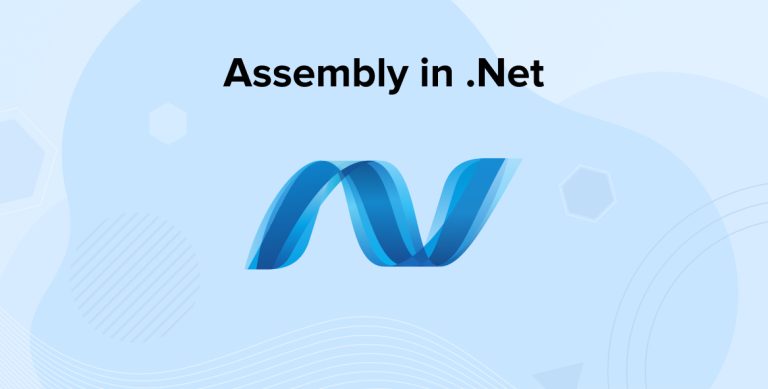
Assembly in .net is a common concept for .NET programmers to face. To successfully construct and release .NET programs, familiarity with assemblies is required.
This tutorial will explain what assemblies are, how they function inside the .NET framework, there many kinds of assemblies that may be used by a .NET development company, how to build assemblies, and their features.
1. What is an Assembly?
Assemblies are groups of related kinds and resources that have been designed to perform as a single entity. When it comes to .NET applications, assemblies are the building blocks for execution, version control , reusing, activation scoping, and safety controls.
For Microsoft .NET-built components, the .NET assembly is the gold standard. Assembly files created with the .Net Framework might be either executable programs (.exe) or non-executable dynamic link libraries (DLL). All .NET assemblies provide a manifest, meta-data, and edition details regarding the types they define. The component (assembly) resolution is an area where the .NET developers have put in a lot of time and effort.
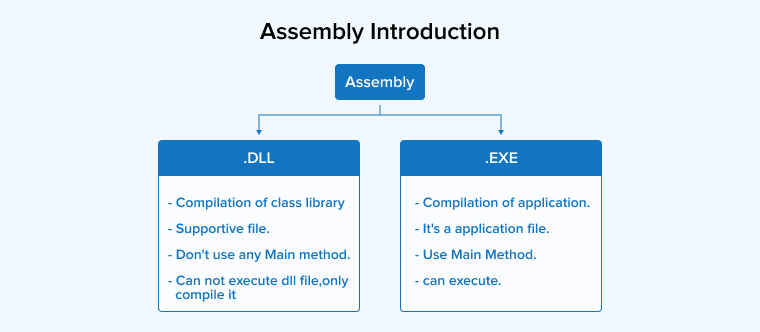
An assembly can be just one file or an ensemble of files that function as a logical unit. The manifest is located in one master module in a multi-file setup, while the remaining modules are simply regular old assemblies. In .NET, a module is a component of an assembly that consists of multiple files. In addition to remarks and features, assembly is an intriguing and useful facet of the .NET architecture.
2. Role of Assembly in .NET Framework
The .NET framework relies heavily on assemblies. They supply both code that might be reused and knowledge about previous versions, making it simple to perform upkeep and roll-out upgrades. There are various .Net development tools available to assist developers in making, managing, and debugging assemblies for use with the .NET framework.
They may be written in a variety of languages, including C#, VB.NET, and F#. In addition to the code, they may be used to include other resources like graphics, audio, and configuration files.
An assembly’s accessibility depends on whether it is a private assembly (only available to the program using it) or shared (visible to numerous apps).
A digital certificate may be used to sign assemblies, adding an extra layer of security and credibility. Assemblies are vital to the .NET framework for the development of stable, versatile applications.
3. What are DLL and EXE in .NET Ecosystem?
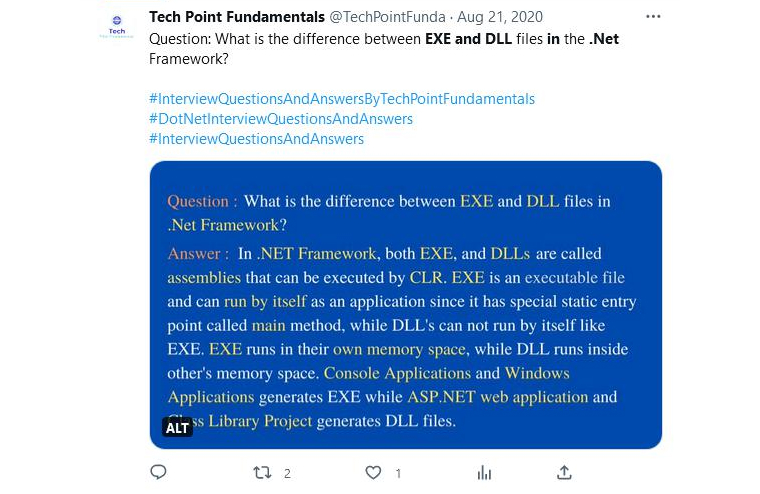
In computer science and software engineering, DLL and EXE are often utilized. When a programmer is finished with an application or project, they can choose to export it as either an executable (EXE) or a dynamic link library (DLL). But what do these words actually mean?
