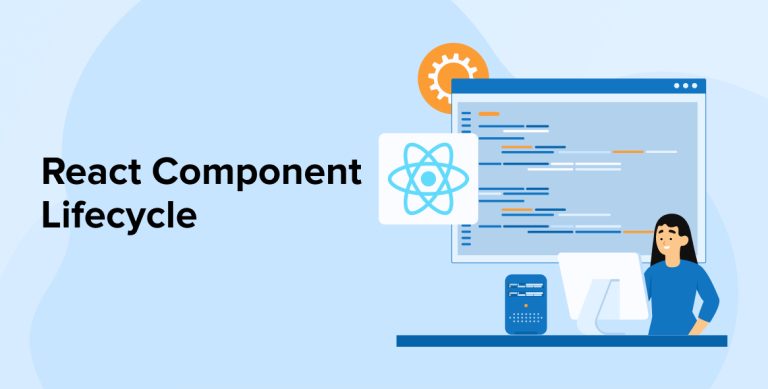
React components are being used to isolate or split different web user interfaces into separate parts by React development companies. These different parts become independent and start using render functions to return elements in JSX. The React elements returned after rendering describe how a particular section of the React application should be displayed to the user. These different parts are known as components and the React component lifecycle has different phases like mounting, updating, and unmounting in React development.
In this blog, we will learn everything about React components and their lifecycle methods each React component lifecycle phase has.
1. Phases of React Component Lifecycle
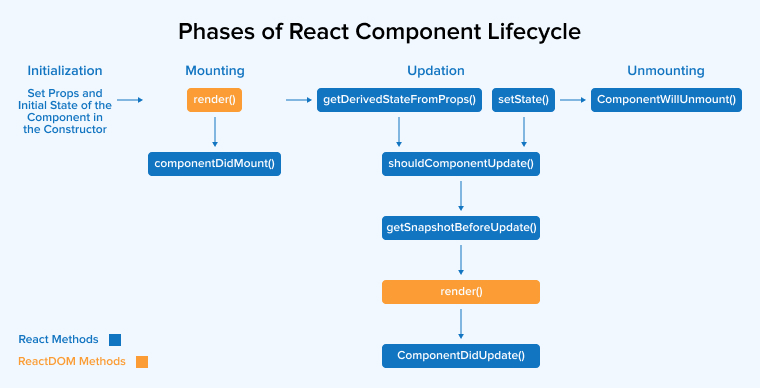
In ReactJS, when it comes to creating components, there is a specific process that the developers need to follow as it comes with different lifecycle methods for every component. These different React lifecycle methods are called the lifecycle for each React component. Generally, the component’s lifecycle method is not complicated which means that developers can call it anytime in the project during a component’s life. To understand it clearly, one must know that the React component lifecycle comes with four different phases known as the Initial Phase, the Mounting Phase, the Unmounting Phase, and the Updating Phase.
Here, we will go through each of the phases one by one and know how it works.
1.1 Initial Phase
The first phase of the component lifecycle method is called the initial phase or the birth phase of the entire cycle. This is the component state that starts its journey towards the DOM. In this phase, the React component has the default Props and the initial state that is required for developing an application. This initial phase only occurs once in the project when it has only the required method. Here are the methods that are a must for the occurrence of this initial phase –
- getInitialState(): This is a method that can be used by the React app development companies to specify the default value of this.state. It is an initial phase method that can be invoked before the React component gets created.
- getDefaultProps(): This is a method that is used by the developers to define the default value of this.props. It can be invoked before creating the props or components.
1.2 Mounting Phase
The second phase of the React component’s lifecycle method is called mounting. It is a phase where the component instance is created and inserted into the DOM by following specific methods. And those methods are –
- componentDidMount(): It is a method that can be invoked as soon as the component gets rendered and inserted into the DOM. With this method in hand, the developers can carry out any DOM querying operations.
- componentWillMount(): It is a method that can be invoked before the component rendering process in the DOM starts. With this method, the component will not get re-rendered when the setState() is called inside.
- render(): This is a method that is defined by the React app developers in every component. render() method returns a single root HTML node element and in this case, if there is nothing to render, the value will be returned as a null or false.
