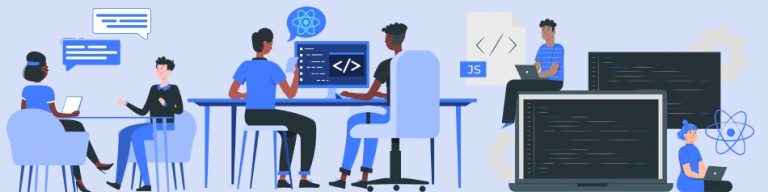
React is a very popular open-source JavaScript library that enables web app developers to create fast, simple, and scalable solutions for clients. It is the most flexible and efficient Javascript library in the IT market. Facebook’s expert software engineer, Jordan Walke created it. Initially, Facebook used React for its news feed section but with time Instagram also started using React, and now every small or big company uses or wants to use React for their applications.
React is an easy-to-understand and learn Javascript library especially for developers from a Javascript background. It comes with various features and functionalities that help developers to create React applications with ease. But for newbies, understanding all the concepts of React and knowing answers to React js questions is one of the best practices of React js and its security before joining any web development firm and starting to create applications.
For that, here in this blog, we have listed some of the top React interview questions that will help developers crack the interview and give them basic React knowledge for a lifetime.
1. List of Top React Interview Questions and Answers
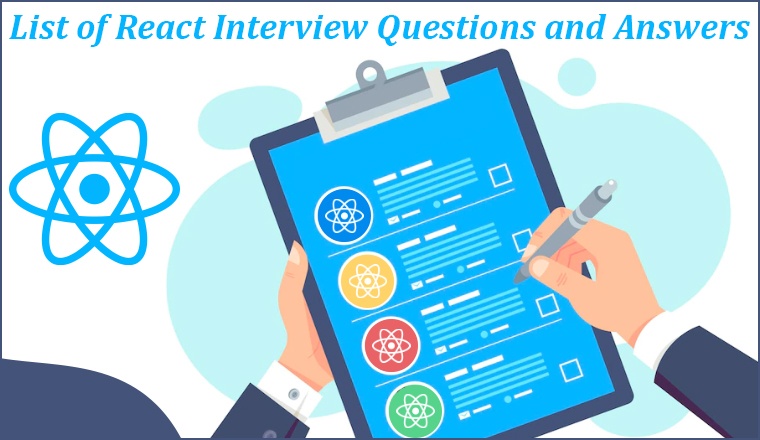
1.1 What is React?
React is one of the most popular JavaScript libraries available in the market. It is known as an effective, efficient, declarative, flexible, and open-source front-end library which was created by one of the top tech giants, Facebook in 2011. This front-end library follows the component-based approach to creating reusable user interface components for robust single-page applications (SPAs).
Basically, React is a technology that is used by developers to create interactive view layers of both mobile and web development.
1.2 What are the Key Features of React?
Some of the important features of React are –
- React is a library that follows a unidirectional data flow/ binding approach to creating interactive UIs.
- It supports server-side rendering.
- With React, the developers can use reusable UI components for creating the view of the application.
- React enables the developers to use the virtual DOM (Data Object Model) and not the real DOM. And the reason behind it is that RealDOM manipulations are very expensive compared to virtual DOM representation.
- There are many extensions available in React which can be used to create full-fledged UI applications. React is extended with React Native, Flux, Redux, etc.
1.3 What are the Advantages of Using React?
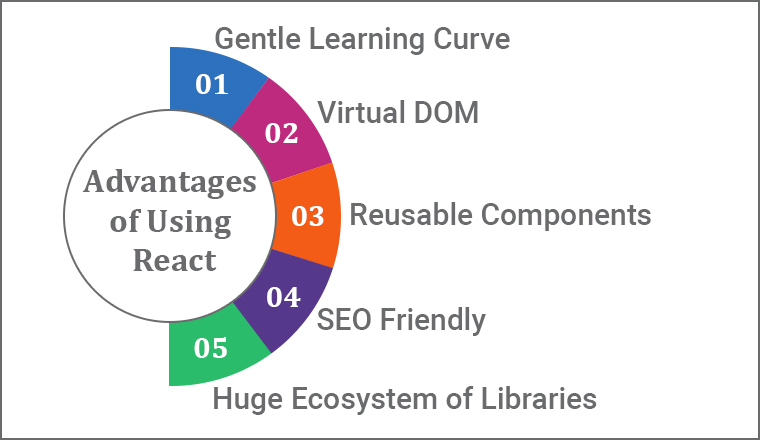
Some of the major advantages of React are –
- Gentle learning curve: When it comes to understanding React, any developer would feel that the learning curve of the React library is very gentle compared to other libraries or frameworks like Angular. React can be easily understood by any developer with some basic knowledge of JavaScript and HTML.
