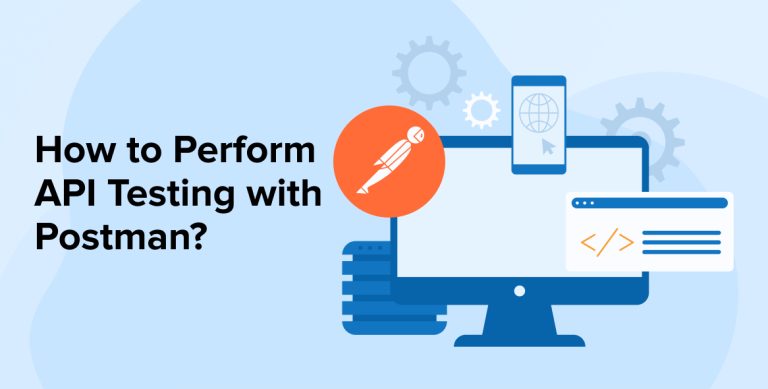
Application Programming Interface (API) is vital in the modern digital world because it allows data to be transferred between various software systems. Consequently, evaluating these APIs is now more important than ever. Today’s software development and QA services would be incomplete without testing application programming interface (API) to guarantee they are dependable, perform as expected, and provide the intended functionality. Here, we’ll explain to you how to start API testing with Postman, which is a strong API testing tool in its own right.
1. How to Test API Using the Postman Tool
Let’s examine how to test API using the Postman Tool, here are the steps that we need to follow:
1.1 Installation and Setup of Postman
To perform Postman API testing, you must have a Postman account to proceed with this guide. Make an account for free if you haven’t done so before. After that, go to your Postman workspace and begin.
In this part, we will develop a User Account Info API. Further on, we will compose integration and functional tests for it. We will save these tests in a fresh set named AccountInfo-API Tests. A functional test examines how one API endpoint behaves to ensure the request-response cycle works as expected. However, integration tests verify that all user flows, which may include two or more endpoints, function as intended.
Now you will notice the collection on the left sidebar of your workspace:
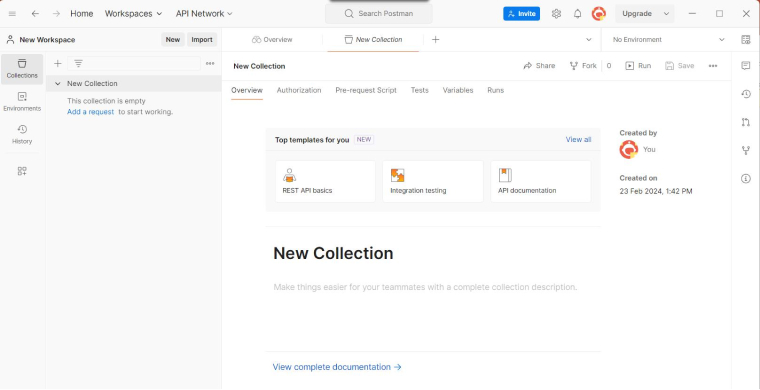
1.2 Create Workspace
You and your coworkers may set up a Postman workspace to manage your API and collaborate.
In Postman, you’ll find three different sorts of workspaces:
- As its name implies, a personal workplace is one that an individual uses exclusively.
- Only others you invite to work with you in your private workspace will have the access. The next step is to create folders for your files and then distribute them to others in your workspace.
- You may make your APIs available to everyone with a Public Workspace. No cost is required to access them, and they can be searched.
All the Postman’s workspace types—Personal, Private, and Public—offer Postman API testing capabilities.
1.3 Create Postman Collections
Among Postman’s most useful features is the Collection Runner, which lets programmers perform several requests simultaneously. When testing an API, the Collection Runner is a useful tool for setting up test suites, defining variables, and generating reports.
You may construct a collection to bundle several API queries for testing purposes. Pick “Collection” from the drop-down menu after clicking the “New” button to start a record. As a memory aid, label the set with a title and its description.
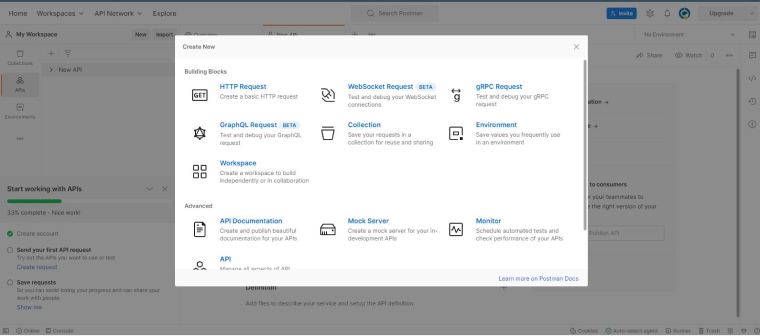
1.4 Create User Requests
Making requests to store our tests is the next step. One way to express an API endpoint is in a request. Select the drop-down option that shows on the right side of the AccountInfo-API Tests collection.
