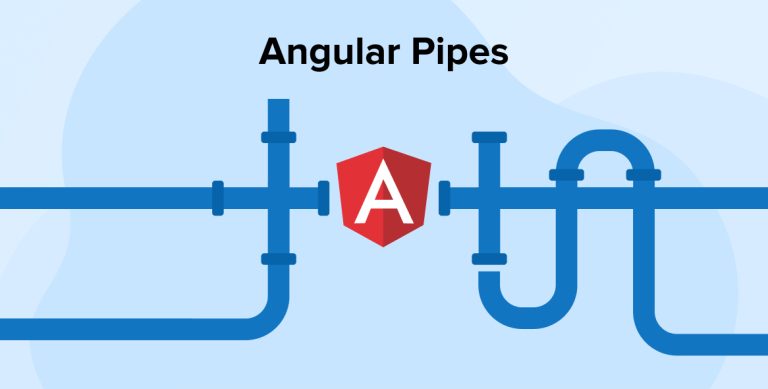
Angular is the most sought-after front-end development framework by business organizations, because it comes with many built-in methods & functionalities, such as Angular pipes. It allows companies to showcase their services and abilities in a very unique manner. Angular Pipes is an approach that enables developers to create apps where the users can change the format of the screen where the data is being displayed. For instance, the users can change the format of the date. To know more about this concept and see what exactly Angular Pipes has to offer, let’s go through this blog.
This is the reason firms choose Angular development companies for crafting their unique business software solutions.
1. What are Angular Pipes?
Angular Pipes is a concept that enables Angular developers to transform the output. Users use this approach to improve the data and present it in a more desirable format. Basically, Angular Pipes changes the data and does not alter it to make it appear well in front of the developers. Simple functions use these pipes to accept an input value from the user, process it, and return the output with a transformed value.
Angular comes with support for multiple pipes that are built-in. But it also enables the Angular developers to create their own custom pipes as per the business requirements.
#Angular Tip: Pipes
— Mini Bhati (@minibhati93) April 19, 2022
Pipes are used for transforming data for display.
Pipes are functions that return a transformed value of the input provided. It accepts parameters as well.
To apply a pipe, use the pipe (|) operator in the template. pic.twitter.com/cj4RA8xgYS
2. Built-in Pipes in Angular
Here are some of the widely used built-in pipes in Angular for typical data transformations –
2.1 JSON Pipe
JSON Pipe is used to transform a JavaScript object and convert it into a JSON string. Here is how it can be done –
import { Pipe, PipeTransform } from '@angular/core'; <h1>JsonPipe</h1> <!-- ngNonBindable deactivates interpolation, directives, and binding in templates. --> <p>{{ car }}</p> <p>{{ car }}</p> <p>{{ car | json }}</p> <p>{{ car | json }}</p> |
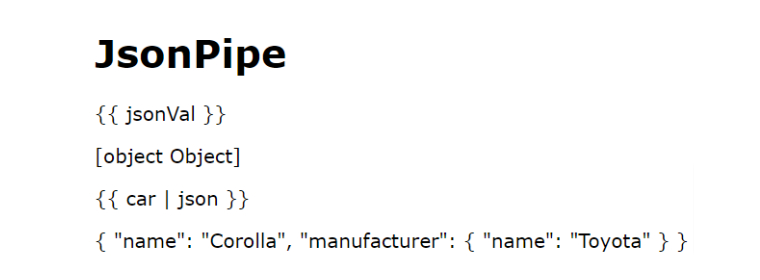
2.2 DatePipe
The use of DatePipe is to transform the date object in Angular applications. This transformation goes as shown below –
