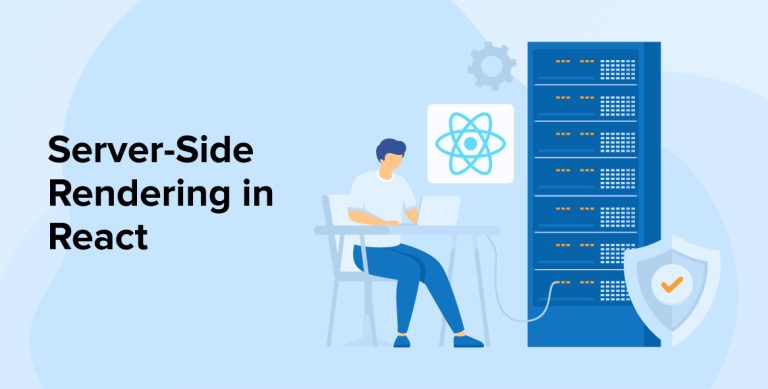
Server-side rendering is a powerful approach that can be followed by React app development companies for developing robust and dynamic web applications. This approach enables the developers to create applications that are not only interactive but also performant while relying on client-side execution. With the use of server-side rendered components, developers can create a codebase that can use technologies like Node and deliver content from the backend directly to the front end. This approach offers higher initial page load speed and features like state management.
In this blog, we will understand what is server-side rendering, the benefits of server-side rendering in React, and also learn how to implement server-side rendering in React applications using Next.js.
1. What is Server-Side Rendering (SSR)?
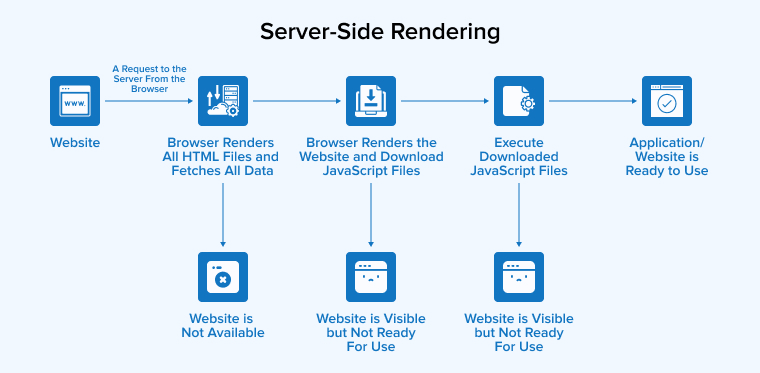
SSR is a simple process that developers use to render the content on the webpage on the server and not on the browser itself by using JavaScript. For instance, when any website is created using PHP, the content of the page is loaded via HTTP which was originally rendered on the server and displayed on the screen for the users. But when you create a React app, the process involves sending a .js file to the client. The browser’s JavaScript engine then generates the markup upon loading the .js file. This method allows for dynamic content rendering but relies on the client side for the initial page construction.
2. Advantages of Server-Side Rendering in React
From providing enhanced user experience to giving you control over how your content should appear on SERPs, there are many benefits of using SSR in React. A few of them include:
2.1 Improved Performance and User Satisfaction
Many developers prefer server-side rendering for the sole purpose of improving the performance. For the initial load, SSR does not require any spinners or loaders. And that’s enough for SSR to triumph over CSR in terms of performance.
The CSS and JavaScript code in React are broken down into small parts. They are later optimized and the server renders the whole pages before delivering them to the user’s browser. This significantly improves the initial loading time.
Quick load time is an important factor when it comes to user experience or user satisfaction rates. It is the reason why SSR in React is utilized in many large-scale and complex projects. You can get your Universal SSR application to work like a typical SPA after that initial load. It also enables smooth transitions between routes and web pages. SSR never re-renders the HTML content holders, it just has to send the data to and fro.
