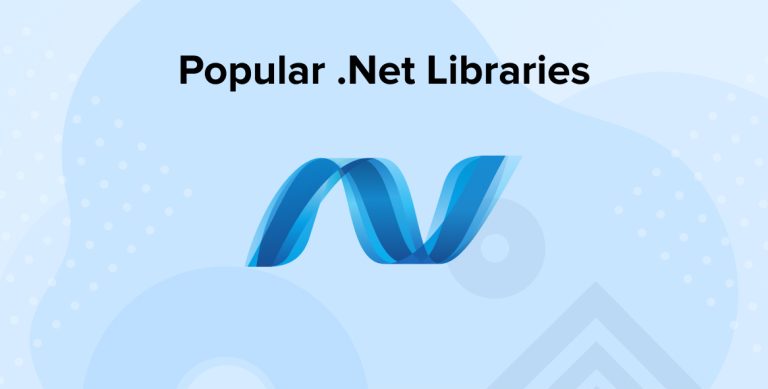
One of the crucial elements of any technology is the ability to select and learn the finest .Net Libraries that enable improving the development activity. The fast expansion of the online market parallels the growth of the digital population.There seems to be a lot of activity, and the level of competition between businesses and developers appears to be rising.
One such technology that aids in providing services that are both user- and customer-friendly is the .NET framework. Microsoft’s. NET core framework has been an enormous success. It allows .NET developers to create robust, efficient, and feature-rich websites, mobile and online .NET applications.
As the .NET development company continues to expand rapidly, it is making more libraries available to meet the varying needs of programmers. Therefore, below enlisted are the top essential .NET libraries for .Net developers to employ while creating .NET apps, to aid in your selection and comprehension of the many portable class libraries considered vital for .NET Core integration.
1. Popular .Net Libraries
1.1 AutoMapper
When developers use AutoMapper to map the properties of two distinct object types, they have demonstrated its effectiveness as an object-to-object mapper library. AutoMapper facilitates straightforward type setup and mapping testing. Object-object mapping methods perform the conversion from one object type to another. AutoMapper provides appealing standards to avoid the bother of manually mapping one data type to another. Because problems in one layer often clash with those in another, object-object mapping generates partitioned models in which problems at a given layer can only impact types at that level.
Benefits of AutoMapper:
- Easier Mapping: If you’re using AutoMapper, you can check the mapping with unit tests in one location, saving time and effort.
- Clean Coding: Data transmission between distinct objects requires only fewer lines. Reduced development time directly results from simpler code since less effort is needed to map objects.
- Great Maintainability: The identical mapping between the two items is centralized in one location, which makes it easier to maintain.
Let’s See How to Use AutoMapper Library:
Step 1: Here are the Employee entity and Employee view model classes.
public class Employee { public Guid Id { get; set; } = Guid.NewGuid(); public string Name { get; set; } public string Email (get; set; } public DateTime CreatedDate { get; set; } = DateTime.Now; } |
public class EmployeeViewModel { [Required] public string Name { get; set; } [Required] public string Email { get; set; } } |
Step 2: In order to implement AutoMapper, first we should download a NuGet Packages to our project:
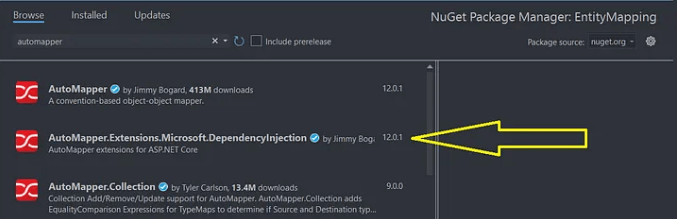
Step 3: Create a mapping profile that specifies how to map the properties between the Employee entity and the EmployeeViewModel.
public class MappingProfile: Profile { public MappingProfile() { CreateMap< EmployeeViewModel, Employee>() .ForMember(dest=> dest.Name, opt=> opt.MapFrom(src => src.Name)) .ForMember(dest=> dest.Email, opt=> opt.MapFrom(src => src.Email)); } } |
Step 4: After creating the proper mapping profile class, next step is to register AutoMapper as a Service in Program.cs file.
