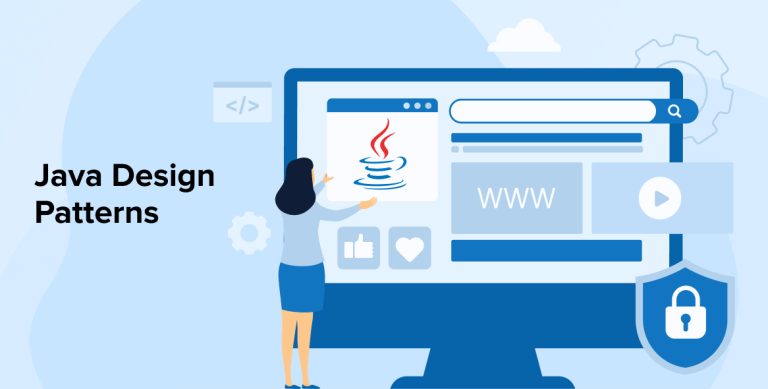
1. What is a Design Pattern?
Design patterns are reusable frameworks that are used to solve recurring design challenges of any Java development company. They function similarly to a set of blueprints from which you can deviate while attempting to address a recurrent design issue in your code.
You can’t simply locate a pattern and paste it into your code as you would with pre-made libraries or pre-defined functions. A pattern is an approach to solving an issue rather than a single line of code. By following the pattern’s instructions, you may create a workaround that is tailored to your own code.
Because both patterns and algorithms represent common approaches to solving specific issues, the two are sometimes used interchangeably. Design patterns in Java are more of an abstract description of a solution, whereas an algorithm always describes a distinct set of steps that can be taken to reach a certain end state. When the same pattern is applied to two separate programs, the resulting code might be different.
Algorithms are similar to recipes in that they both specify the actions to be taken to complete a task. A pattern, on the contrary, is more akin to a blueprint in that it lays out the end product and its characteristics but leaves the specific sequence of execution up to the developer.
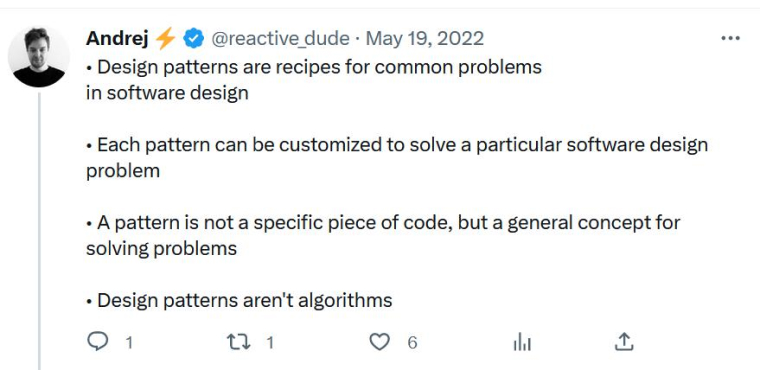
2. Advantages of Design Pattern
- They are able to be used in a variety of different endeavors.
- Solutions provided by them aid in articulating the overall structure of the system.
- They sum up what it’s like to work in software engineering.
- They make an application’s architecture more clear.
- Since they are the result of the labors of experienced programmers, these solutions have stood the test of time.
- The use of design patterns is not a certain way to fix any issue. They make it possible to better understand the system’s architecture and design.
3. What are the Types of Design Patterns?
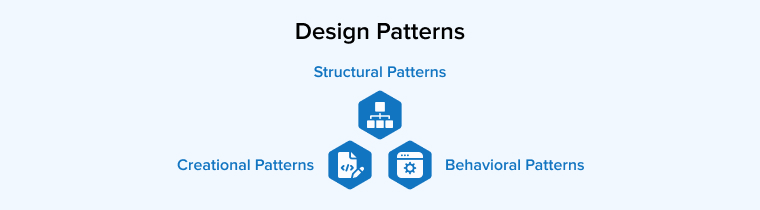
3.1 Creational Patterns
As their name implies, creational patterns are primarily concerned with the act of creating objects. These patterns are meant to be used for creating instances of classes. Using the standard approach to create the necessary objects might add unnecessary difficulty or even cause design flaws in the underlying software. This issue is addressed via creational design patterns.
When solving an issue in software design, when should you turn to creational patterns? To maximize their value, creational patterns work best when they can be applied to instances of several classes. To be more specific, if your program uses polymorphism and has to choose between different classes at runtime rather than compile time, then you will need to use runtime type checking.
The purpose of creational design patterns is to help developers create an Object in the most effective way feasible.