1.3 Updating Phase
After mounting, the next step is to go through the updating phase in this lifecycle. In this phase, developers can change the state of the React component and get new Props. It also enables them to handle user interaction between the components as it offers a better communication hierarchy. Besides this, the main goal of the updating phase is to make sure that the React component can display the latest version of itself. It is different from the Birth or Death phase. In addition to this, it can be repeated again and again in a project. Some of the most important lifecycle methods used in the updating phase are –
- shouldComponentUpdate(): This is a method that can be invoked by the React app developers when a project needs to change the component or update it into the DOM. It allows one to control the behavior of the component and if the component gets updated successfully, it will return true as a value.
- componentWillRecieveProps(): In this method, the developers invoke when any component receives new props. In order to update the state of the component as a response to the changes that the prop has made, the developer needs to compare nextProps and this.props for performing the transition.
- componentWillUpdate(): This method can be invoked just before the component updating occurs. Here, the developers cannot just change the state of the React component by invoking this.setState() method. Besides, this method won’t be called if shouldComponentUpdate() returns the value as false.
- componentDidUpdate(): This lifecycle method is immediately invoked once the update occurs in the component. Any code that needs to be executed after the update offices can be put inside this method.
- render(): It can be invoked by the developers when it is time to examine this.state and this.props in order to return types like Arrays & Fragments, React Elements, String & Number, and Booleans or Null.
1.4 Unmounting Phase
This is the last and final phase and it can be called when the instance of a component is unmounted or destroyed from the DOM. It comes with the following method –
- componentWillUnmount(): It is a lifecycle method that developers invoke before the component is unmounted or destroyed permanently. With the help of this method, one can perform required cleanup tasks like canceling network requests, invalidating timers, and cleaning up DOM elements.
Now after learning briefly about different phases of the component lifecycle and their methods, let’s dive into each of these phases in detail and see what each React component phase has to offer.
2. Component Mounting Phase
Generally, a component mounts itself at the initial state when it is developed and inserted into the DOM for the first time. This first-time insertion is when a component is rendered for the first time. This component mounting phase comes with the following methods –
- constructor()
- render()
- componentWillMount()
- componentDidMount()
In the component mounting phase, constructor method and render method are essential as they are a part of the basic concepts of React. On the other hand, the componentWillMount method is an approach that is called right before a component mounts or is rendered. But this method is hardly used by the developers in creating React applications as this method sits between the render method and the which makes it difficult to use. The main reason behind this is that as the componentWillMount method is before the render method, developers can use it to set the default configuration for a component, but actually this is done better with the constructor method. But having said that, between these two lifecycle methods nothing changes which means that there is no requirement of setting the configuration again.
There is one way to make this method perform its best at any setup during runtime and that is by connecting the method with external APIs like Firebase. But when the developers decide to make this setup possible for their applications one thing needs to be taken care of is that in this situation the highest level of the React component such as the root component of the system should be used as it will make other components not use the method.
Now after understanding the component mounting phase, we will go through a simple component class example that will enable you to see how this method can actually be called before rendering the component.
class Example extends React.Component { componentWillMount() { console.log('Hello World!'); } render() { return <h1>Hello world!</h1>; } } |
2.1 The componentDidMount() Method
Now, let’s go through another method of the component mounting phase known as the componentDidMount lifecycle method. It is a method available in the system after the mounting of the component. This is possible once the HTML from the render method has completed its loading. This method is called only once in the component life cycle. The goal of this method is to give signals to the component and see if all the sub-components have been rendered properly.
In this method, the developers can easily call the API as the components that are mounted here are available to the DOM. Besides this, in the componentDidMount method, developers can easily set up that cannot be done without the DOM. In addition to this, componentDidMount is a method that enables the developers to connect their React application to external apps, add event listeners in the app, set timers, and do many more things.