// Add services to the container, builder.Services.AddAutoflapper(typeof(Program).Assembly); builder.Services.AddControllersWithViews(); var app = builder.Build(); |
Step 5: We can map Employee entity with EmployeeViewModel in the controller methods.
using AutoMapper: using Microsoft.AspNetCore.Mvc; using PracticeAPP.Models; using PracticeAPP.Models.Entities; using PracticeAPP.Models.ViewModels; using System.Diagnostics; namespace PracticeAPP.Controllers { public class HomeController: Controller { private readonly ILoggerlogger<HomeController>logger; private readonly IMapper_mapper; public HomeController(ILogger<HomeController>logger, IMapper mapper) { _logger= logger; _паppег= mapper; } public IActionResult Index() { return View(); } public IActionResult Register(EmployeeViewModel model) if (!ModelState.IsValid) return BadRequest(ModelState); var employee= _mapper.Map(model); return View(employee); } |
1.2 Polly
Polly is a .NET library for addressing transitory faults and retries. It also provides programmers with an articulated and thread-safe way to define policies like Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback.
Furthermore, with just a few lines of code, Polly can protect your resources, prevent you from making queries to broken services, redo unsuccessful requests, previous cache results, terminate long inquiries, and, in the worst-case scenario, provide a default response. It’s also thread-safe and works with both synchronous and asynchronous calls.
Polly can enable the .NET ecosystem to use try..catch and when added in a modern distributed environment, it can help in making calls to various databases, processes, and more.
Consider something like Polly in the .NET ecosystem that uses try..catch to provide Retry, Circuit Breaker, etc. . Add in modern distributed environments where i make a number of calls to other processes, databases, HTTP, middlware etc.
— Ian Cooper (@ICooper) September 4, 2020
Your Gateway probably wants a try block
Benefits of Polly:
- Effective Policies: Polly can handle transitory faults and gives resilience. Retry, Circuit Breaker, Bulkhead Isolation, Timeout, and Fallback are all Polly policies that can be used to realize these capabilities.
- Thread-safety: None of Polly’s policies poses a threat to thread safety. Policies may be securely reused across numerous call sites and run in parallel across many threads.
Let’s See How to Use Polly Library:
Polly is a .NET resilience strategies and transient fault handling library, allowing developers to express resilience policies such as Retry policy, Circuit Breakers, Timeout, Bulkhead Isolation policy, and Fallback. Polly targets ASP.NET Core, making it a vital tool for . NET Core resilience.
1.3 Swashbuckle
Use this .NET library to create polished API docs. You may use the Swagger UI to inspect and experiment with API activities in Swashbuckle as well. Its primary function is to produce a Swagger specification file for your .Net-based project. This package comprises a comprehensive set of tools for building .NET APIs. It may surprise you to learn that we used C# instead of JavaScript to write all of this.
Add Swagger to your .NET Core Web API https://t.co/yAoqxmD676 #dotNet #dotNetCore #Swagger #Swashbuckle pic.twitter.com/GGoBlem0LA
— Carlos Mendible (@cmendibl3) July 4, 2016
Benefits of Swashbuckle
- Documentation: It is utilized to quickly and easily provide browser-based, beautiful API documentation by generating an OpenAPI definition from controllers, routes, and models.
- Authentication Mechanism: Multiple authentication mechanisms are supported by Swashbuckle, such as OAuth2, API keys, and JWT (JSON Web Tokens).
There are three main components to Swashbuckle:
- Swashbuckle.AspNetCore.Swagger: a Swagger object model and middleware to expose SwaggerDocument objects as JSON endpoints.
- Swashbuckle.AspNetCore.SwaggerGen: a Swagger generator that builds SwaggerDocument objects directly from your routes, controllers, and models. It’s typically combined with the Swagger endpoint middleware to automatically expose Swagger JSON.
- Swashbuckle.AspNetCore.SwaggerUI: an embedded version of the Swagger UI tool. It interprets Swagger JSON to build a rich, customizable experience for describing the web API functionality. It includes built-in test harnesses for the public methods.
Let’s See How to Use Swashbuckle Library:
Step 1: Install the Swashbuckle.AspNetCore.
