; @Directive({ selector:'[highlightcolor]', }) export class HighlightColorDirective{ constructor (private eleRef: ElementRef){ } @HostListerner ('mouseover') on MouseOver(){ this.eleRef.nativeElement .style.color ='red'; } @hostListener('mouseleave') onMouseLeave(){ this.eleRef.nativeElement.style.color='green' ; } }] |
For instance, let us say that you’re using an Angular 2 quickstart package. So you need to clone the repository. Once your repository is cloned, make sure to run npm install and npm start to provide a boilerplate app.
Now create a file called app.myerrordirective.ts on the src/app folder and add the below-mentioned code.
import {Directive, ElementRef} from '@angular/core'; @Directive({ selector:'[highlight-error]' }) export class MyErrorDirective{ constructor(elr:ElementRef){ elr.nativeElement.style.background='red'; } } |
Now add the following code to emphasize the constructor of your class and utilize the recently created directive. Make sure you’re adding this directive to the declarations on the app.module.ts file.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { MyErrorDirective } from './app.myerrordirective'; import { AppComponent } from './app.component'; @NgModule({ imports: [ BrowserModule ], declarations: [ AppComponent, MyErrorDirective ], bootstrap: [ AppComponent ] }) export class AppModule { } |
Now, use the recently created directive. So go to the app.component.ts file and add the following:
import { Component } from '@angular/core'; @Component({ selector: 'my-app', template: `<h1 highlight-error="">Hello {{name}}</h1>`, }) export class AppComponent { name = 'Angular'; } |
Now your final result will look like this.

What’s next?
Let’s learn how to create your own structural directive with example for better understanding.
4. Creating Structural Directive
In the above-mentioned section, we discussed how to create a custom attribute directive using Angular. The process of creating a structural behavior is exactly the same. Here we need to create a new file having the directive code and add declarations. Now you’re all set to use it in your component. For structural directive, implement a copy of the nglf directive. There is a reason why we’re doing it this way. We also need to look at how Angular can custom directives to handle behind the scenes rather than only implementing the directive.
We will start with our app.customifdirective.ts file:
import { Directive, Input, TemplateRef, ViewContainerRef } from '@angular/core'; @Directive({ selector: '[CustomIf]' }) export class CustomIfDirective { constructor( private templateRef: TemplateRef<any>, private viewContainer: ViewContainerRef) { } @Input() set CustomIf(condition: boolean) { if (condition) { this.viewContainer.createEmbeddedView(this.templateRef); } else { this.viewContainer.clear(); } } } |
Now add it to our declarators
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { MyErrorDirective } from './app.myerrordirective'; import { MyCustomIfDirective } from './app.mycustomifdirective'; import { AppComponent } from './app.component'; @NgModule({ imports: [ BrowserModule ], declarations: [ AppComponent, MyErrorDirective, MyCustomIfDirective ], bootstrap: [ AppComponent ] }) export class AppModule { } |
After adding to the declarators, use it in your components
import { Component } from '@angular/core'; @Component({ selector: 'my-app', template: `<h1 my-error="">Welcome {{name}}</h1> <h2 *customif="condition">Welcome {{name}}</h2> <button (click)="condition = !condition">Click</button>`, }) export class AppComponent { name = 'Angular'; condition = false; |
Now that you already know how to create structural directives, let us now discuss how to create components manually while you’re building an Angular application.
5. Creating Components Manually
As we all know, one of the best ways to create an Angular component manually is the Angular CLI. Here is the process of creating the core component file within an existing Angular project.
If you’re a developer and planning to build an Angular application and want to create a new component, you need to
- Go to your Angular project directory.
- Now, create a new file, <component-name>.component.ts.
- On the top of the file, you need to add the below-mentioned statement.
import { Component } from '@angular/core';
- Now add a @Component decorator.
@Component({ })
- Once you have added @Component decorator to your Angular application, now you need to choose a CSS selector for the component.
@Component({ selector: 'app-component-menu, })
- Don’t forget to define the HTML template because a component is used to display information. In some cases, you might see that this template is a separate HTML file.
@Component({ selector: 'app-component-menu, templateUrl: './component-menu.component.html', })
- Choose the component’s template style. Make sure you’re not defining details of the component’s template in the same file, define them in a separate file.
@Component({ selector: 'app-component-menu, templateUrl: './component-menu.component.html', styleUrls: ['./component-menu.component.css'] })
- Remember that you need to add a class statement that has the code for the component.
export class ComponentMenuComponent {<br> <br>}<br>
6. Conclusion
In this post, we discussed Angular directives and the different types of directives to create custom ones that suit your requirements. Directives in Angular are considered the building blocks of every application and are very important. Developers can also use built in directives in the conditions, loops, for animating, and much more to build engaging applications and operate a variety of data smoothly. Lastly, we can say that Angular Directives are the most important bit of an Angular application. Also, you’ll be surprised to know that one of the most used units in Angular by developers is actually a directive. We can say that an Angular component is not more than a directive as it comes with a template.
Apart from this, many multinational companies are using Angular like PayPal, Google, Facebook, etc because of its excellent features offerings. It allows developers to build rich web applications and create interactive client-side applications using the most popular JavaScript front-end framework.
So that’s it for the post. We hope you find this post helpful. If you still have any queries running in your head, please don’t hesitate to mention them in the comment section given below and we will get back to you soon. Also, if you think we have missed out something important to add in this post, do let us know in the box, we will really appreciate your efforts!
Thank you and happy reading!
This article is a great introduction to Angular directives. You are able to present the topic in a way that was understandable to newcomers shows that you have a thorough understanding of the subject.
This article provides a comprehensive breakdown of Angular directives and their various types. It's incredibly helpful for anyone looking to dive into Angular development, whether they're new to the framework or seeking to enhance their skills.