Here is the example that tells the developers how to use the componentDidMount method to make API calls –
class Example extends React.Component { componentDidMount() { fetch(url).then(results => { // Some operations with the result }) } } |
3. Component Updating Phase
The next phase of the component lifecycle is the component updating phase. After mounting is carried; it is not necessary that the components will stay in the same state. Sometimes they have the tendency to change the underlying props and this means that the components have to be re-rendered. But when the updating phase is going on, the developer gets the freedom to control choosing when and how to update the phase. For this, there are five different updating methods for the component lifecycle and these methods must be called in a specific order to make it work. Here is the list of those lifecycle methods in the order they are supposed to be called –
- componentWillReceiveProps
- shouldComponentUpdate
- componentWillUpdate
- render
- componentDidUpdate
Let’s discuss the first three methods which are a bit critical and every developer might not be aware of their meaning and usage unlike the last two component lifecycle methods mentioned above.
3.1 The componentWillReceiveProps() Method
Generally, Props pass externally into one component by its parent component. While doing so, sometimes it happens that the props get hooked in the parent component’s state. This means that if the state of the parent component changes, the props that are passed to any other component will reflect that change. Therefore, the componentWillReceiveProps method is used by the developers before a component carries out changes with the new props.
The componentWillReceiveProps method is called with the new props and it can be passed as an argument. With the help of this method, the developers can easily access the next set of props along with the present ones. This is why this method is very useful. Here we will see an example of this method –
class Example extends React.Component { constructor(props) { super(props); this.state = {number: this.props.number}; } componentWillReceiveProps(nextProps) { if (this.props.number !== nextProps.number) { this.setState({number: nextProps.number}); } } render() { return ( <h1>{this.state.number}</h1> ) } } |
3.2 The shouldComponentUpdate() Method
In the React component updating phase, another method is the shouldComponentUpdate method, which developers can call in code before the component begins to re-render following updates to a new set or new props. This method will receive two arguments, the next state and the next props. This behavior of the component helps it to start the re-rendering process after the observable change in the stare of props. Besides this, React app development companies use this method to enable React to know that the component in a particular application has an output that is not affected by any kind of change in the state or props.
This method tends to return a true or false value. If it returns true, it means that the component will go ahead and carry out the re-rendering process. If it returns false then there will be no update in the component. The best result amongst these two is false for any ideal scenario. Here is an example showing that a component only changes if it gets a new input that is different than before.
class Example extends React.Component { shouldComponentUpdate(nextProps, nextState) { if (this.state.input == nextState.input) { return false; } } } |
A different way to use this method is when you need to change the props. Here, shouldComponentUpdate can be used instead of componentWillReceiveProps as it calls the component only when it actually is re-rendered. Similarly, if you need to change the state of the component, componentWillReceiveProps must be used. Here is an example of using the shouldComponentUpdate method before the component is re-rendered to perform animations or any other effects.
class Example extends React.Component { componentWillUpdate(nextProps, nextState) { // Some operations here } } |
3.3 The componentDidUpdate() Method
The last method of the component updating phase is the componentDidUpdate method. This method is called after any rendered HTML completes its loading process. It receives two arguments, the component state before the updating period begins and the props. This is the best method to perform an interaction with the browser. It must be carried out until the developer wants to compare the current props to the previous ones. Here is an example of the same –
class Example extends React.Component { componentDidUpdate(prevProps, prevState) { if (this.props.input == prevProps.input) { // Ajax call // Perform any function } } } |
4. Component Unmounting Phase
The last phase is a component unmounting phase. Here in this phase, components do not always tend to be present in the DOM. The components might be removed from the DOM because of any changes in the state. Below we will discuss the method that you can use for unmounting the component.
4.1 The componentWillUnmount() Method
In the unmounting phase, componentWillUnmount is the only method and this can be called before removing the component from the DOM. It helps to perform cleanups like canceling network requests, invalidating timers, canceling any subscription, or removing event listeners made in componentDidMount. Here is the code for the same.
class Example extends React.Component { componentWillUnmount() { document.removeEventListener("click", SomeFunction); } } |
5. Wrap up
As seen in this blog, components in React app development are quite fascinating as on the surface they seem like not doing much of a change in the system but when a developer deeps down into knowing the component’s process and watches its changes, he can find out that React components make some huge changes in the system and they are very essential. There are different types of methods in the React component lifecycle as described in this blog and all these lifecycle methods have some small or major part to play in the smooth working of the entire React app development project.
Comments
Leave a message...