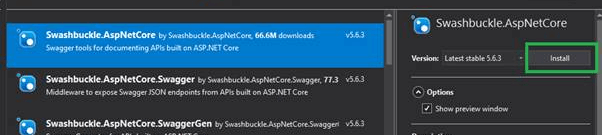
Step 2: Now, please Configure the program.cs file for swagger and the application you can able to check the API via Swagger.
//Add AddSwaggerGen builder.Services.AddSwaggerGen(); builder.Services.AddControllersWithViews(); var app = builder.Build(); //Configure the HTTP request pipeline. if (!app.Environment.IsDevelopment()) { app.UseExceptionHandler("/Home/Error"); } //Config Swagger for Dev if (app.Environment.IsDevelopment()) { app.UseSwagger(); app.UseSwaggerUI(); } |
1.4 CacheManger
To support the cache providers in .NET implementation enhanced functionalities, CacheManager was built as an open-source .NET Network library in the C# programming language.
With the .NET CacheManager library, developers can simplify their work and handle even the most intricate cache situations with only a few lines of code.
Benefits of CacheManger:
- Seamless Coding: Because of the potential for concurrency, changing a value in a distributed setting can be tricky. CacheManager abstracts away the complexity, allowing you to do so with a single line of code.
- Sync Mechanism: You can keep the local instances in sync while utilizing many layers in combination with a distributed cache layer and having numerous instances of your app running.
- Cross-platform Compatible: CacheManager now includes cross-platform compatibility and has been tested on Windows, Linux, and iOS thanks to the new .NET Core / ASP.NET Core project structure and libraries.
Let’s See How to Use CacheManger Library:
Step 1: Add Configuration for CatcheManager in Program.cs file.
services.AddLogging(c => c.AddConsole().AddDebug().AddConfiguration(Configuration)); services.AddCacheManagerConfiguration(Configuration,cfg=>cfg.WithMicrosoftLogging(services)); services.AddCacheManager<int>(Configuration, configure: builder => builder. WithJsonSerializer()); services.AddCacheManager<DateTime>(inline => inline.WithDictionaryHandle()); services.AddCacheManager(); |
Step 2: Now, We will use CatcheManager in Values Controller.
[Route("api/[controller]")] public class ValuesController: Controller { private readonly ICacheManager _cache; public ValuesController(ICacheManager valuesCache, ICacheManager intCache, ICacheManager dates) { _cache = valuesCache; dates.Add("now", DateTime.UtcNow); intCache.Add("count", 1); } // DELETE api/values/key [HttpDelete("{key}")] public IActionResult Delete(string key) { if (_cache.Remove(key)) { return Ok(); } return NotFound(); } // GET api/values/key [HttpGet("{key}")] public IActionResult Get(string key) { var value = _cache.GetCacheItem(key); if (value == null) { return NotFound(); } return Json(value.Value); } |
// POST api/values/key [HttpPost( “{key}")] Public IActionResult Post(string key, [FromBody]string value) { if (_cache.Add(key, value)) { return Ok(); } return BadRequest("Item already exists."); } // PUT api/values/key [HttpPut("{key}")] public IActionResult Put(string key, [FromBody]string value) { if (_cache. AddOrUpdate(key, value, (v) => value) != null) { return Ok(); } return NotFound(); } } } |
1.5 Dapper
To facilitate communication between the programs and the database, you can make use of Dapper, an Object-Relational Mapper (ORM) that is more precisely. Micro ORM that supports SQL server. Dapper allows you to write SQL statements in the same format as SQL Server. Dapper’s speed is unparalleled since it does not alter SQL queries done in .NET. It can execute methods either synchronously or asynchronously.
Benefits of Dapper:
- Size: It is lightweight, easy to access and operate.
- Security: Dapper is immune to SQL Injection so that you can execute parameterized queries freely. Assistance for many database providers is another important aspect.
- Database Compatibility: Dapper is compatible with a wide range of database services. It’s an extension to ADO.NET’s IDbConnection that adds convenient new methods for working with your database.