2.2 Distribution Of Content
Applications whose pages and content are rendered on the server side can be easily shared on social media platforms. When you share a link to your SSR app in a blog, it automatically prompts an image and title for it. Moreover, the social network snippets with rendered content are very helpful in driving more traffic to your app.
2.3 Accurate Page Loading Metrics
SSR is very well adept at gathering accurate metrics in real-time. This allows you to maintain as well as optimize your website. Unlike CSR, SSR provides detailed data like how your user is navigating from one page to another. Such data on user interaction is very valuable for offering enhanced UX/UI.
2.4 Search Engine Friendliness
Neither users like the slow load times, nor do the Google bots. These bots are tasked with indexing the web pages quickly. They can index static HTML pages quickly but not JavaScript. Just like a user has to download and then display the web content, bots ought to do the same.
However, they are allotted a fixed time to spend on a single page. So, your web page or website may remain unindexed and hence unranked in search engines if it fails to load on time.
SSR provides all the necessary elements for SEO in its initial response. Therefore, the web pages rendered on the server side will load quickly and hence, easily indexed. A website using React server-side rendering has a greater chance of ranking higher in search engine results.
3. Implementing SSR in React Using Next.JS
Now, after understanding what is server-side rendering (SSR) and going through its advantages, let’s dive into how to implement SSR using Next.js for an online bookstore application. With this approach, your online bookstore can provide a highly performant and user-friendly experience, enhancing your presence in the market.
Step 1: Create a New Next.js Project
The very first step is to create a new Next.js project. For this, the developer will have to run the following commands in the terminal –
npx create-next-app my-bookstore-app cd my-bookstore-app |
Step 2: Add Required Dependencies
After that, the React developer will have to add dependencies to the new project which can be done by using the following command –
npm install react react-dom next |
After the command has run to add the dependencies, the package.json might look like this.
{ "name": "my-bookstore-app ", "version": "0.1.0", "private": true, "scripts": { "dev": "next dev", "build": "next build", "start": "next start", "lint": "next lint" }, "dependencies": { "@next/font": "13.1.6", "eslint": "8.34.0", "eslint-config-next": "13.1.6", "next": "13.1.6", "react": "18.2.0", "react-dom": "18.2.0" } } |
The above result shows the version of the packages which might differ in your case.
Step 3: Set up the Environment Configuration
After adding the dependencies, it’s time to set up the configuration environment. For this, the developer will have to define an environment variable that will be stored in a .env.local file. This file holds all the required information about the configuration settings that can be later used by the React developers across various environments like production, staging, and development.
To set a configuration environment variable in the project, the developer will have first to create a file named ‘.env.local’ in the root of the project directory and define variables like ‘API_URL’ for API endpoints.-
API_URL=http://localhost:3000 |
After doing so, it is not advisable to check this file into source control. The reason behind this is that this file might contain sensitive information like API keys or database credentials. Instead of checking the file, the developer should create a template file named .env.example that can be used to store placeholder values for the configuration environment variables.
Step 4: Create a New Page
The next step is to create a new page for the project. While working with Next.js, the developers must keep one thing in mind: this framework uses a file-based routing system and this means that all the files present in the page directory will specify each page of the application.
Now, in order to create a new page, all one has to do is create a new file in the page’s directory. For instance, a developer can create a file named pages/books/index.js.
