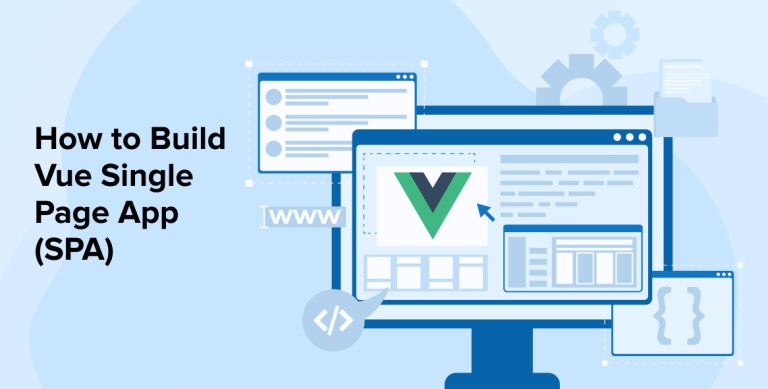
This article will demonstrate how to set up a local Node server and run a Vue single page app. This local server utilizes hot module reloading through Webpack to render changes as you make them in the browser, giving you constant feedback. Single-file components (SFC) in the .vue file format, including header, will be created as you go. What you will learn here may serve as the cornerstone of any new project that you might handover to a Vuejs development company.
1. Overview of Vue.js
Vue.js is a widely used UI framework written in JavaScript. Vue.js, which was developed in 2014 by Evan You (formerly of Google), is frequently compared with both React and Angular. It takes inspiration from both frameworks, namely their prop-driven approach to development and their powerful templating capabilities. Since it utilizes only standard HTML templates and CSS, Vue is much easier to learn than other frameworks like React and Angular, which employ CSS in JavaScript code.
It’s important to learn how to use the Vue components before beginning a new project. Vue CLI 3, the latest version of Vue.js’s command line interface (CLI), is a useful tool. The Vue CLI’s capability to produce and pre-configure a new single-page application with the Vue create command is the major feature that sets it apart from other Vue development tools.
2. What is a Single Page Application?
A Single Page Application (SPA) can be anything around the web–be it a web page, website or a web application that functions within a web browser and renders a single file at a time. It doesn’t require refreshing the page during use as the majority of its content is static. The SPA leverages HTML, CSS and JavaScript application programming interfaces to make the necessary changes when the data has to be changed or updated.
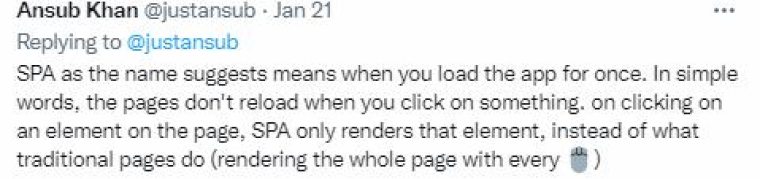
For creating cutting-edge SPAs, Vue library offers a fantastic development mode together with its core libraries and extensive tools support.
- Client-side router
- Rapid development environment
- Software that helps with IDEs
- Developer tools for your browser
- TypeScript integrations
- Testing utilities
You may achieve the advantages of a single-page application (SPA) while continuing to use a server-centric development strategy by combining Vue with a solution like Inertia.js.
3. Steps to Create Vue Single Page App
3.1 Download the Latest Version of Vue CLI
Vue CLI may be obtained by issuing a command using npm or Yarn. Node Package Manager (npm) allows you to easily add third-party libraries to your project. However, Yarn offers further advantages like caching while still executing NPM tasks in the background. Which one you use is a matter of preference. However, keep in mind that it is not advisable to combine orders. Choose one and stick with it throughout the project if you can.
Following this section, npm commands will be used throughout the remainder of this article. To get the Vue CLI files from the registry, namely the npm (Node Package Manager) service, use the following command:
npm i -g @vue/cli |
Note: Be aware that having installed a npm package worldwide may fail on some systems due to a permission problem. Instead use sudo for npm install, which is against security best practices, you may change where npm stores its defaults. If an EACCES error occurs, proceed as described in the npm documentation.