Each request’s tests must now be written. You may develop tests using the syntax of JavaScript with Postman’s built-in API testing tool. You may retrieve the response received from a request to write test scripts, and tests are conducted right away after the request is run.
1.5 GET Requests
The most common method for getting data from an API is to utilize GET requests. To initiate your first request using Postman, please refer to the instructions below.
To add a new HTTP request, simply click on the collection you would want to utilize and finally, click the “Add request” link.
- Choose a name that best describes your HTTP request.
- No action is required on your part as the GET method is already chosen.
- The endpoint URL can be set using the URL field.
- To send, just click the button. Postman will display the result after sending the request to the specified endpoint.
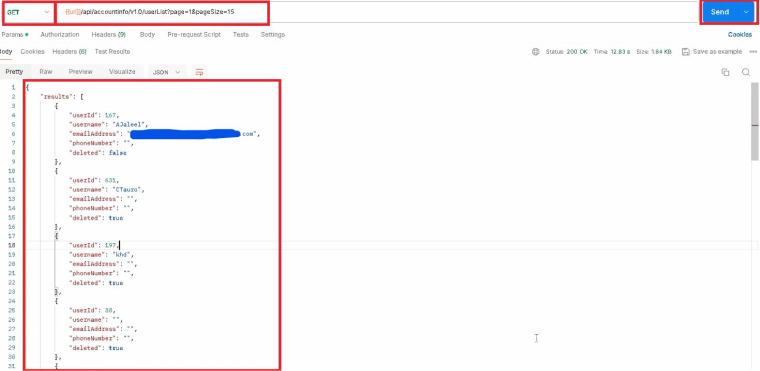
1.6 POST Requests
A request body is an optional component of POST requests, in contrast to GET ones. In the request body, Postman lets you provide data in several forms.
How can you use Postman to deliver POST requests that include form data?
- Put in a fresh request.
- Just click on the POST HTTP method in the selection menu.
- In the URL area, provide your API endpoint.
- The request body may be customized by clicking on the Body tab.
- Please choose form-data.
- Use the KEY column to set the title of the entry.
- Use the VALUE column to set the contents of the entry.
- To send, just click on the icon.
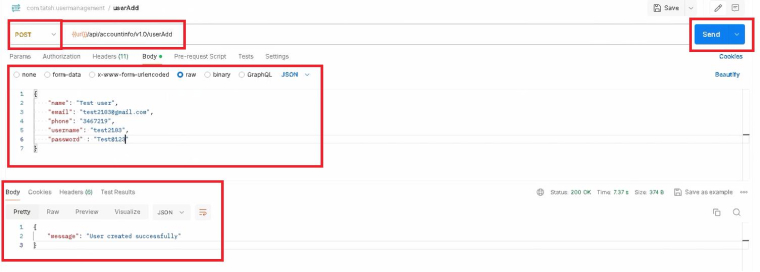
1.7 PUT Requests
PUT requests to insert or modify an existing entity.
Methods for making a PUT request:
- Make use of the following service URL: “{{url}}/api/accountinfo/v1.0/userEdit” with the following payload for user id : 23105.
{ “name”: “Test User”, “email”: “test2103@gmail.com” “phone”: “1234567890”, “username”: “test2103”, “password”: “Test@456” }
- To add the payload script, open the POSTMAN client, head to the PUT method, click on the Body section, pick RAW, pass JSON, and finally, choose JSON from the dropdown menu.
- Data is stored in the key-value format and JSON begins with curly brackets.
If your request was successful, you will see the following answer once you press the SEND button.
- Response Status Code 200 has been successfully received.
- Response Payload.
- Header.
- Name, Email, Phone, UserName, and Password Updated as Requested.
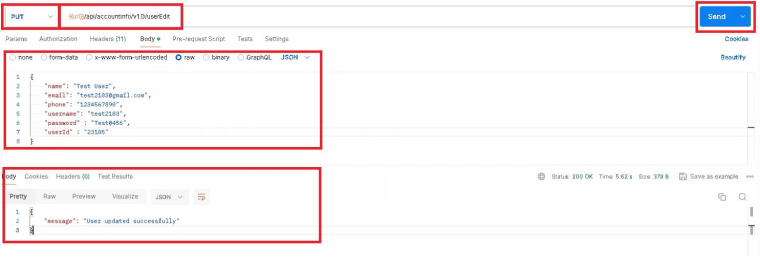
1.8 Delete Requests
Deleting data is handled via Delete requests in Restful APIs. Follow these simple steps to submit a DELETE request to an API endpoint of your choosing:
- Make a new request.
