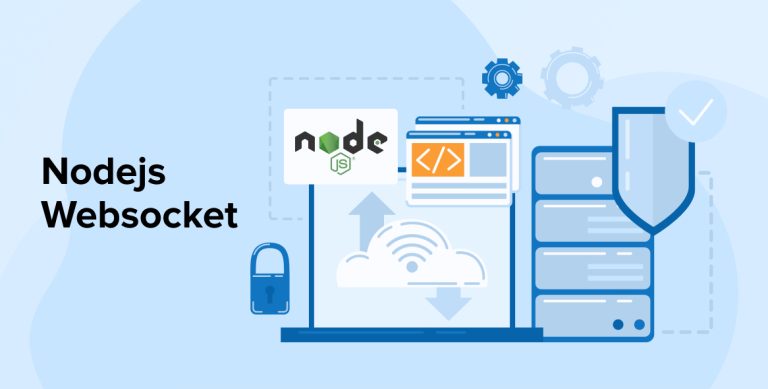
Generally, Web apps are developed in a way that backend and frontend are closely connected with each other so they can easily serve data to the user’s browser. However, nowadays, developers create applications that are loosely coupled which means that they have separate backends and frontends that are connected through a network-oriented communication line.
For instance, with HTTP protocol, the developer prefers to use the RESTful pattern in order to implement a communication line between both front end and back end of the system for transferring the data. But in this case, as the HTTP-based RESTful approach uses one-way communication, sending data directly to the client from the server is not possible without using workarounds like polling. One such perfect approach is the WebSocket protocol.
WebSocket helps developers to solve the drawback of the traditional HTTP pattern by providing a full-duplex (or two-way) communication approach. This is why this concept is used by the majority of Node.js development companies to create real-time apps. To know more about WebSocket, let’s go through this blog.
1. What is a WebSocket?
Node.js developers use the WebSocket library to create WebSocket servers for Node.js applications. This protocol offers real-time, continuous, and two-way communication between client and server through a single TCP socket connection. This means that one can listen to the incoming connection requests and can respond to them via raw messages as either byte or string buffers. Besides, when it comes to WebSockets, it is possible to work with it on the server and later on the browser’s Native WebSockets API on the connected clients.
Basically, Nodejs WebSocket is a computer communications protocol that defines the establishment of the API to build a persistent connection between the server and the web browser. Node.js app development companies use WebSocket to create real-time applications, displaying stock data, chat applications, and more.
2. What Types of Applications Use WebSockets?
In today’s time, developers use the WebSocket protocol wherever a solution requires real-time communication. Here are some of the use cases that prove this –
- Stock Price updates
- Chat Apps
- Live Audience Interaction
- Live Location Tracking on a Map
- Online Auctions
- IoT Device Updates
All the WebSocket examples we saw here create a TCP socket connection between multiple processes and devices. Similarly, the developers can perform a simple implementation process for the functionality of the application by using a hosted pub/hub service or HTTP Long Polling. To understand this concept in a better way, here we will go through a simple example where we will use WebSockets and Node.js.
3. Node.js WebSockets Code (Server)
Here, we will see how the developer writes the source code for the Nodejs WebSocket server to make it receive messages. In this application, we will require both a WebSocket and a web server (simple HTTP server). Here the web server allows the Node.js app developers to load the client that is running in a browser, and the WebSocket server that helps in handling the bidirectional chat messages.
In order to create the Node.js application, the developers will first have to install both the WebSocket packages and the Express.js that can help in offering the WebSocket server and web server respectively.
npm init |
[Follow the prompts and accept the defaults]
npm install --save express npm install --save ws |
Here, in this code the web server part will serve a single web page to the client, websocket-client.html, on port 5000:
const express = require('express') const webserver = express() .use((req, res) => res.sendFile('/websocket-client.html', { root: __dirname }) ) .listen(5000, () => console.log(`Listening on ${5000}`)) |
The developer can create a WebSocket server by just writing a few lines of code using the Node.js WebSocket library package:
const { WebSocketServer } = require('ws') const sockserver = new WebSocketServer({ port: 443 }) |
After the developers create an instance of a WebSocket server, they need to specify the port to run on i.e. 3000 or 5000. After this, we can define the action that will occur once we establish the WebSocket connection to build WebSocket communication. For this, here we have written a function connection event to the console and we will forward any function message received to the previously connected client. Typically, WebSockets operate on ports similar to traditional HTTP/HTTPS (i.e., port numbers 80 and 443). Have a look at the following code to get a clear idea about it.
sockserver.on('connection', ws => { console.log('New client is connected!') ws.send('connection is established') ws.on('close', () => console.log('Client disconnected!')) ws.on('message', data => { sockserver.clients.forEach(client => { console.log(`distributing message: ${data}`) client.send(`${data}`) }) }) ws.onerror = function () { console.log('error in websocket') } }) |
The system completes the server implementation part after running the above code. This means that now, each time a WebSocket message is received on any WebSocket server, the server will proxy the entire message to all the clients connected to the socket. This is how any basic group-chat application works. Here is the full “index.js” code for the same, have a look at it.
const express = require('express') const webserver = express() .use((req, res) => res.sendFile('/websocket-client.html', { root: __dirname }) ) .listen(5000, () => console.log(`Listening on ${5000}`)) const { WebSocketServer } = require('ws') const sockserver = new WebSocketServer({ port: 443 }) sockserver.on('connection', ws => { console.log('New client is connected!') ws.send('connection is established') ws.on('close', () => console.log('Client disconnected!')) ws.on('message', data => { sockserver.clients.forEach(client => { console.log(`distributing message: ${data}`) client.send(`${data}`) }) }) ws.onerror = function () { console.log('error in websocket') } }) |
The developers can run the above code from the command line as given below to efficiently perform operations –
node index.js |
The code we saw above brings all the messages that are either in a text format or JSON encoded (JSON.stringify). The reason behind it is that by default this code uses the utf-8 charset.