Let’s See How to Use Dapper Library:
Step 1: Let’s start by creating a new Entities folder with two classes inside
public class Employee { public Guid Id { get; set; } Guid. NewGuid(); public string Name { get; set; } public string Email { get; set; } public DateTline CreatedDate { get; set; } = DateTime.Now; } public class Company { public int Id { get; set; } public string Name { get; set; } public string Address { get; set; } public string Country { get; set; } public List Employees { get; set; } = new List(); } |
Step 2: Now, we are going to create a new Context folder and a new DapperContext class under it:
public class DapperContext { private readonly IConfiguration _configuration; private readonly string _connectionString; public DapperContext(IConfiguration configuration) { _configuration = configuration; _connectionString =_ configuration.GetConnectionString("SqlConnection"); } public IDbConnection CreateConnection() => new SqlConnection(_connectionString); } |
Step 3: We have to do the registration in the Program class:
builder.Services.AddSingleton(); builder.Services.AddControllerswithViews(); |
Step 4: Now We have to create Repository Interfaces and Classes and Then, let’s implement this method in the class:
public interface ICompanyRepository { public Task<IEnumerable<Company>> GetCompanies(); } |
using Dapper; using PracticeAPP.Context; using PracticeAPP.Contracts; using PracticeAPP.Models.Entities; namespace PracticeAPP.Repository { public class CompanyRepository: ICompanyRepository { private readonly DapperContext _context; public CompanyRepository(DapperContext context) { _context = context; } public async Task<IEnumerable<Company>> GetCompanies() { var query= "SELECT * FROM Companies"; using (var connection = _context.CreateConnection()) { var companies = await connection.QueryAsync<Company>(query); return companies. Tolist(); } } } } |
1.6 Ocelot
Ocelot is an asp.net core library that is a bridge for .NET APIs. The target audience mainly consists of .NET developers who need a hub for their decentralized service or microservice architecture. Nevertheless, it will run on any system where ASP.NET Core is available and work with any service that understands HTTP.
It tricks the HTTP Request entity into the state determined by its parameters until it runs into a request builder middleware. An HTTP Request Message is constructed there and then utilized to make contact with a service. The Ocelot pipeline culminates in the submission of the request by the middleware. After that point, no more calls to middleware are made. As requests go upstream up the Ocelot pipeline, they are accompanied by the answer from the downstream service.
It would be best if you examine both, and see what works. I think Ocelot is more advanced and with more features. But YARP integrates really well with the existing .NET ecosystem.
— Milan Jovanović (@mjovanovictech) July 7, 2023
This is how the Ocelot API Gateway Works in our project.
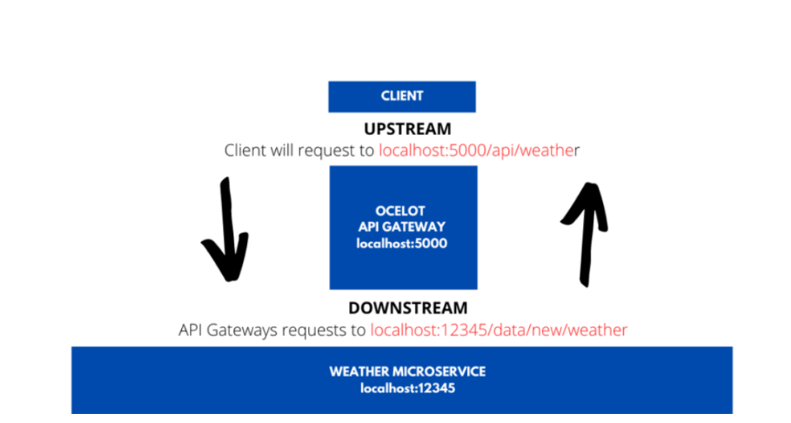
Benefits of Ocelot:
- Usability: Routing, request aggregation, web sockets, rate limiting, authentication, authorization, caching, load balancing, configuration, etc. are some of the many uses for this net core module.
Let’s See How to Use Ocelot Library:
Step 1: You should add Ocelot to the service container by calling the AddOcelot method in the ConfigureServices method of the Startup class as shown below:
public void ConfigureServices(IServiceCollection services) { Action<ConfigurationBuilderCachePart> settings = (x) => { x.WithMicrosoftLogging(log => { log.AddConsole(LogLevel.Debug); }).WithDictionaryHandle(); }; services.AddOcelot(Configuration, settings); } public async void Configure(IApplicationBuilder app, IHostingEnvironment env ) { await app.UseOcelot(); } |
Run the projects.