- Improve App’s Efficiency with Virtual DOM: React library enables web development experts to use virtual DOM to render the view of React applications. Virtual DOM is nothing but a representation of the real DOM elements and every time the data in the React app changes, a new virtual DOM is developed. And the creation of a virtual DOM is much faster & smoother than UI rendering inside the browser. This is why with the use of virtual DOM developers can improve the performance and efficiency of the React app.
- Reusable components: React, the JavaScript-based library uses component-based architecture. These React components are reusable components and independent bits of code. These parent components and child components of React can be shared across various JavaScript-based applications that have the same kind of functionalities and lifecycle methods. When the developers re-use pure components, it increases the development pace.
- SEO-friendly: React is known as one of the best libraries when it comes to creating SEO-friendly apps. This JavaScript library enables the developers to create engaging user interface/s that can easily grab users’ attention and navigate them through the app. It also can be easily read by various search engines. React also allows server-side rendering, which eventually helps in boosting the SEO of an application.
- Huge ecosystem of libraries: React front-end library comes with various libraries and tools from which the developers can choose any one of their choices. Besides, React’s compositional nature enables the developers to have an easy-to-use architecture for developing an application as per the client’s needs.
Further Reading On:
Top Angular Interview Questions and Answers
1.4 What are the Components in React?
In React, a component is known as a core building block. This means that every application created using React is made up of small pieces called components. These components enable the developers to create UIs easily and these UIs can be broken down into multiple pieces known as child components. And when they are all merged together into one component, it is known as a parent component. There are two main types of React components-
Functional Components: Functional components are the simple functions of JavaScript. React developers can create a functional component with the use of JavaScript functions and these functions might receive data as parameters.
Example:
class Cat extends React.Component { render() { return <h2>Hi, I am a Cat!</h2>; } } |
Class Components: Another type of component in React is the class component. Here the components are more complex. Besides, unlike functional components, the class components can work easily with each other and make the applications better. We can pass data from one class component to another class component. And here, JavaScript ES6 classes can be used to create class-based components.
Example:
class Cat extends React.Component { render() { return <h2>Hi, I am a Cat!</h2>; } } |
1.5 What are the Differences Between Class and Functional Components?
Class and functional are the two main types of React components. And they differ from each other when it comes to their working style and capacity. Let’s have a look at the difference between these two –
Class Components | Functional Components | |
State | Class components can manage state. | Functional components cannot manage state. |
Reusability | The components here are reusable. | The components are non-reusable. |
Lifecycle methods | Class components can work with different types of lifecycle methods. | Functional components cannot work with lifecycle methods. |
Simplicity | Class components are complex compared to functional components. | Functional components are easy & simple to understand. |
1.6 What is the main use of render() in React.js?
In React, render() is used to render the HTML to the web page and besides this, the render function can be mainly used for the following reasons in React –
- The purpose of the render function is to display a certain HTML code inside a certain HTML element.
- In the render() method, the state cannot be changed or any side effect cannot be caused.
- In the render() method, the props can be read easily and the state & return process can be carried out with the JSX code to the root component of the ReactJS application.
1.7 What is State in React?
In React, a state is a built-in object that is used by the React developer to contain information about the component. Here the state of a component can be changed at any time and whenever there is any change, the component re-renders. And the change in the state can happen as a response to system-generated events or user actions. These changes specify the component’s behavior and how it will render itself.