<h1>DatePipe</h1> <!-- ngNonBindable deactivates interpolation, directives, and binding in templates. --> <p>{{ dateValue | date : "shortTime" }}</p> <p>{{ dateValue | date : "shortTime" }}</p> <p>{{ dateValue | date : "fullDate" }}</p> <p>{{ dateValue | date : "fullDate" }}</p> <p>{{ dateValue | date : "d/M/y" }}</p> <p>{{ dateValue | date : "d/M/y" }}</p> |
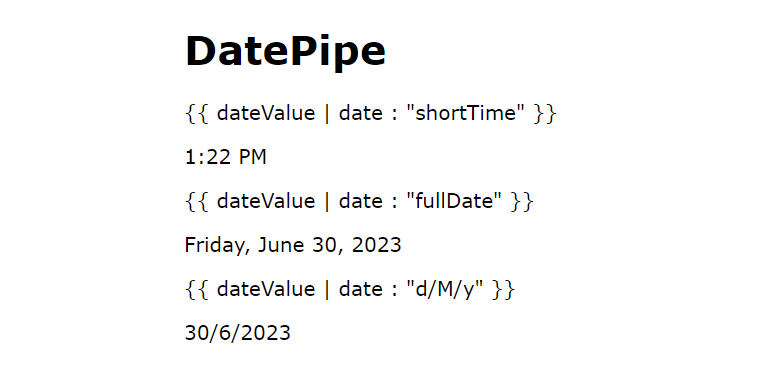
2.3 PercentagePipe
PercentagePipe comes with an approach that enables the developers to format a number as a percent. You can do it like this-
<h1>PercentPipe</h1> <!-- ngNonBindable deactivates interpolation, directives, and binding in templates. --> <p>{{ 0.123456 | percent }}</p> <p>{{ 0.123456 | percent }}</p> <p>{{ 0.123456 | percent : "2.1-2" }}</p> <p>{{ 0.123456 | percent : "2.1-2" }}</p> <p>{{ 42 | percent : "10.4-4" }}</p> <p>{{ 0.123456 | percent : "10.4-4" }}</p> |
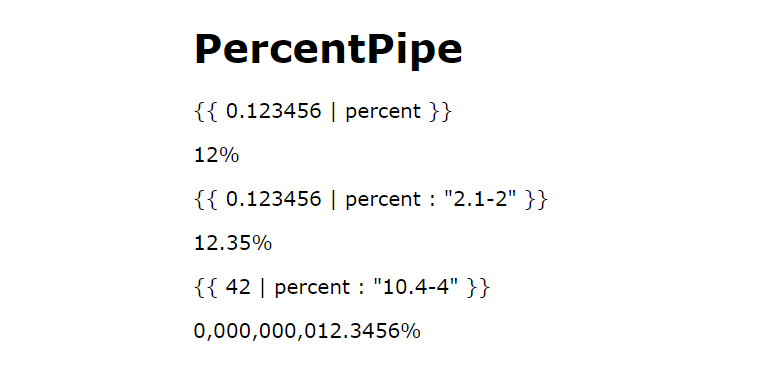
2.4 Lowercase & Uppercase
Lowercase is a built-in pipe that is used to transform a string to lowercase. Here’s how developers can do so –
<h1>LowerCasePipe</h1> <!-- ngNonBindable deactivates interpolation, directives, and binding in templates. --> <p>{{ "ANGULAR" | lowercase }}</p> <p>{{ "ANGULAR" | lowercase }}</p> |
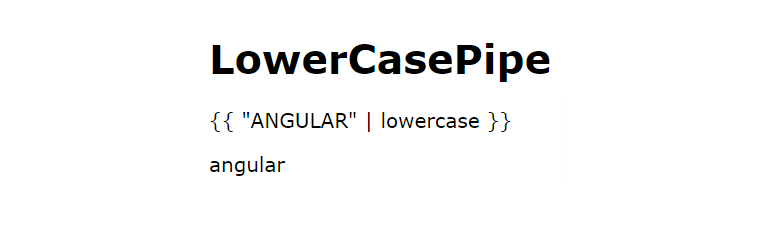
And when the developer needs to transform the string to uppercase, the following code must be followed –
<h1>UpperCasePipe</h1> <!-- ngNonBindable deactivates interpolation, directives, and binding in templates. --> <p>{{ "angular" | uppercase }}</p> <p>{{ "angular" | uppercase }}</p> |
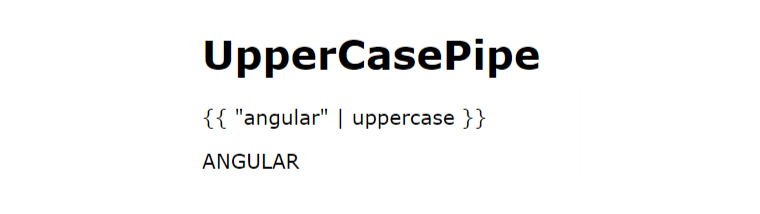
2.5 SlicePipe
SlicePipe is another built-in pipe of Angular that helps in returning a slice of an array. In this approach, the first argument is the slice’s start index of the slice and the second argument is its end. You can see this in the below code –
<h1>SlicePipe</h1> <p>{{ [1, 2, 3, 4, 5, 6] | slice : 1 : 3 }}</p> <p>{{ [1, 2, 3, 4, 5, 6] | slice : 1 : 3 }}</p> <p>{{ [1, 2, 3, 4, 5, 6] | slice : 2 }}</p> <p>{{ [1, 2, 3, 4, 5, 6] | slice : 2 }}</p> <p>{{ [1, 2, 3, 4, 5, 6] | slice : 2 : -1 }}</p> <p>{{ [1, 2, 3, 4, 5, 6] | slice : 2 : -1 }}</p> <pre> <ul> <li> {{ v }} </li> </ul> |
- {{ v }}
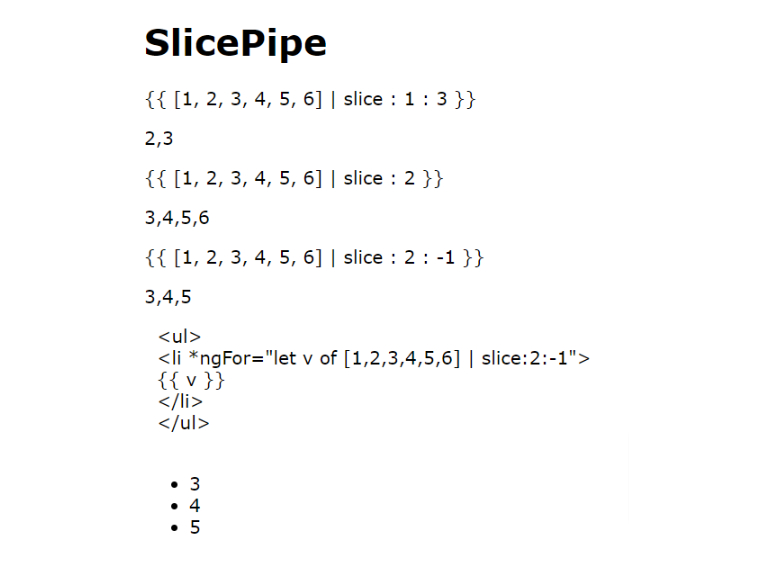
2.6 Async pipe
The last built-in pipe for Angular in our list is the Async Pipe which accepts a promise or an observable and enables the developers to render the output.