Now make sure that you’ve made all projects as startup projects. To do this, follow these steps:
- In the Solution Explorer window, right-click on the solution file.
- Click “Properties”.
- In the “Property Pages” window, select the “Multiple startup projects” radio button
- Click Ok.
Press the F5 key to run the application.
1.7 Autofac
For .NET applications, Autofac provides an IoC container. It keeps programs flexible even as they expand in size and complexity by controlling inter-class relationships. In order to achieve this goal, developers can use typical .NET classes as building blocks.
Benefits of Autofac:
- Flexibility: Autofac dot net library controls inter-class dependencies to keep programs flexible even as their size and complexity increase.
- Community support: Most ASP.NET developers choose Autofac as their DI/IoC container of choice since it is compatible with .NET Core architecture as well without any issues.
Let’s See How to Use Autofac Library:
Step 1: Install Autofac and Autofac.Extensions.DependencyInjection nuget package
Step 2: The code in program class will change to use Autofac as the DI container.
builder.Host.UseServiceProviderFactory(new AutofacServiceProviderFactory()); |
Step 3: We will create class and interface with method return static string.
using PracticeAPP.Contracts; namespace PracticeAPP.Repository { public class AutofacRepository: IAutofacRepository { public string getStringAutofac() { return "Hello Autofac Data"; } } } namespace PracticeAPP.Contracts { public interface IAutofacRepository { public string getStringAutofac(); } } |
Step 4: Finally, I will update the Controller to use the interface to return the constant text “Hello Autofac Data” as a return to the GetStringAutofac method.
using Microsoft.AspNetCore.Mvc; using PracticeAPP.Contracts; namespace PracticeAPP.Controllers { public class AutofacController: Controller { private readonly IAutofacRepository _autofacRepository; public AutofacController(IAutofacRepository autofacRepository) { this._autofacRepository=autofacRepository; } // GET api/values [HttpGet] public string GetStringAutofac() { return _autofacRepository.getStringAutofac(); } } } |
1.8 MediatR
An entity that models the dynamics between many parties is described by the mediator pattern in the field of software development. This pattern is classified as a behavioural pattern because it has the ability to alter the actions of the code while it is being executed.
MadiateR is a simple implementation of a mediator in .NET. Request/response, instructions, inquiries, notifications, and instances. In this library both synchronous and asynchronous are all made possible by the smart dispatching of C# generic variance.
MediatR can be thought of as an “in-progress” version of Mediator that facilitates the creation of CQRS systems. Among the many helpful .Net libraries, stands out.
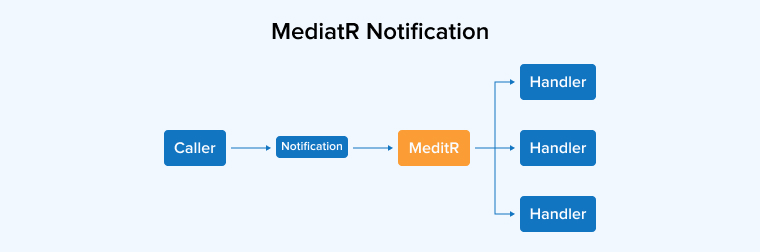
Benefits of MediatR:
- Reduced Dependencies: The direct dependence on numerous objects can be reduced by using the MediatR pattern to make them cooperative.
- Communication: It serves as the primary means of interaction between your user interface and your data storage system. Classes provided by MediatR in .NET Core facilitate efficient, freely linked communication between numerous objects.
Let’s See How to Use Autofac Library:
Step1: Create a new web API project.