Example:
class App extends React.Component { constructor(props) { super(props); this.state = { createdBy: "TatvaSoft", year: 2022, type: "Mobile App" }; } render() { return ( <div> <h1>This App is created by {this.state.createdBy}</h1> <p> It is a {this.state.type}. </p> </div> ); } } |
1.8 What are the Key Differences Between Props and States?
State | Props | |
Usage | State in React can hold information about the components. | Props in React enable the data to pass from one component to other components as an argument. |
Read-Only | State can be changed. | Props are read-only. |
Child components | State cannot access child components. | Props can access Child components. |
Mutability | It is mutable. | Props are immutable. |
1.9 What is JSX?
JSX means JavaScript XML. JSX is a React extension that embeds JavaScript Code inside HTML elements. . When the developers use JSX, it allows them to make the HTML files easy to understand. With the help of the JSX file, the performance of the React app is robust. It also provides the developers with XML-like syntax to write in the same file as JavaScript code, and then preprocessors can transform those expressions into real JavaScript code.
Example:
const example = <h1>This is an example of JSX</h1>; |
The above code somewhat looks like HTML, But it is neither HTML nor JavaScript. It is JSX.
1.10 Why can’t Browsers Read JSX?
Web browsers cannot read JSX directly and the reason behind it is that they can only understand JavaScript objects. And JSX is not a regular & simple JavaScript object. Therefore, when it comes to running a React app created using JSX on the web browser, the developers need to first transform the JSX file into a JavaScript object. And this is done with the use of transpilers such as Babel.
1.11 What is Virtual DOM? & How It is Working?
Document Object Model (DOM) represents an HTML document with a tree structure that is completely logical. Each branch of this tree structure ends in a node, and a node is something that has objects in it. In React, one can find out the lightweight representation of the real DOM in the memory which is called virtual DOM. Basically, in a React application, when the state of an object changes, there will be a change in only that particular object in the real DOM and this is because of virtual DOM. So instead of updating all the objects of the app’s structure, virtual DOM only changes the modified object.
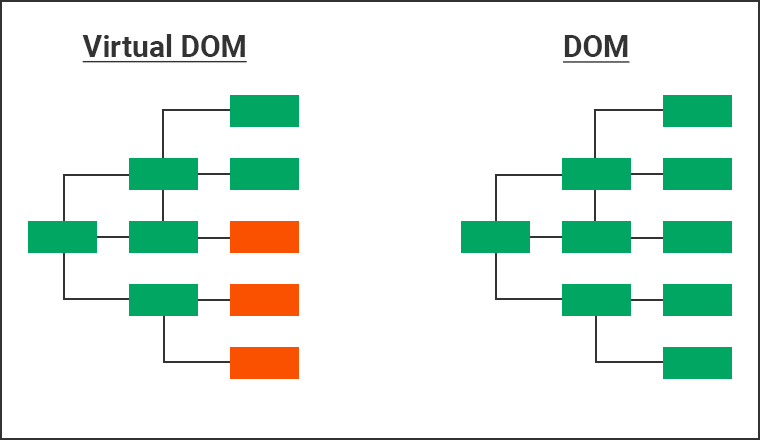
Further Reading On:
Top Node.JS Interview Questions and Answers
1.12 What are the Differences Between Controlled and Uncontrolled Components?
Controlled and uncontrolled components are the two different approaches that are used to handle input from React elements in applications.
1.13 Controlled Component
When it comes to the controlled component, the input element value is controlled by React. Here the input element’s state is stored inside the React code, and with the use of event-based callbacks, all the changes made in the React element can be easily reflected in the React code. This means that when any user enters valid data inside the input element of a controlled class component, a function name onChange is triggered and the validity of the entered value is checked inside the code. And if the entered value is valid, the change in the state is observed and the input element is re-rendered with the new value.
1.14 Uncontrolled Component
On the other hand, the uncontrolled component is a concept in which the input element’s value is handled by the DOM. This means that the input elements inside uncontrolled components act like normal input form elements of HTML. And here the input element’s state is completely controlled by the DOM. This means that no event-based callbacks are called when there is any new input element or change in the old one. Basically, in an uncontrolled component approach, React does not perform any action when there is any type of input element changes.