3. Custom Pipes in Angular
When the businesses have some specific transformations, the developers have to create custom pipes in Angular as the default approach won’t be enough. For this, the developers have to follow some steps that enable them to offer the best custom pipe services in Angular. And here we will go through these steps –
3.1 Creating a Custom Pipe
The very first step is to create a pipe. And here we will go through a simple example that will enable you to offer a string parameter that gives an output as Hello. Look at the code for it –
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'sayHi' }) export class CustomPipe implements PipeTransform { public transform(name: string): string { return 'Hi, ' + name; } } |
Here is the above code, you can see a name that is specified in the @Pipe decorator is a pipe’s name that will be utilized in HTML like uppercase, lowercase, and a date. When developers implement the transformation function, it performs like magic, enabling them to deliver the desired transformation to businesses according to their requirements.
3.2 Registering a Custom Pipe
The next step is to register the custom pipe that is created and in order to do so, the developers need to add a custom pipe class. And in the below code, we are going to create a module for the pipe that can be used for other modules if exported.
import { NgModule } from '@angular/core'; import { CustomPipe } from './custom.pipe'; @NgModule({ declarations: [ CustomPipe ] exports: [ CustomPipe ] }) export class CustomPipeModule { } |
3.3 Using a Custom Pipe
Now, it’s time to use the pipe after it’s created, registered, and the module has been imported. For this, suppose you have a user object with property firstName in our component which is actually a string that holds the first name of the user. If this is the case, you can add it to the HTML paragraph for another use, as shown in the below code –
this.user = { firstName: 'Jordan', ... }; -------------------------------------------------- <div> <p>{{ user.firstName | sayHi }}</p> </div> <!-- output <p>Hi, Jordan</p> --> |
3.4 Custom Pipes Examples
Now after having a look at the process that helps you create the custom pipe, let’s go through the examples of custom pipes that are being used in real-world applications.