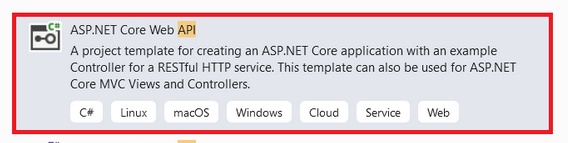
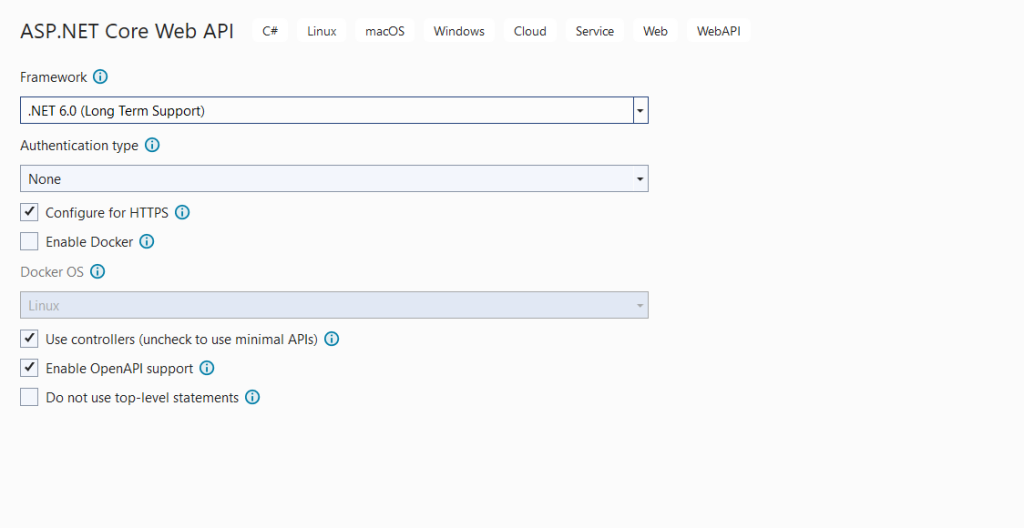
Step 2: Create a folder with these three classes.
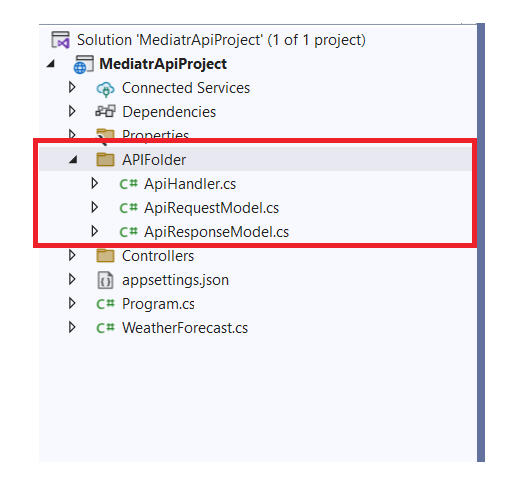
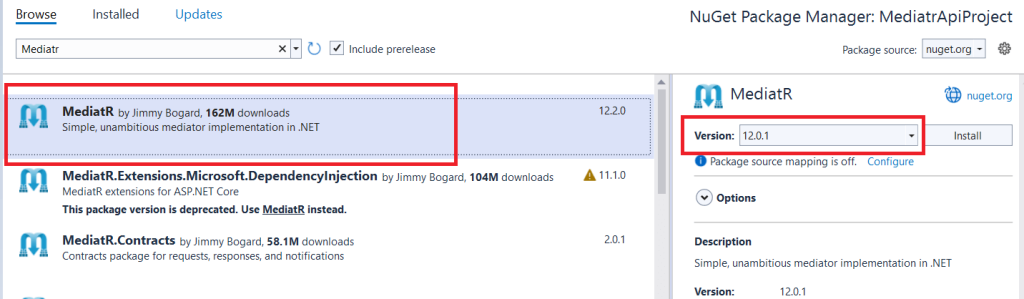
Step 3: ApiRequestModel: This class represents a request for API.
using MediatR; namespace MediatrApiProject.APIFolder { public class ApiRequestModel: IRequest { public int Number { get; set; } } } |
— IRequest<> It represents a request with a response.