Controlled Component | Uncontrolled Component |
The controlled component is under the authority of the component’s state. | The uncontrolled component is under the authorization of the DOM. |
This type of component accepts the recent value as props. | This type of component accepts the values with the use of refs. |
It is controlled by the parent component. | It is controlled by the DOM. |
Controlled components have better control over the form values and data. | Uncontrolled components have limited control over the form of data and values. |
Maintaining the internal state with this component isn’t possible. | It helps in maintaining the internal state. |
1.15 What are the Different Lifecycle Methods of React?
React lifecycle comes with different components and each of them can be monitored or manipulated during the three main phases – Mounting, UnMounting, and Updating.
- Mounting: It means that all the elements are put into the DOM. When it comes to mounting a component, there are four types of in-built methods like – constructor(), render(), getDerivedStateFromProps(), and componentDidMount().The render() method here will always be called and the other three are optional.
- UnMounting: It is a phase in the lifecycle where the component is removed from the DOM. It comes with one in-built method called componentWillUnmount().
- Updating: This is the phase of the React lifecycle where the component can be updated whenever there is a change in the component’s props or state. There are five in-built methods when it comes to updating components and they are – render(), getDerivedStateFromProps(), shouldComponentUpdate(), componentDidUpdate, and getSnapshotBeforeUpdate().
When it comes to React class components, there are different methods and each lifecycle method has its own function components. Some of these lifecycle methods of React are –
- componentDidUpdate(): It is a method that runs immediately after any type of updating occurs in the application.
- componentDidMount(): It is a method that runs when the component output has been re-rendered to the Document Object Model (DOM).
- componentWillUnmount(): It is a method that runs before the unmounting of the component from the DOM. Besides, it is also used to clear up the space in the memory.
Besides the above-listed lifecycle methods, some other legacy lifecycle methods are rarely used. In addition to this, when it comes to a function component, the Hooks are used instead of the above-mentioned lifecycle methods.
1.16 What are Props in React?
The props in the React library are known as the inputs to a React component. Props are the objects that hold a set of values that will be passed to the React component while creating an app with the use of a naming convention that looks like HTML-tag attributes. To make it simpler, one can say that props are the app’s data passed from a parent component to a child component. The main aim of props is to offer various component functionalities like –
- Triggering state changes.
- Passing custom data to the components of React.
- Using this.props.reactProp inside the render() method.
Example:
function Laptop(props) { return <h2>Brand of this laptop is { props.brand }!</h2>; } |
function Brand() { return ( <> <h1>Laptop Brand</h1> <Laptop brand="Apple" /> //We can pass the data from one component to another, as a parameter. </> ); } |
const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Brand />); |
1.17 What is the Difference Between React Native and React?
Generally, it happens that people get confused between React Native and React. To solve this confusion, let’s understand both these technologies clearly.
React Native is a popular mobile framework that enables developers to create native mobile applications for platforms like Windows, Android, and iOS. It makes compiling native app components very easy. Besides, the app created with the use of React Native enables the developers to use React to create multiple components and implement React under the hood.
On the other hand, React is one of the most used JavaScript-based libraries. It supports both the front-end web development approach and the running of applications on the server. Developers use React to create robust user interfaces and web applications.
React | React Native |
React can be executed on different types of platforms. | With React Native, some extra efforts are needed to run the app on different platforms. |
It uses CSS and JavaScript libraries for animation. | It comes with built-in libraries for animation. |
React uses HTML tags while creating apps. | React Native doesn’t require an HTML tag. |
It is used for creating web apps. | It is used for mobile apps. |
React uses its own router to navigate web pages. | React Native comes with a built-in navigator library. |
2. Conclusion
As seen in this blog on react interview questions, React is one of the most popular libraries in the market, and in the last few years, it has got more popularity among the top IT organizations such as Instagram, Facebook, Uber, PayPal, and more. This is why techies are more interested in React and more & more developers are learning this technology. For them, we have listed some of the top React interview questions and answers which can help you prepare for your interview. Developers can also get React certifications from trusted online companies to become an expert in creating interesting UIs. Basically, having a proper understanding of all these basic yet important React concepts enables every developer to create a good first impression in the interview.
As a newbie in the field of web development, I was confused when I went on interview for a React developer position. However, this insightful article helped me to go through the complexities and crack the interview. Thank you for sharing this invaluable resource!