4. WebSockets Code (Client / Browser)
Now, after understanding the WebSocket coding on the server side, we will learn about the WebSocket coding on the browser or client side. Here, a developer doesn’t have to install additional packages or software applications to use WebSockets with modern web browsers. All they need to do is create a listening WebSocket by specifying a correctly formatted URI, as shown in the code below.
const webSocket = new WebSocket('ws://localhost:443/'); |
After this, you must define an event handler to manage all the messages received from the server.
webSocket.onmessage = (event) => { console.log(event) document.getElementById('messages').innerHTML += 'Message from server: ' + event.data + "<br>"; }; |
When the above code is executed, the messages will start coming to the browser from the WebSocket server.
If someone wants to send a message back using a message event from the client side, they use a socket object and the send() method to establish a connection with the server-sent events.
webSocket.send('hello world') |
The thoroughly tested WebSocket client code will allow the browser to pass messages smoothly and after the messages start going from the client side to the server side, in order to make the chat app functional, the developers need to add an input field and a ‘send message’ button on the browser side. You must write the frontend HTML code as soon as possible in the following HTML file.
<label for="message">Enter Message:</label> <input type="text" id="message" name="message"><br><br> <input type="submit" value="Send"> </form> <div id="messages"></div> <script> const webSocket = new WebSocket('ws://localhost:443/'); webSocket.onmessage = (event) => { console.log(event) document.getElementById('messages').innerHTML += 'Message from server: ' + event.data + "<br>"; }; webSocket.addEventListener("open", () => { console.log("Connection Established"); }); function sendMessage(event) { var inputMessage = document.getElementById('message') webSocket.send(inputMessage.value) inputMessage.value = "" event.preventDefault(); } document.getElementById('input-form').addEventListener('submit', sendMessage); |
While writing the above code, the developers must make sure to name the HTML page as “websocket-client.html” and launch a few tabs in the web browser to see that they are being navigated to HTTP protocol – http://localhost:5000. After this is done, one can see all the received messages in every tab as shown in the below image.
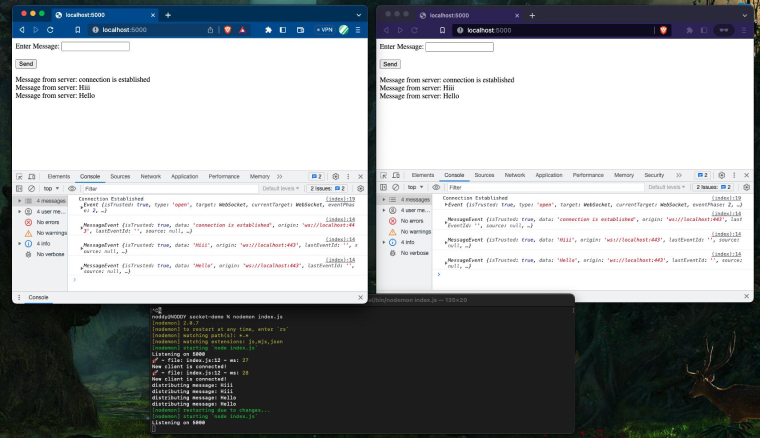
5. Conclusion
As seen in this blog, WebSockets are an interesting approach to creating real-time web applications. They offer flexibility to developers to leverage two-way communication for their applications. . The WS library efficiently builds WebSocket connections between the client and the server side, which eventually makes data transfer an easy process.
6. FAQ
Is node JS good for WebSockets?
NodeJS is a technology that has the capability to simultaneously maintain various WebSockets connections. Unlike any other JavaScript technologies, NodeJS doesn’t have a WebSocket solution to manage fallbacks, upgrades, and settings to create and maintain a WebSocket connection. Overall, NodeJS is good for WebSockets as it offers a scalable and fast environment.
How to use WebSocket with NodeJS?
To use WebSocket in NodeJS, the developers need to first install a dependency known as socket.io. To install it, run the command npm install socket.io –save in the cmd, and then add this dependency to the server-side JavaScript file. Additionally, the developer must install the express module by running the command npm install express –save in the cmd, which is necessary for server-side applications.
What is the difference between NodeJS WebSocket and socket io?
WebSocket is a technology that helps NodeJS developers take advantage of two-way real-time communication between the server and the client. On the other hand, developers know Socket.IO as a library that provides an abstraction layer on top of WebSocket, ultimately enhancing the creation of real-time applications.
Is WebSocket better than rest?
The WebSocket is an ideal method for creating real-time and scalable applications and on the other hand, REST is well-suited when an application gets a lot of requests.
Comments
Leave a message...