Since you’re probably more acquainted with the term “EXE,” we’ll start there. The acronym EXE stands for “executable.” At a minimum, every .NET application package will have an executable (EXE) file, which can be accompanied or not by a dynamic link library (DLL). There is a location in the source code of an exe file that serves as the starting point for the execution of the application’s code. When an EXE file is run, the operating system gives it its own section of memory to use.
Conversely, EXE files and libraries may make use of DLLs, which stand for Dynamic Link Libraries and include routines and procedures. Because DLL is a library, you can’t just run it. There will be a mistake if you do it. In contrast, a developer can use the DLL’s function name and its signature to call the DLL from a different program.
This functionality makes DLLs ideally suited for delivering software for device drivers. A DLL file, in contrast to an EXE, does not allocate its own memory space but rather uses the stored data of the application that calls it.
4. What are the Types of Assemblies in .NET?
In .NET, there are two kinds of assemblies: private assembly and shared assembly. Private assemblies are utilized by particular applications and are unable to be shared. However, numerous apps can use shared assemblies simultaneously. To make it easier to access and deal with shared assemblies, the .NET Framework features include Global Assembly Cache (GAC). Let’s discuss them:
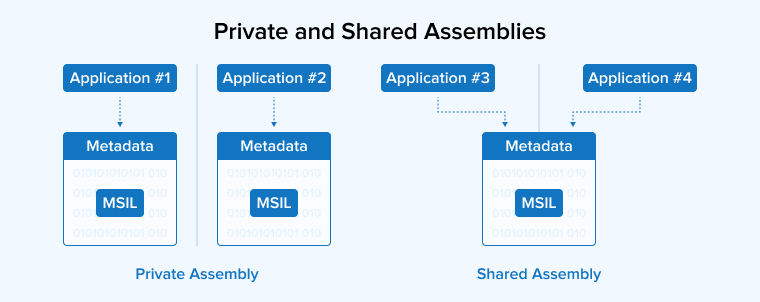
4.1 Private Assembly
They are most common in settings where resources do not need to be shared on a large scale. They are normally hidden from view and kept in the application’s directory. Since each program may utilize its own copy of the assembly folder without impacting the others, private assemblies are also helpful for updating.
4.2 Shared Assembly
A shared assembly is also known as a public assembly. Consider the case where we need to develop DLL files that can be used in a variety of contexts and consumed by various programs. The assembly is now stored in the system-wide assembly cache rather than within any individual program. Besides, as a shared assembly is an assembly that several people can use, there is only one copy of it, and it cannot be copied into other applications.
Alternatively, a global assembly cache is just a hard drive file wherein all the shared assemblies are stored. The application’s necessary repositories may be found in the GAC(Global Assembly Cache). Each shared assembly consists of –
- Face Name
- Public Key Token
- Version
- Culture Data
5. Structure of an Assembly
An assembly consists of the following four components, such as Assembly Metadata, Type Metadata, Microsoft Intermediate Language (MSIL), and Resources.
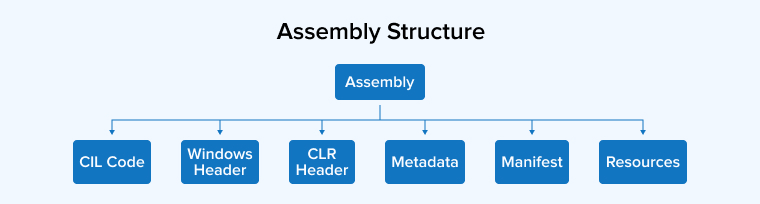
5.1 Assembly Metadata
Everything is explained in the assembly’s overall information. The metadata of an assembly can also be referred to as the assembly manifest. The assembly metadata contains information such as the following:
- The formal title for the particular assembly.
- The version number or build number.
- The favored environment for the assembly.
- These provide the originator of the assembly with a unique identifier.
- Here you may find a complete list of the assembly’s component files.
- Provides a complete catalog of all assembly references.
- Reference data is included for all exported classes, approaches, properties, and other components of the assembly.
5.2 Type Metadata
Type metadata describes the nature of an assembly. Type metadata can also refer to publicly available resources and types. Resources are things like picture files and other things the program needs to function properly.
5.3 Microsoft Intermediate Language
MSIL is essential to the ability of .NET to be used in any language. All programs are translated into the same machine-independent language, regardless of the language used to write them. Now that all languages translate to the same MSIL, features like encapsulation, inheritance, polymorphism, exception handling, and debugging are independent of the underlying language. .NET’s ability to function autonomously is likewise based on MSIL. By using MSIL, you can execute it anywhere by writing just once.