With this installed system-wide, you may use the command line interface (CLI) from any location on your computer. In the absence of a global installation, this will function just in the directory in which it was placed. The “i” option in a command indicates “install,” while the -g flag installs the program system-wide.
Execute the preceding to see if Vue CLI was installed correctly:
vue --version |
What follows is the output, along with a version number. Though the version number returned may be different, it indicates that Vue CLI was installed correctly.
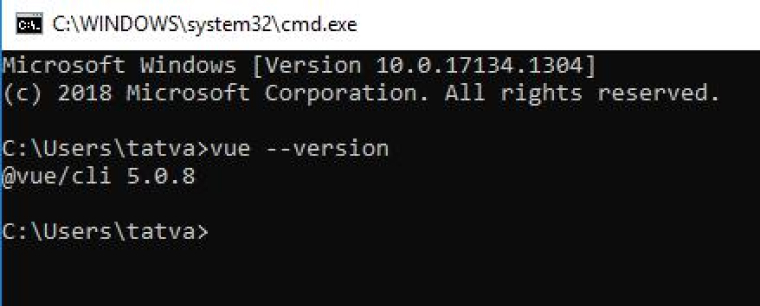
You should now have npm installed globally, as well as install Vue CLI tools, both of which will be used to produce a Vue.js project in the following stage.
3.2 Generating a Single-Page Application
Since setting up a new Vue.js project from the start might take hours, you may discover that manually creating a project isn’t the most effective use of your time as you create new Vue application/s. Vue CLI’s real strength is in its ability to construct a template tailored to your needs. This means you can immediately begin working on your web or app project because everything is already set up for you.
From a command prompt, Vue CLI will gather some information about your project, grab any resources it needs, and set everything up so you can start the development right away.
Start by creating the project directory you’d want your Vue project to live in, and then execute the following Commands to create a new single-page application:

In the highlighted portion of the command, you can see the whole name of the parent directory. What you provide here will become the name of the directory where your Vue.js files will be stored. In this article, we’ll be using the “single_page_app” as our directory name, but you’re free to use any other name as well.
When you’re through with the command, hit Enter to proceed. The following prompt will then appear:
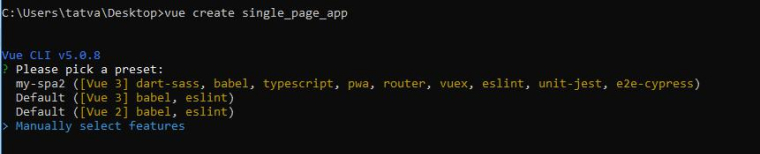
The Vue 2 or Vue 3 defaults are suitable if you don’t want to customize your project. However, we will select the features manually for this article. You may observe the CLI’s implementation of each option you pick by using the selection menu.
Select “Manually select features” and after that click Enter. Instantly, you’ll be presented with a plethora of options, such as: Choose Vue version, TypeScript, the Vue Router, and Vuex. Some of these options have been checked off before. Pick as many as you like; there’s no limit. However, for this post, choose the following by hitting <space> on the entry:
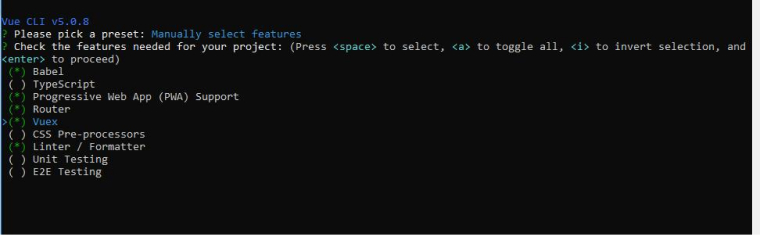
When you’re ready to proceed with your selections, tap the Enter key. The command line interface (CLI) will follow up with inquiries about each option you’ve chosen for your project. The first inquiry concerns your preferred Vue release: 2.x or 3.x. In this article, we’ll be using Vue 3, however if you’re looking for more help from the Vue community, Vue 2 may be a better option.