- Pick the DELETE HTTP requests from the menu.
- To provide the final destination URL, use the Url field.
- Select “Send” from the menu.
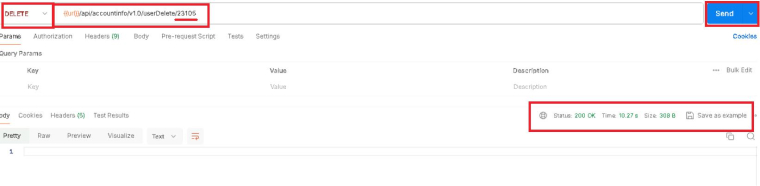
1.9 Parameterize Requests
One of the best things about Postman is the data parameterization. Use variables with parameters over repeatedly making the same queries with different data. This information could originate in an environment variable or a data file. By using iterations and parameterization, the automation testing process becomes easier, and the same tests are avoided.
Double curly brackets, represented by {{sample}}, are used to construct parameters.
Now we can make a GET request that takes parameters.
- To initiate a GET request, configure your HTTP settings.
- Setting the environment is necessary to utilize the parameter.
- Click Save after changing the address of the variable to the URL like- https://localhost:44366/ or https://yoursitename.com/
- To proceed to the next screen, click its “close” button.
- Before you hit “Send,” return to your “Get” request.
The outcomes of your request are likely to be visible.
1.10 Create Postman Tests
Requests can have Postman Tests, which are pieces of JavaScript code, appended to them to check for things like success or failure and to compare actual outcomes to expectations. In most cases, it will begin with pm. test. It is similar to the assert and verify commands seen in other tools.
Using the parameterized requests, let’s put Postman to work testing our API.
Step-1:
- Navigate to the testing pane. Snippet codes are located on the right side.
- Select “Status code: Code is 200” from the section containing excerpts.
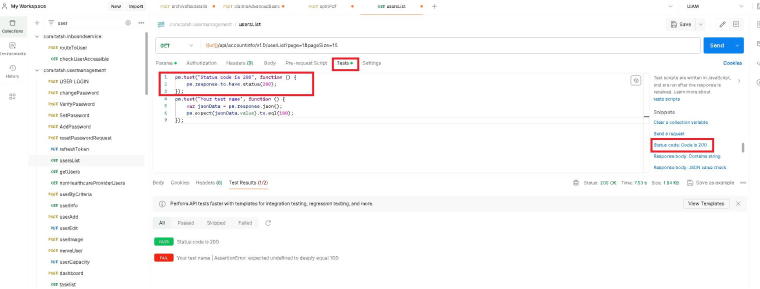
Step 2: Hit the “Send” button. You should now see the test results.
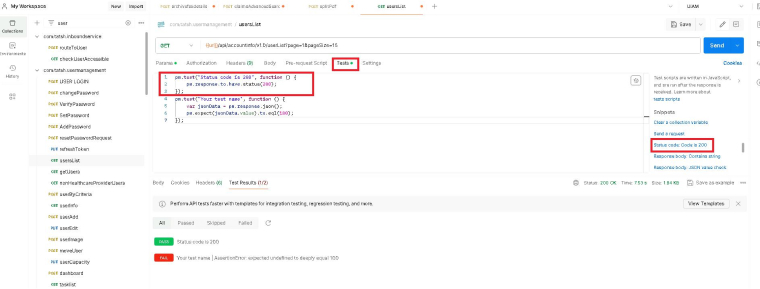
Step 3: Return to the tests page and create another test. Now let’s see how the real outcome stacks up against the predicted one.
Select “Response body: JSON value check” from the snippets section. We will verify that jojo_amber has the userid 1.
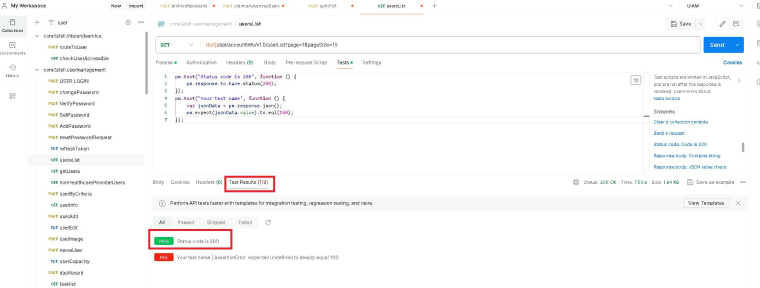
Step-4:
- To make the test name more specific, we should replace “Your Test Name” with “Check if user with 1721 is jojo_amber” in the code.