Abstract Factory Pattern
The abstract factory pattern is a factory of factories that resembles the pattern. If you’re acquainted with the Java factory design pattern, you’ll recognize that we utilize a single factory class to return different subclasses depending on the parameters sent in. The if-else block is not needed in an abstract factory pattern since each subclass has its own factory class, and the abstract factory class simply takes the input factory class and returns the appropriate subclass.
Builder Pattern
When an object has many properties, the factory and abstract Factory design patterns can become cumbersome to use, therefore the builder pattern was developed as an alternative. By offering a function that returns the completed Object, this pattern fixes the problem of a high number of optional arguments and inconsistent state.
Pattern of the Factory Method
When you have a superclass that has Multiple subclasses and we require to get back one of the subclasses on the basis of input, you utilize the factory design pattern. With this approach, the factory class is in charge of creating instances of the Class, rather than the client application. Either the factory class or its associated factory method can be made static.
Prototype Pattern
When you already have an object that serves a comparable purpose and creating a new one would take too much time and effort, you may use the prototype pattern. This pattern thus offers a means through which we may make a duplicate of an Object and then make changes to that copy as required. The Object is copied using Java’s cloning mechanism in this design. According to the prototype design pattern, you must have the ability to replicate from the Object itself. No other group is permitted to do this action. Nevertheless, whether shallow or deep copy of the object’s characteristics is used is design decision that is dependent on needs.
Singleton Pattern
To guarantee that there is only ever one instance of a Class running in the Java Virtual Machine, the singleton design pattern places constraints on how often a Class can be instantiated. It has long been a point of contention among programmers whether or not to use the singleton paradigm.
3.2 Structural Patterns
Building bigger structures out of lighter, individual elements that are, in most cases, of distinct classes is facilitated by structural patterns. spotting a fundamental approach to spotting relationships between items, these patterns may also be utilized to simplify the design process. The primary goal of implementing structural patterns is to improve software performance without significantly altering the product’s original design.
Adapter Pattern
One of the structural design patterns, the adapter pattern facilitates communication across interfaces that otherwise wouldn’t be connected. An adapter is a device that connects two different interfaces.
Decorator Pattern
To dynamically alter an object’s behavior, the decorator design pattern is employed. This change in behavior is exclusive to the item in question and will not affect any other objects of the same kind. The decorator design pattern is a compositional design pattern that employs abstract classes or interfaces, like the adapter pattern, bridge pattern, or composite pattern. Dependency and compilation allow us to modify an object’s behavior at compile time so that it is consistent across all instances of the class. We need the decorator approach when we can’t modify an object’s behavior or add new features while it’s running.
Facade Pattern
A common design pattern, the Facade Pattern abstracts complexity away from its underlying implementation. It simplifies complex references to library or subsystem functions, making the code easier to read and navigate.
The technique may also be utilized to improve the API’s user experience significantly. The underlying subsystem can be controlled more easily.
Proxy Pattern
Proxy patterns allow one Object to act as a stand-in for and restrict access to another Object. When implementing permissioned access to features, we employ this technique.
Composite Pattern
When trying to illustrate a part-whole structure, the composite pattern is what we turn to. The composite design pattern is useful when we need to build a structure in which all of the constituent items must be handled in the same way.
Bridge
The bridge design pattern serves to separate the application’s interfaces from the implementation and keep the operational details from the client applications when there is a hierarchy of interfaces in both the interfaces and the implementations. The bridge pattern adheres to the principle of using composition rather than inheritance.
Flyweight
When we have a large number of Class Objects to develop, we turn to the flyweight design approach. By reusing Objects, the flyweight design pattern helps alleviate the strain on memory, which is especially important for low-memory devices (such mobile phones or embedded systems). The Java implementation of the string pool is a great illustration of the flyweight design pattern.
3.3 Behavioral Patterns
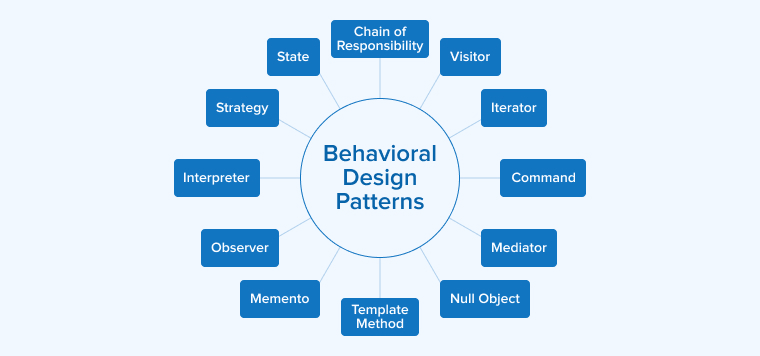
Behavioral design patterns are types of design patterns that characterize and name the ways in which various entities interact. Using behavioral design pattern helps transform complex software flowcharts into more manageable links between different classes and objects.