Vue CLI will then inquire as to whether you want to use history mode. While using the history mode, each route will have its own unique URL. Using the project’s history mode requires a Node web server.
Type Y to confirm that you want to view the history. It also removes hash from the URL and makes the URL path normal. Here we are selecting N to keep the hash in the URL.

You will then be asked some questions about the linter’s formatting preferences. In the process of software development, a linter is used to inspect all the code and provide feedback. Multiple syntactical rules may be enforced by this linter as code is being written. Moreover, your IDE will be able to interpret the settings included in this configuration file and apply them to the code automatically once you save it. Your code will remain constant regardless of the developer, their operating system, or the integrated development environment (IDE) they use.
Choose ESLint + Prettier config:
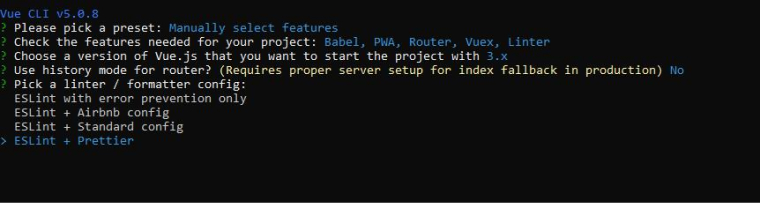
In doing so, we pick a set of guidelines that ESLint will check against. Options such as utilizing semicolons at the end of lines or const instead of var in JavaScript are examples of these setups.
The second setting determines when your code will be formatted by ESLint. This can occur either when you save your work or when you push your changes to a hosting site like Github, GitLab, or BitBucket. Lint on save allows you to inspect changes before committing them to version control, which is why it is highly advised to use.
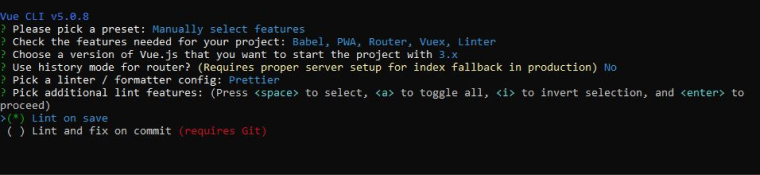
Once you’ve decided which lint features you want to use, Vue CLI will ask where you’d want to keep your configurations—in separate files or in the package. json. There are a few reasons why it’s common practice to keep settings in their own files. First, you’ll be able to preserve your package, and second, you’ll save time when switching between projects by sharing the same configurations to make your app’s content as readable as possible.
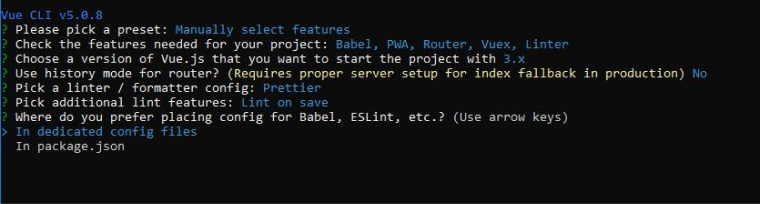
The CLI tool will enable you to store your preferences as a default for future work after you’re done. You may use this if you’re in the business of creating projects for clients and want things to seem professional.
Creating a preset from this configuration is a good idea; Vue CLI will prompt you to rename the preset before saving.
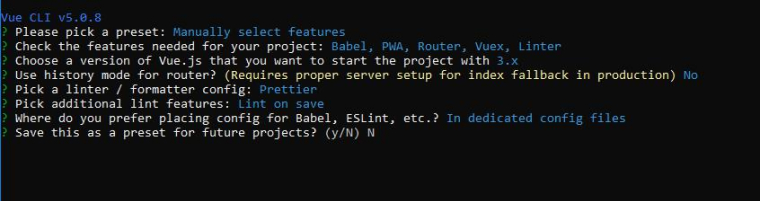
You can take these parameters and apply them to another project.