When this file is created, the developer can specify React components in it that will be rendered when the website user visits the same book path. Here is an example of the code that goes behind it –
function BooksPage() { const [books, setBooks] = useState([]) useEffect(() => { async function fetchBooks() { const res = await fetch('/api/books) const books = await res.json() setBooks(books) } fetchBooks() }, []) return ( <div> <h1>Books</h1> <ul> {books.map((book) => ( <li key={book.id}>{book.title} by {book.author}</li> ))} </ul> </div> ) } export default BooksPage |
Step 5: Create an API Endpoint
The next step is to create an API endpoint. This can be used to fetch the details or list of all the products available on the website. Next.js is a framework that enables developers to use a built-in API routing system that can help in creating serverless API endpoints for the site. In order to create an API endpoint, the developers need to first create a new file in the pages/api directory. For instance, one can create a file named pages/api/products.js. In this file, the developer will have to specify the function that can be used to execute the API endpoint for the users’ requests. Here is the code for the same –
const books = [ { id: 1, title: 'Book 1', author: 'Author 1' }, { id: 2, title: 'Book 2', author: 'Author 2' }, { id: 3, title: 'Book 3', author: 'Author 3' }, ]; export default function handler(req, res) { res.status(200).json(books ) } |
Step 6: Update the Page to Use Server-Side Rendering
Now, once the endpoint API is created, Next.js will use client-side rendering (CSR) as a default option to render pages. But as we want to use the server-side rendering (SSR) approach, the developer will have to switch to it and for that, the developer will have to update the page component so that it uses a getServerSideProps function. This is a special function that can be used by React developers to render the pages on the server side. To update the page and make it use a server-side rendering process, the following code can be used –
import { useState } from 'react' function BooksPage({ books}) { const [loading, setLoading] = useState(false) return ( <div> <h1>Books</h1> <ul> {books.map((book) => ( <li key={book.id}>{book.title} by {book.author}</li> ))} </ul> </div> ) } export async function getServerSideProps() { const res = await fetch(`${process.env.API_URL}/api/books`) const books= await res.json() return { props: { books} } } export default BooksPage |
Step 7: Start the Development Server
The next step is to start the development of the server and for this, the following command should be run in the terminal –
npm run dev |
The above command will help the developer to start the local server development at http://localhost:3000.
Step 8: Test the Application
The last step is to test the React application by visiting the /books URL path in the browser. If all the books are displayed as requests from the client side, this means that your server-side rendering in the Next.js application is successful.
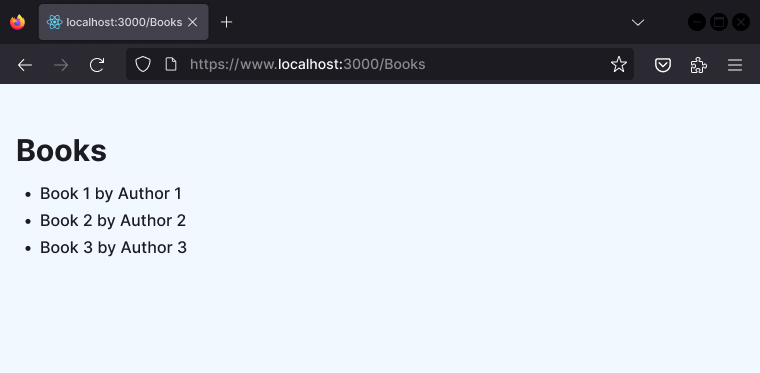
4. Concluding Words
As seen in this blog, server-side rendering is an essential approach and when it is carried out with React.js, developers can offer a robust application with improved user experience. This is why all React app developers must have a proper understanding of the above React server-side rendering approach and implementation of server-side rendering with frameworks like Next.js.
FAQs:
What are the Benefits of Server-Side Rendering in React?
Server-side rendering in React offers benefits such as better user experience, faster page loading, improved performance, SEO optimization, and accessibility for SPAs.
Is SSR Faster than CSR?
Yes, SSR is faster than CSR. In CSR, you have to download and execute JavaScript before rendering the pages which slows down the loading time. Meanwhile, the clients receive fully rendered HTML pages from the server in SSR, resulting in faster load times.
How Does React Server-Side Rendering Work?
In React Server side rendering, servers generate the HTML file and send it to the browser so that it can display the requested content in real-time.
Why Server-Side Rendering?
Server-side rendering is beneficial for websites like eCommerce stores, news sites, and blogs that host large quantities of content. Successful implementation of server-side rendering makes your UI responsive and loads the pages faster on the client side.
Comments
Leave a message...