Chain of Responsibility
By passing a client request via a series of objects, the chain of responsibility pattern helps software designers attain loose-coupling. The object in the chain then determines whether or not the request must be passed onto the next object in the chain for processing.
The try-catch block of code allows for more than one catch statement. Each catch block acts here like a mini-processor, handling only the exception at hand. If an error is found in the try block, control will immediately pass to the first catch block, If the current catch block is unable to handle the request, it will pass it through to the following Object in the chain. If the exception is not handled by the last catch block, it is thrown back up the call stack to the original program that generated it.
Command
Loose coupling in a request-response architecture may be achieved with the help of the command pattern. Requests are communicated to the invoker, which then forwards them to the enclosed command object in the design pattern. To carry out the specified task, the command object communicates the request to the relevant receiver method.
Interpreter
The interpreter pattern specifies a means through which a language’s grammar may be represented and interpreted.
Iterator
The iterator pattern, another behavioral pattern, is employed to supply a regular means of moving through a collection of items. For iterating through a collection, developers often turn to Java’s Collection Framework, where the iterator interface offers convenient helper functions. This technique is also used to supply specialized iterators that meet the needs. Iterator methods are used by client applications, and the iterator design conceals the underlying implementation of Collection traversal.
Mediator
The mediator design pattern establishes a single point of contact for all system objects to use when coordinating with one another. Direct object interaction can increase maintenance costs and reduce the system’s scalability since it makes the individual components dependent on one another. The mediator pattern is concerned with creating loose-coupling across objects and facilitating communication between them. The mediator functions as a router, allowing for communication between objects using its own internal logic.
Memento
The memento design pattern is implemented whenever there is a need to record an object’s current state for subsequent restoration. Implementing it using this pattern ensures that the object’s recorded state data is secure and cannot be accessed from beyond the Object.
The Memento pattern requires two Objects: a creator and a keeper of the memento. The Object whose state has to be maintained and recovered serves as the originator, and its state is retained in an inner class. The “Memento” inner class is protected from being accessed by other objects since it is confidential.
Null Object
The goal of this pattern is to model the non-existence of an object with a null one.
Observer
When you care about keeping track of the current status of an Object and receiving updates anytime that status changes, an observer design pattern may be of assistance. The Object in the observer pattern is the one doing the monitoring, while the Object being monitored is referred to as the “subject.” The java.util.Observable class and the java.util.Observer interface offer an integrated framework in Java for using the observer pattern.
The lack of support for multiple assets in Java classes and the inconvenient nature of modifying a class just for incorporating the observer pattern means that this design pattern is not generally utilized. Applications may sign up to and post data to other applications using Java Message Service (JMS), which employs the observer pattern and the mediator pattern to do this.
State
The state design pattern is implemented when an Object’s behavior modifies in response to a shift in its internal state. A state parameter in the Object and an if-else condition block allow us to modify the Object’s behavior depending on the current state. The state pattern serves to execute state and context systematically and in a loosely connected manner.
Strategy
When numerous algorithms exist for a given job, and the client must choose which one to employ at runtime, we utilize the Strategy pattern. Policy is another name for a strategy pattern.
The Collections.sort() function, which accepts a Comparator as an argument, is a great illustration of this pattern. The objects get ordered in various ways due to the various applications of comparator interfaces.
Template Method
In order to generate method stub and defer part of the implementation stages to subclasses, template method pattern is employed. A default implementation that may be shared by all or some of the subclasses can be provided by the template method, which also specifies the steps necessary to carry out the algorithm.
Visitor
When working with several instances of the same type of object, the visitor pattern comes in handy. Using a visitor approach, we may decouple the objects’ functional logic from their own implementation.
4. Summary
Java comes with many advantages and disadvantages. One of them is, when software developers encounter recurring problems, they commonly turn to software design patterns for answers. Design patterns are not actual lines of code, but rather explanations of how to go about solving common development issues. To put it simply, design patterns are just well-documented solutions to common issues. They are not meant to be taken as suggestions on how to solve the software problem at hand, but rather as a framework against which solutions might be evaluated.
In this post, we took a cursory glance at several different types of design patterns. A design pattern is a recurring method that may be used to address a specific issue. Our main goal was to figure out when and where a certain pattern may be useful in practice.
This blog post provides a concise and informative introduction to Java Design Patterns. I found the breakdown of the three main types of design patterns (creational, structural, and behavioral) to be particularly helpful. Overall, this is a well-written and valuable resource for Java developers of all experience levels.