5.4 Resources
Either the assembly’s own resources or resources from outside the assembly can be inserted in a .NET assembly with relative ease. It’s easy to get at the stuff you need for an assembly. Some crucial classes exist for managing an assembly’s resources. Here is a list of them:
- ResourceManager: Access and management software included within an assembly.
- ResourceWriter: A kind of software designed to record data about available resources somewhere else.
- ResourceReader: A kind of software installed inside of an assembly that crawls the Internet for relevant information.
6. How to Create an Assembly in .NET
Creating a .NET assembly is a breeze using the Visual Studio IDE. After finishing a project, you may generate an assembly by running the code through a compiler. The CLR’s(Common Language Runtime) behavior toward the assembly you produce depends on the type you choose when creating it. Assembly properties like version, culture, and strong-naming data can also be configured.
It’s worth noting that in .NET, code may be easily reused and versioned by building an assembly. Writing less duplicate code is possible when you create an assembly once and use it in different apps and projects. Furthermore, you may prevent incompatibilities between variants of the same assembly on the same computer by using strong naming and setting version data.
using System; using System.Resources; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; [assembly: AssemblyTitle("MyFirstAssembly")] [assembly: AssemblyDescription("My First Example assembly")] [assembly: AssemblyConfiguration("")] [assembly: AssemblyCompany("MyCompany")] [assembly: AssemblyTrademark("")] [assembly: ComVisible(false)] [assembly: AssemblyProduct("MyProduct")] [assembly: AssemblyCulture("")] [assembly: AssemblyCopyright("Copyright © MyCompany")] [assembly: Guid("YOUR-GUID-HERE")] [assembly: AssemblyVersion("1.0.0.0")] [assembly: AssemblyFileVersion("1.0.0.0")] [assembly: NeutralResourcesLanguage("en-US")] namespace MyFirstAssembly { public class HelloWorld { public void Hello() { Console.WriteLine(".NET Assembly!"); } } } |
Here, we’ll make a brand-new assembly and give it the name MyFirstAssembly. We provide details about the assembly, including its name, outline, variant, and culture. To further denote that this assembly is not meant for COM interop, we additionally set the ComVisible property to false. We conclude by defining a class named HelloWorld with just one function that sends an input to the console.
The generated assembly can then be referenced assemblies and instances of the classes it defines are used in other apps and projects. Make a new console application that references the MyAssembly, instantiate the HelloWorld instance, and then launch the Hello procedure.
7. How to Create a Single File Assembly in C#
Now, we’ll look at how to make a single-file assembly using C#. Both the assembly information and the MSIL code will be included in the resulting file assembly. To make a standalone assembly, type the following command:
csc /out: SingleFileAssembly.dll /target: library AssemblyExample.cs AssemblyTypeExample.cs |
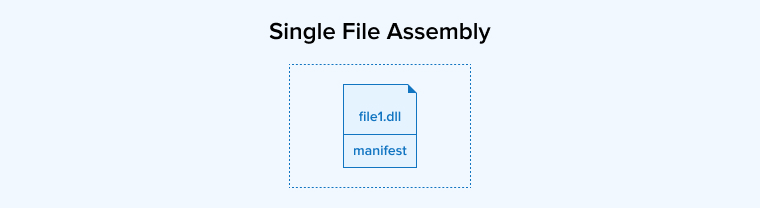
The preceding line generates an assembly consisting of a single file called SingleFileAssembly. Both the library assembly files and the output file name can be specified with the /out and /target arguments, respectively.
8. Features of .NET Assembly
The following are some of the common features of each given assembly:
- The meaning of .NET framework assemblies should be obvious. The assembly itself is the repository for all assembly-related data.
- Version dependencies are tracked in the assembly manifest.
- Assemblies can be loaded in a side-by-side fashion.
- The files that comprise an assembly may often be copied and pasted into a new location to set up the assembly.
- Assemblies can be put restricted or open to the public.
9. Conclusion
Developing with .NET requires a good grasp of assemblies. Assemblies are an integral feature of the .NET framework because they facilitate the sharing and reuse of code. You can build .NET apps that are safe, easy to maintain, and quick to deploy if you take the time to learn how assemblies function.
10. FAQs
What is Assembly and Metadata in .Net?
In .NET, assembly is a collection of resources that work together and create a logical unit of functionality. Whereas, metadata is known as the information that is stored in the assembly that specifies methods and types of the assembly.
What is the use of .NET Assembly?
Assembly is known as an essential part of the .NET framework. It offers versioning information and reusable code which enables easy updates and maintenance. Besides this, assemblies can also be used to package resources like configuration files, images, and sounds.
Where are .NET Assemblies located?
In the .NET framework, assemblies can be located in the application’s directory, subdirectory, or global assembly cache.
Comments
Leave a message...