- Please use jsonData[0].name instead of jsonData.value. Review the body of the previous GET result to obtain the route. The first result, jsonData, should begin with 0 as jojo_amber is 1721. Utilize jsonData[1] to obtain the second result, then continue with subsequent results in the same manner.
pm.test("Check if user with 1721 is jojo_amber", function () { var jsonData = pm.response.json(); pm.expect(jsonData[0].name).to.eql("jojo_amber"); });
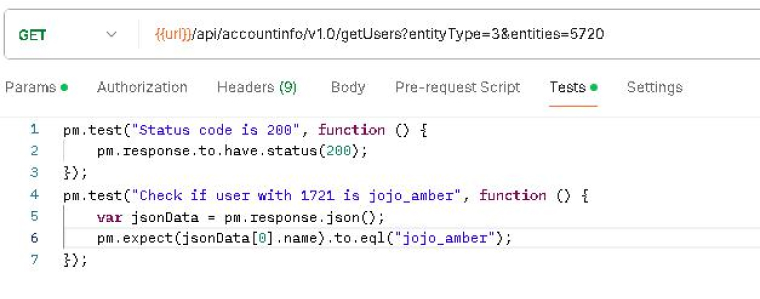
Step-5: Hit the “send” button. Two tests passed for your request now.
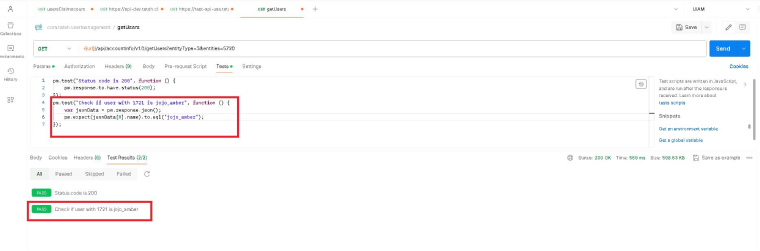
It should be noted that the Postman test allows for the creation of several types of test suite. Check out the tool and see if any of the tests are a good fit for your requirements.
1.11 Use the Collection Runner
Using Postman’s Collection Runner, you may execute several requests inside a collection or tests tab at the same time. Select the “Runner” button located in the upper-right portion of the Postman window to access the Collection Runner. To begin running a collection, choose it and then hit the “Start Run” button.
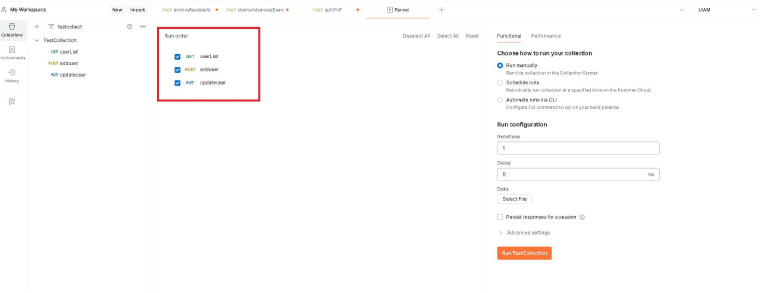
1.12 Analyze the Test Results
After the requests have been executed by the Collection Runner, the test results will be shown in the Postman interface. You may check the details of each request and see which ones test passed and which ones failed.
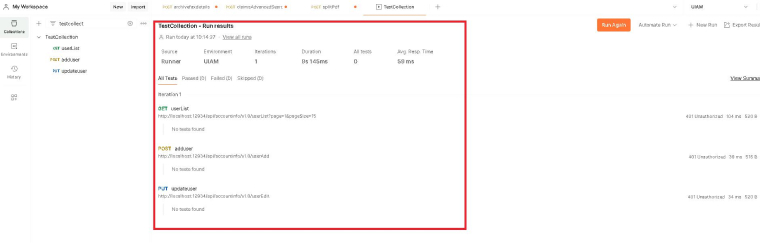
2. Conclusion
Our article examined the significance of conducting API testing for your application and how to implement it with Postman. Developers have greater control and flexibility in their testing workflows because of its extensive features, which allow them to create custom test cases, generate reports, and conduct load testing.
For the last point, Postman is an excellent tool for API development, testing, documentation, and mocking. Have fun coding!
FAQs
Is Postman the best for API Testing?
Postman remains one of the first tools most businesses use for API testing. It gives teams the ability to promptly fulfill their API testing requirements without requiring them to acquire a new, complex tool or framework. It provides all the functionality required to effectively test and automate APIs.
What are the 4 methods of API testing?
The four primary categories of API testing techniques are Delete, PUT, POST, and GET.
Which API testing is better than Postman?
The answer is Hopscotch. An open-source tool for developing and testing APIs, Hopscotch may be used as a substitute for Postman. Its interface for testing and debugging APIs is easy to use.
How to do API testing?
Follow the below steps to perform API testing:
- Recognize the needs of the API.
- Give the output state of the API.
- Concentrate on compact, useful APIs.
- Select an appropriate test automation tool.
- Start performing.
Comments
Leave a message...