Now, on your terminal, you should see a summary of your choices that looks like this:
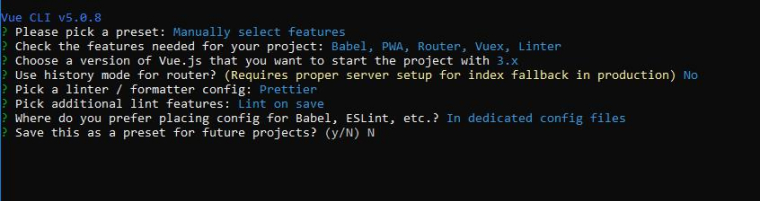
As soon as you hit Enter, Vue CLI will begin developing your app.

Once you’re done, cd (change directory) to the project’s directory (single_page_app):
Then, type “npm run serve” to launch the application. In most cases, this will cause your app to launch on localhost at the port number 8080. If the port is different, the command line interface will indicate as much.
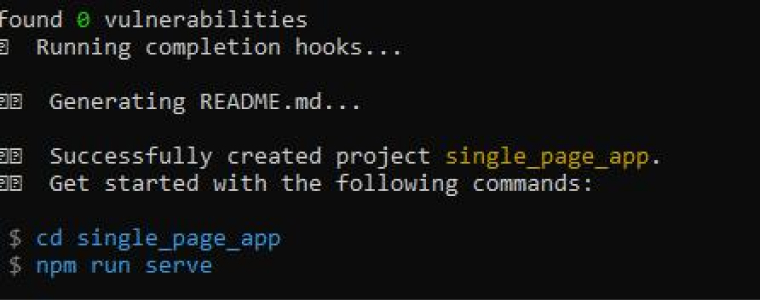
The CLI has already downloaded the necessary dependencies, so you don’t have to. Start your preferred web browser and type localhost:8080 to see the output of your build. The Vue logo and the technologies you choose in the setup wizard will be shown on a welcome screen.
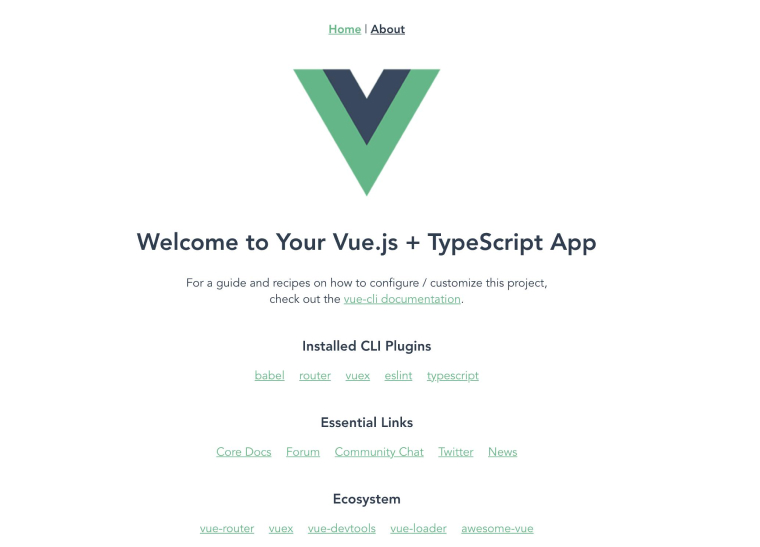
This server can be left active for the duration of the lesson so that you can track your progress.
A variety of alternatives relevant to your project were picked in this area. Vue CLI grabbed all the necessary files and set them up in advance. You may now begin modifying the produced code base and building your own single file vue component.