Currency Pipe
As you know that Angular comes with a built-in CurrencyPipe which can be used in the applications when needed. But if for any application, developers need to have something customized, here is the code that will show you how to build one.
For instance, think you need this – 105.5 > R$ 105,50
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'currencyCustomFormat' })export class CurrencyCustomFormat implements PipeTransform { public transform( value: number, currencySign: string = 'R$ ', chunkDelimiter: string = '.', decimalDelimiter: string = ',' ): string { if (!value) return currencySign + '0' + decimalDelimiter + '00'; const changedValue = this.addCommas(value.toFixed(2)); const formatted = changedValue.toString().replace( /[,.]/g, function (value) { return value === ',' ? chunkDelimiter : decimalDelimiter; } ); return currencySign + formatted; } private addCommas(nStr) { nStr += ''; const a = nStr.split('.'); let a1 = a[0]; const a2 = a.length > 1 ? '.' + a[1] : ''; const rgx = /(\d+)(\d{3})/; while (rgx.test(a1)) { a1 = a1.replace(rgx, '$1' + ',' + '$2'); } return a1 + a2; } } |
As seen in this code, one can receive multiple inputs as required if the above code is used. Here, the first parameter is the value which is formatted itself and then we are receiving the chunkDelimiter (string), the currencySign (string), and the decimalDelimiter (string), which are useful in transforming the functions.
Here’s how the pipe can be used –
{{ 9999.9 | currencyCustomFormat : 'R$' : '.' : ',' }} <!-- output: R$ 9.999.90 --> |
Document Format Pipe
Another example of a custom pipe is a document format pipe. In some places, people have their documents named CPF, which is a unique number. And this number refers to the formatting of the document. Therefore, send and receive the output value of the document correctly with its value. For this document custom pipe is used.
For instance, if we want this – 11111111111 -> 111.111.111–11
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'cpfCustomFormat' }) export class CpfCustomFormat implements PipeTransform { public transform(cpf: string): string { if (!cpf || cpf.trim() === '') { return ''; } let formatted: string; formatted = cpf.substring(0, 3) + '.'; formatted = formatted + cpf.substring(3, 6) + '.'; formatted = formatted + cpf.substring(6, 9) + '-'; formatted = formatted + cpf.substring(9, 11); return formatted; } } |
Here as seen in the above code, the pipe is simpler as no other parameter is required to value itself. This is how the pipe looks at the end –
{{ '99999999999' | cpfCustomFormat }} <!-- output: 999.999.999-99 --> |
4. Types of Angular Pipes
Angular pipes are categorized into two major types: Pure and Impure pipes
4.1 Pure Pipes
The first type of Angular pipe is a pure pipe. It is a type of pipe where there is no use of any internal state. Besides this, even the output of the pipe remains the same if the parameters that are passed are the same. This pipe is called by Angular when it detects that the parameters have been changed.
4.2 Impure Pipes
Another type is an impure pipe. Angular calls this type of pipe for every little change that is detected in the code regardless of any change in the input fields. There are many different pipe instances created when it comes to the impure pipe. Here is an example that shows how one can specify if the pipe is impure with the use of pure property.
@Pipe({ name: 'democustompipe', pure : true/false }) export class DemoCustompipePipe implements PipeTransform { |
5. Conclusion
Angular pipes are a huge and essential concept that enables developers to transform the output of an application. Though they are very simple but are considered very impactful functionalities in the Angular world. As discussed in this blog, there are many different built-in Angular pipes but still, if the developer wants to offer a unique functionality to any application as per its requirements, he can create pipes in Angular and can transform the function of any application.
FAQs
What are pipes in Angular?
Ans: Pipes are essentially functions that we can add straight to any term or value in a structure to change its effect. You may modify the format of the data that is shown on the screen with Angular Pipes.
What are the 2 types of pipes in Angular?
Ans: There are two types of pipes in Angular:
1. Pure Pipes: When Angular notices an alteration in the value or arguments supplied to a pipe, only then is it called a pure pipe.
2. Impure Pipes: Regardless of the value or parameter(s) changes, an impure pipe is referred to for each variation detection cycle.
Comments
Leave a message...