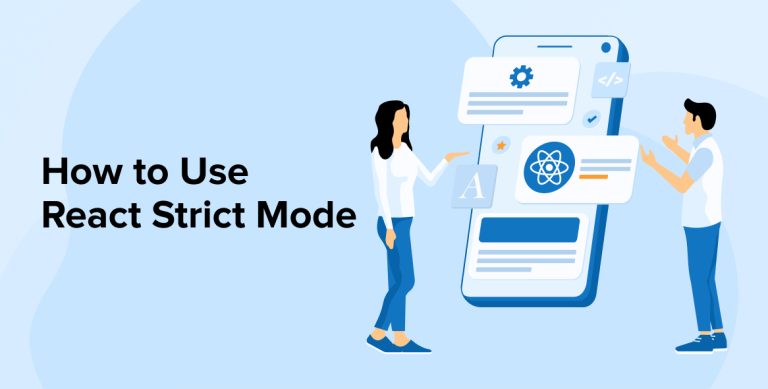
React is a technology that has been around for a long time and it has been used by app development companies to create unique applications. With time, this technology has come up with a lot of new techniques, and ways to handle UI issues and tools. In March 2022, React launched its new version, v18. With it came some changes in its architecture which mostly focused on behavioral changes of React StrictMode API, new React hooks, and shipping Concurrent Mode. In the React app development ecosystem, Strict Mode has been a feature for some time, but in v18 of React, this feature has become more efficient in finding early bugs. This helps in making the codebase more predictable for the developers.
To know more about strict mode and see why every React app development company uses it, let’s go through this blog.
1. What is StrictMode?
In the React app development ecosystem, StrictMode is known as a popular JavaScript feature that enables developers to create a solution that can run in a safe development mode even if there are potential issues detected. This also includes the creation of specific objects or variables that are in read-only mode. Strict Mode enables the development teams to prevent JavaScript functions like eval() and arguments from being utilized in creating applications. This JavaScript feature is mainly used by app development companies while creating data-driven applications and automating tasks. StrictMode helps companies streamline the installation and configuration process by making the processes easier.
To use strict mode just add "use strict" at the beginning of your JS file.
— Basharath (@wahVinci) December 27, 2022
A simple example ↓ pic.twitter.com/dvnMVtabsz
2. Why use React StrictMode?
For any JavaScript app developer, using Strict Mode is very easy. They can smoothly implement this feature and make projects of varied sizes and capacities. Besides this, some other reasons make it a perfect match for React development and they are:
- Strict Mode comes with an early warning feature that will make the developers aware of any non-strict practices or unsafe code well in advance.
- This React feature helps the development team to easily catch minute code errors and potential bugs that would be missed by developers.
- With this approach, developers can get easy access to security features for memory leaks and potential resources.
- React Strict Mode comes with the ability to make developers aware of the application’s state by focusing on their performance.
3. How does it Work?
Now, after learning about React Strict Mode and going through the reasons why it can be used in app development, we will see how one can make use of React StrictMode. React’s strict mode comes with some specific functions and tools that can help the developers find and identify potential errors in the application. Besides identifying performance-related issues, it also helps in finding unsafe use of setState(). Therefore, it is a must to implement Strict Mode in the development environment. Here’s how it works with your application:
- React’s StrictMode will run in the development mode which is possible after adding it to a component at the beginning of the application.
- Then it will start identifying software bugs and issues that are present in the application code and will notify the developers.
- With this React feature, developers can easily check potential problems like memory leaks in the code and warn the users.
To use this feature in your React application, developers must follow the below-given points:
3.1 Setup Your Development Environment
First of all, the React app developer will have to scaffold a new React project. For this, in the terminal, the developer will have to run the following command:
npx create-react-app react-strict-mode-demo-app |
Once the application is created and all the dependencies are automatically installed, the React developer will have to navigate the project directory and run the application. For this, the developer will have to run the below-given command in the terminal:
cd react-strict-mode-demo-app && npm start |
After running the above command, one will be able to see similar output below in the terminal:
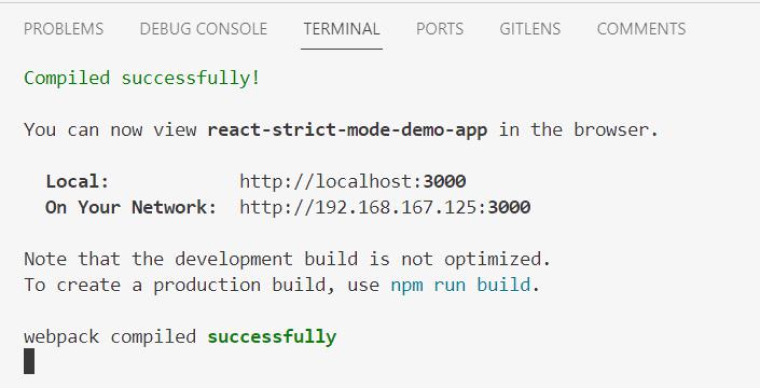
After that, the developer can look at the react-strict-mode tutorial in the browser.
Local: http://localhost:3000 On Your Network: http://192.168.167.1253000 |
Here, the development build is not optimized. To create a production build, the developer will have to use npm run build.
After that, the React application will start reloading on the development environment and the app will run on the localhost:3000 by default.
