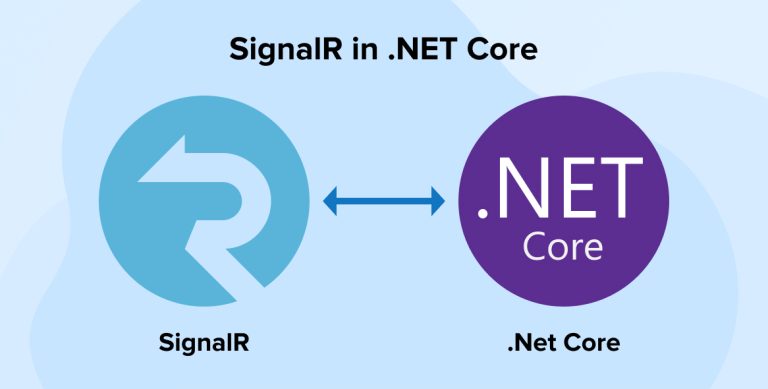
SignalR is known as one of the most popular libraries amongst .NET development companies for creating applications. Real-time functionality is a concept where the developers create an application in such a manner that the server-side code content is connected to all the clients as soon as the client is available rather than waiting for it to request new data from the server. SignalR communication service offers a bidirectional communication channel between the client side and server side of the application. And this service is not limited to web applications only, it can be used for any web page or application that uses .NET Framework 4.5 or JavaScript.
To know more about SignalR, what are its prerequisites, how to implement SignalR in .NET Core, and more, let’s go through this blog.
1. What is SignalR?
ASP.NET Core SignalR is one of the most popular used open-source libraries that help developers simplify adding real-time and robust web functionalities to applications. Real-time web functionality is something that allows the server-side code to push the stream data to the client side instantly after getting the request. This means that, in a real-time enabled process, the server-side code is written in such a way that it instantly sends content or data to the connected client as soon as it is available instead of waiting for the client’s new request to send data back.
For instance, let’s suppose that the real-time application is a chat application. Here, the server sends messages and data to the client as soon as the client is available. In this context, SignalR service can be used to also send messages as push notifications within the web application. Here, SingalR uses a digital signature and encryption to make a secure communication channel.
Some of the good candidates for SignalR service are –
- Some of the best candidates for SignalR are monitoring and dashboard apps such as instant sales updates, company dashboards, or travel alerts.
- .NET Core SignalR enables the applications to have to get high-frequency updates from the server side. These are the apps that need real-time updates at the friction of a second. For instance, social networking apps, gaming apps, GPS apps, voting or auction apps, and map applications.
- Applications like chat, travel alerts, games, social networks, and other applications that require notifications on an instant basis are because of SignalR.
- Collaborative apps such as team meeting software solutions and whiteboard applications are the best candidates for SignalR service.
SignalR Net Core service offers an API for creating remote procedure calls (RPC) to send data that goes from the server-to-client side. The remote procedure calls have the capability to invoke various functions on clients from server-side code. In this case, there are multiple supported platforms available, each of which has its respective client SDK. Because of this, the RPC call invokes the programming language which varies.
Features of SignalR Service
- This approach allows the messages to be sent to all the connected clients simultaneously.
- Every developer handles connection management automatically with the help of SignalR.
- With the SignalR service, sending messages to specific clients or groups is possible.
- SignalR Hub protocol is one of the most important features of this service.
- This service scales to handle increasing traffic.
2. Prerequisites
Some of the major pre-requisites of working with ASP.NET Core SignalR are –
- Visual Studio Code
Visual Studio Code, popularly known as VS Code. It is a source code editor developed by tech-giant Microsoft. It helps the developers write client code, with syntax highlighting, intelligent code completion, debugging, code refactoring, snippets, embedded Git, and more. Having knowledge of this source code editor is necessary for any developer who wants to work with SignalR.
- ASP.NET Core Web Application
ASP.NET Core is a high-performance and cross-platform, open-source framework that is used by the .NET development companies to create modern, internet-connected, and cloud-enabled apps. Knowing how to create these types of applications is a prerequisite when it comes to working on .NET Core SignalR.
- Basic Knowledge of ASP.NET Core
Another thing that is on the list of prerequisites for SignalR is having basic knowledge of .NET Core, a general-purpose software solution development framework. It enables the .NET developers to create various types of software solutions such as Mobile, Web, Desktop, Internet of Things, Gaming, Cloud, and more. And having a basic understanding of this technology is required to start working with SignalR service.
Further Reading On : ASP.NET Page Life Cycle
3. Steps to Implement SignalR in .Net Core
Here, let’s have a look at the steps that can help developers in SignalR configuration and implementation in ASP.NET Core –
STEP 1: The very first step is to create a web application project using the ASP.NET Core framework. As seen above, creating a .NET web application is a prerequisite for carrying out SignalR implementation, so we will go through the steps to define methods.
So, for implementing SignalR in ASP.NET Core, the developers need to first add the SignalR client library to the web application project. In order to add the SignalR client library, you need to follow the screenshot.
In Solution Explorer, right-click the project, and select Add > Client-Side Library.
Add Client-Side Library dialog:
- Select unpkg for Provider
- Enter @microsoft/signalr@latest for Library.
- Select Choose specific files, expand the dist/browser folder, and select signalr.js and signalr.min.js.
- Set Target Location to wwwroot/js/signalr/.
- Select Install.