3.3 Creating a Header Component
Create a header for your Node server-hosted single-page application. For this, open the 2 files available in the view folder. You can edit HomeView and AboutView files as you require. You can also add other vue single file components.
Let’s add one basic Header component.
Step 1: In the components folder, create the “HeaderComponent.vue” file and add the following code in that.
Here the .vue extension indicates that this is a vue single file component. We can consider each vue component as a vue instance.
<template> <div> <p>Header Component</p> </div> </template> <script> export default { name: "HeaderComponent", props: { msg: String, }, }; </script> |
Step 2: Update the “App.vue” file. Include the Header Component in the same. Your updated “App.vue” file will look like this:
<template> <div id="nav"> <headercomponent> <router-link to="/">Home</router-link> | <router-link to="/about">About</router-link> </headercomponent></div> <router-view> </router-view></template> <script> import HeaderComponent from './components/HeaderComponent.vue'; export default { name: "App", components: { HeaderComponent } } </script> <style> #app { font-family: Avenir, Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; } #nav { padding: 30px; } #nav a { font-weight: bold; color: #2c3e50; } #nav a.router-link-exact-active { color: #42b983; } </style> |
Open a new terminal window, go to the “single_page_app” folder’s root, and execute the following command to run the project:
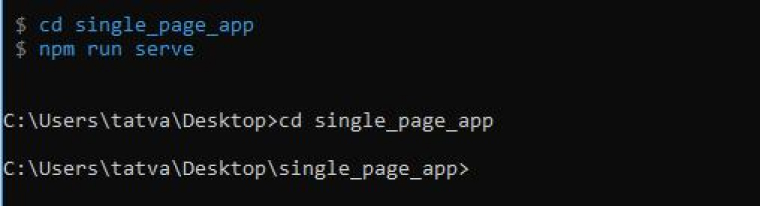
The following output is what you can expect:
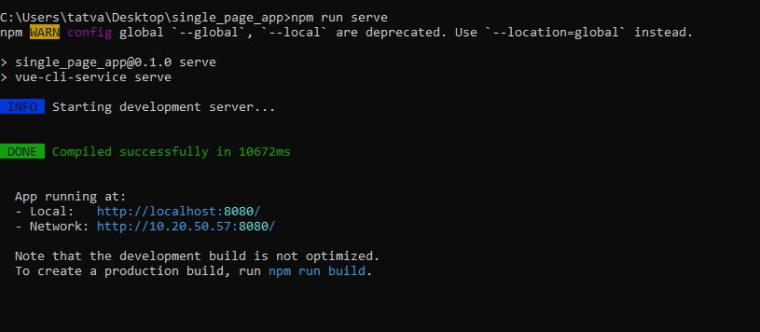
Vue Single Page App:
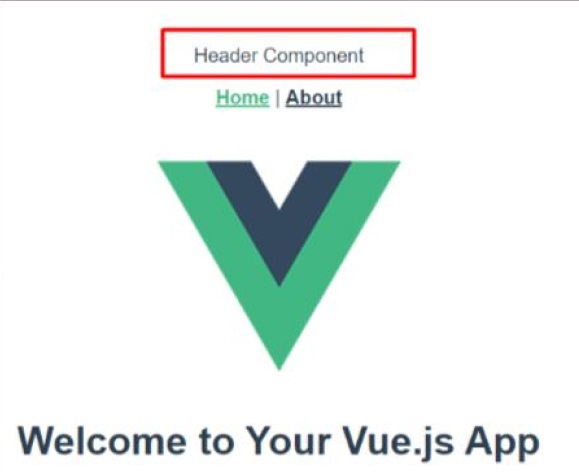
Furthermore, you may inspect the project’s files by opening them in an editor like Visual Studio Code. Many different kinds of data and folders will be available to you either way. Due to the choices made during the initial development of this project, the corresponding configuration files may be found under the root directory. With this step, it will be possible to instruct Vue CLI to generate dedicated config,js files for each service you employ, such as Babel, TypeScript, and ESLint, by means of a specialized configuration file. There are also a lot of other folders beyond these files.