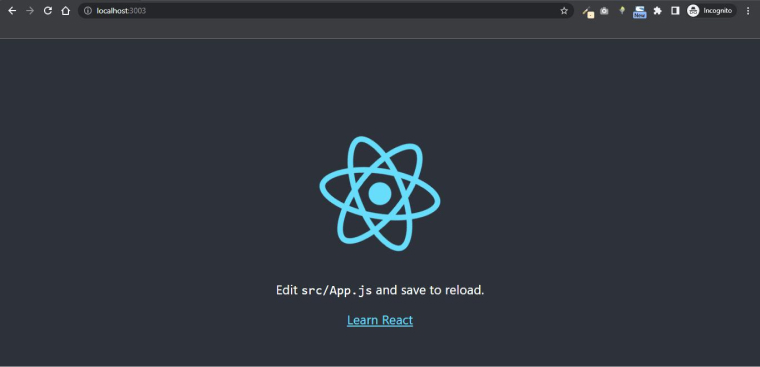
3.2 Getting Started with React Strict Mode
Now, we will start using React Strict Mode in the application. To do so, the developer will have to wrap the child components of the code with either <React.StrictMode> or <StrictMode>. After this, one must import StrictMode from React at the top of the component. Here is how it can be done:
import React from 'react'; import Home from './home.js'; import AboutUs from './about-us.js'; function App() { return ( <div> <Header /> <React.StrictMode> <div> <Home /> <AboutUs /> </div> </React.StrictMode> <Footer /> </div> ); } |
or
import React, { StrictMode } from 'react'; import Home from './home.js'; import AboutUs from './about-us.js';function App() { return ( <div> <Header /> <StrictMode> <div> <Home /> <AboutUs /> </div> </StrictMode> <Footer /> </div> ); } |
4. Unsafe Lifecycle Warning
Now, after seeing how React Strict Mode can be implemented. We will have a look at its unsafe lifecycle warnings. In React, class-based lifecycle methods have seen many changes in the API. Some methods were used widely in previous times but are now officially deprecated to support the modern-age APIs. For this, Strict Mode in React warns developers about deprecated APIs like componentWillUpdate, componentWillMount, and componentWillReceiveProps, then it will be considered an unsafe app development methodology. To make developers aware of it, React has added a UNSAFE prefix to these APIs. An example of this is described below:
- UNSAFE_componentWillUpdate
- UNSAFE_componentWillMount
- UNSAFE_componentWillReceiveProps
This specifies that the React Strict Mode is smart enough to warn the React app developers when any third-party package is being used along with these deprecated APIs.
5. Deprecated FindDOMNode Warning
Now, we will have a look at the deprecated findDOMNode warning. Here this warning is known as a class-based API that developers can use to target an element in the DOM tree. Here is an example of how it can be done from any component:
import React from 'react'; class Layout extends React.Component{ componentDidMount() { const element= ReactDOM.findDOMNode(this); console.log(element); } render () { return <Navigation>{this.props.children}</Navigation>; } } |
The above code might look fine in the developer’s eye, but it can cause problems with React’s abstraction principle. The reason behind this is that the parent component of the program must ensure that the child components are rendering the correct DOM nodes and are reaching down smoothly. But one of the biggest disadvantages of findDOMNode is that it only calls the API one time, which means that if the node element gets changed due to some state update in the application, those changes won’t be reflected and updated in the application.
This is why React Strict Mode warns developers of findDOMNode.
6. Legacy String Ref API Warning
React also warns about the warning called legacy string ref API. Any developer who has worked with React will know that when the class-based architecture was the de facto way of developing application components, they might have used string ref API as shown in the code below:
import React from 'react'; class CustomForm extends React.Component { render() { return <input onClick={() => this.handleInputChange()} ref='input' />; } handleInputChange() { console.log(this.refs.input.value); } } |
This API is known as a legacy for many different reasons:
- The string ref API can make it difficult for the developers to read and do static analysis of the application code with a type checker.
- In the code, when a wrapped component can’t find out if the child component already consists of a ref, then this issue can only be resolved using a callback ref pattern.
The React StrictMode warns the React app developers to use a modern createRef API or a callback pattern.
7. Detecting Legacy Context API
Now, Let’s have a look at the legacy context API which is similar to refs API. The React Strict Mode also warns against the usage of legacy context API. The reason for this is that this API will be removed from future releases. So, instead of this API, it is suggested that one uses a modern context API that uses the provider-consumer pattern as soon in the below code:
const AppThemeContext = React.createContext('dark') // consume it here <AppThemeContext.Provider value={data}> {children} </AppThemeContext.Provider> |
The above use of modern context API is a recommended way of handling app state context.
8. Conclusion
As seen in this blog, React Strict Mode is used to find potential issues early and write better code. It helps developers improve their development experience by offering warnings about deprecated APIs, identifying unsafe lifecycle methods, finding potential side effects, and promoting best practices for efficient React app development.
FAQs
1. Are there any limitations to using StrictMode in React?
React StrictMode can only be applied in the app development process and it does not affect the production behavior of the application. This is considered the biggest limitation of using Strict Mode in React. Besides this, some issues might not be detected or displayed by Strict Mode.
2. What types of warnings does StrictMode miss to specify?
Some of the warnings that Strict Mode misses to detect are logic errors or memory leaks within a React app.
3. What is React Strict Mode? Is it optional?
StrictMode is known as a streamlining command that can be used by React app developers to create highly efficient programs. It is considered an optional feature in React as it enables the developers to identify potential errors.
4. What is the use of Strict Mode?
Strict Mode in JavaScript is used to fix mistakes that affect engine optimization and to prohibit syntax that is to be specified in future ECMAScript versions. It also helps in enhancing code security.
Comments
Leave a message...