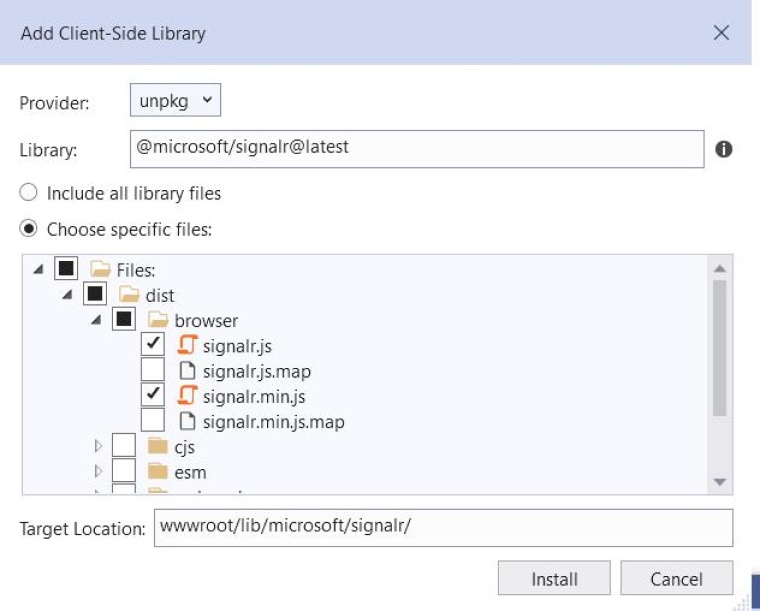
After you are done with the installation process, it’s time to create SignalR Hub: ChatHub Class. To do so, follow the below given .NET SignalR code.
3.1 // ChatHub.cs
using Microsoft.AspNetCore.SignalR; using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; namespace SignalrImplementation.Models { public class ChatHub : Hub { public async Task SendMessage(string user, string message) { await Clients.All.SendAsync("ReceiveMessage", user, message); } } } |
Now after that, you need to add a service reference in the startup.cs’s ConfigureServices method. For that follow the below code.
3.2 // startup.cs
public void ConfigureServices(IServiceCollection services) { services.AddControllersWithViews(); services.AddSignalR(); } |
Now, you can add a chat hub class in the Configure method in startup.cs as shown in the below code.
// startup.cs
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Home/Error"); app.UseHsts(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting(); app.UseAuthorization(); app.UseSignalR(routes => { routes.MapHub("/chatHub"); }) app.UseEndpoints(endpoints => { endpoints.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); }); } |
After this, it’s time to create a new JavaScript file for HubConnection, as soon as in the below code.
3.3 // chat.js
const connection = new signalR.HubConnectionBuilder() .withUrl("/chatHub") .build(); connection.on("ReceiveMessage", (user, message) => { const msg = message.replace(/&/g, "&").replace(//g, ">"); const encodedMsg = user + " :: " + msg; const li = document.createElement("li"); li.textContent = encodedMsg; document.getElementById("messagesList").appendChild(li); }); connection.start().catch(err => console.error(err.toString())); //Send the message document.getElementById("sendMessage").addEventListener("click", event => { const user = document.getElementById("userName").value; const message = document.getElementById("userMessage").value; connection.invoke("SendMessage", user, message).catch(err => console.error(err.toString())); event.preventDefault(); }); |
This was all about the logic that goes behind the implementation process. Now it’s time to create a User Interface for the Chat test.
3.4 // Index.cshtml
@page <div class="container"> <div class="row"> </div> <div class="row"> <div class="col-md-12"> <div class="col-md-6"> <div class="col-md-3">YourName</div> <div class="col-md-9"></div> </div>; </div>; <div class="col-md-12"> <div class="col-md-6"> <div class="col-md-3">Message</div> <div class="col-md-9"> </div> </div> </div> </div> <div class="row"> <div class="col-12"> <hr /> </div> </div> <div class="row"> <div></div> <div class="col-6"> <ul id="messagesList"></ul> </div> </div> </div> |
After following the above step, we are done with the SignalR implementation process in .NET Core. Now, you can run the demo application in the web browser as shown below.
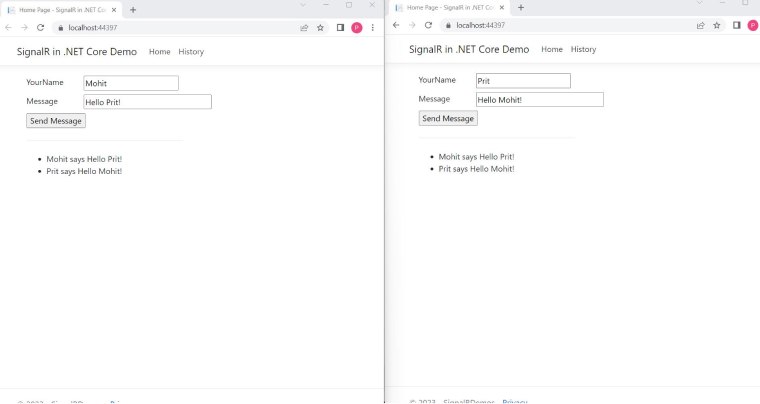
4. GitHub Repository SignalR in .NET Core Example
Some of the best examples of GitHub repository SignalR samples in .NET Core are –
- MoveShape
- ChatSample
- AndroidJavaClient
- WhiteBoard
- PullRequestR
- WindowsFormsSample
Further Reading On : .Net Core Dependency Injection With Example
5. Conclusion
As seen in this blog, SignalR is a technology that has the capability to identify the process of creating real-time web functionality. It comes with a Javascript client library and ASP.NET Site server library, making it easier for the developers to push content updates to the client side from the SignalR Hub and manage client-server connections. While using this service for web apps, the developers need to add the SignalR library to an existing application created in ASP.NET in order to have real-time data and functionality. SignalR service helps .NET development companies create robust, high-performing, and real-time web applications.
Comments
Leave a message...