Let’s look at the folder structure of our application:
“Single_page_app” folder.
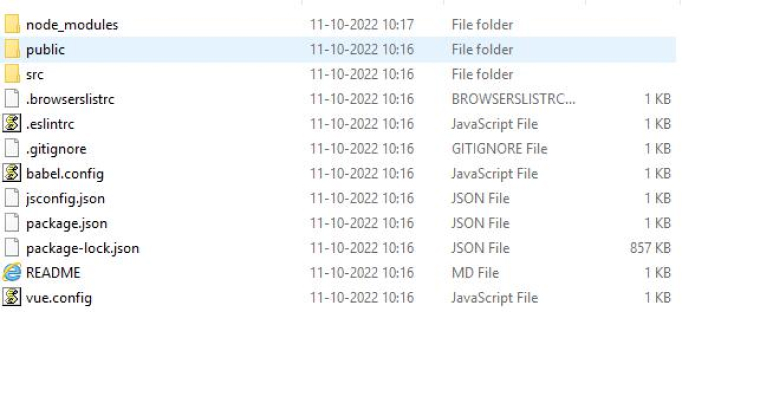
Public Folder
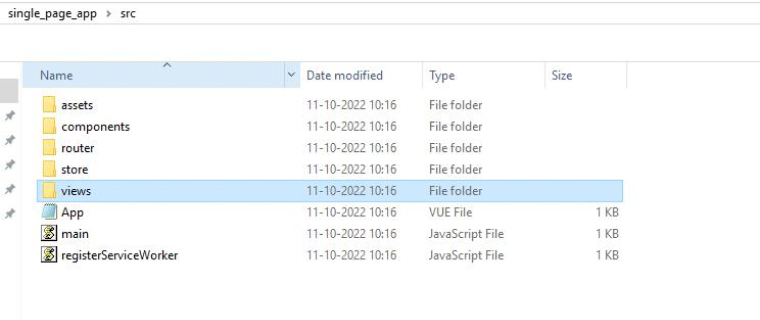
Views Folder:
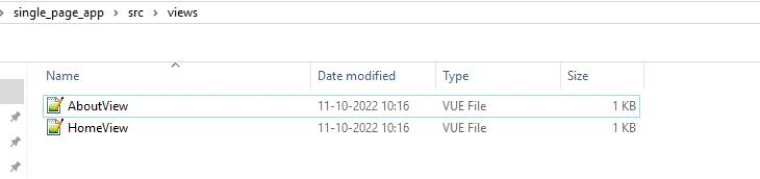
Components Folder:
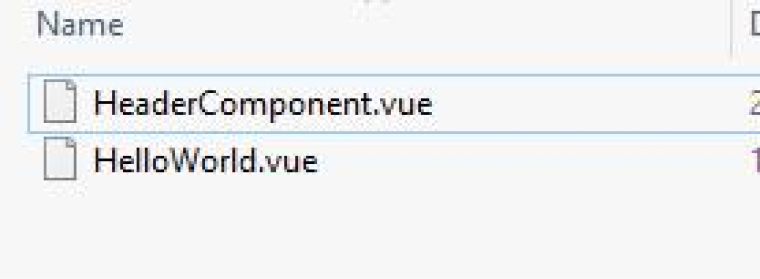
4. Conclusion
Using the Vue CLI, you can now build your own Vue single page app. You have learnt how the various parts of a Vue app work together and have successfully built a Vue Single Page App using features you choose during the preliminary setup. You’ve also looked over the framework that underpins most SPAs, so you should be able to leverage that knowledge to take your endeavor in new directions.
You may take advantage of a wide variety of resources inside the expanding Vue.js ecosystem. These programs allow you to save time and get things rolling quickly by allowing you to store default settings. We’ve just scratched the surface of what the Vue.js ecosystem has to serve, but the command line interface (CLI) is likely to become an indispensable resource along the way.
Comments
Leave a message...