Step 4: ApiResponseModel: This class represents a response of API.
namespace MediatrApiProject.APIFolder { public class ApiResponseModel { public string Response { get; set; } = string.Empty; } } |
Step 5: ApiHandler: And this class keeps the logical part
using MediatR; namespace MediatrApiProject.APIFolder { public class ApiHandler: IRequestHandler public async Task Handle(ApiRequestModel apiRequest, CancellationToken cancellationToken) { var response = $"Number :- {apiRequest.Number}"; return new ApiResponseModel { Response=response }; } } } |
using MediatR; using Microsoft.AspNetCore.Mvc; namespace MediatrApiProject.Controllers { [ApiController] [Route("api/[controller]")] public class APIControllerBase : ControllerBase { private ISender _mediator; protected ISender Mediator => mediator ??= HttpContext. RequestServices.GetService<ISender>(); } } |
using MediatrApiProject.APIFolder; using Microsoft.AspNetCore.Mvc; namespace MediatrApiProject.Controllers { public class HomeController: APIControllerBase { public HomeController() { } [HttpGet(nameof(ApiController))] public async Task<ActionResult<ApiResponseModel>> ApiController(int number) { try { var request = new ApiRequestModel { Number = number }; return await Mediator.Send(request); } catch (Exception ex) { throw; } } } } |
Step 6: Test this API using swagger.
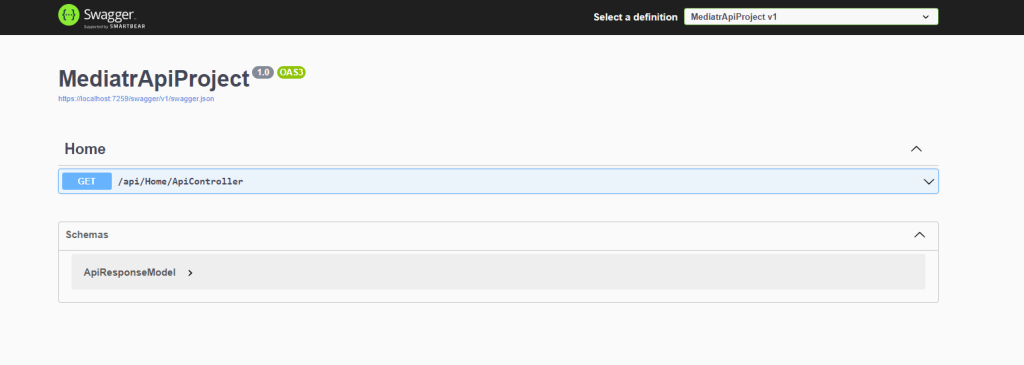
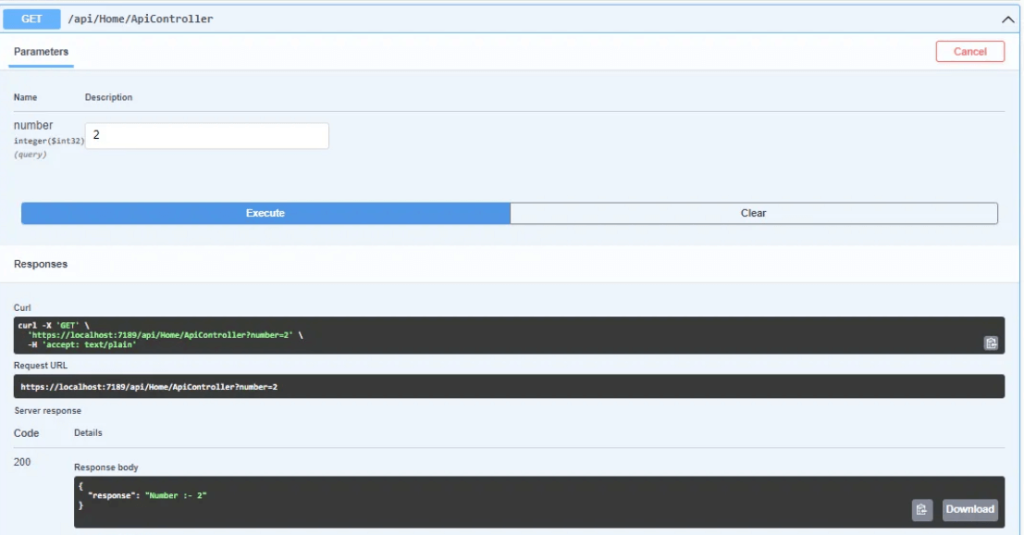
1.9 Fluent Validation
Fluent Validation is a .NET framework class library for creating validation rules that are highly typed. Fluent Validation is an excellent substitute of data annotations for model validation. It provides for a much more substantial segregation of issues, enhances control over validation rules, and makes them easy to comprehend and test. The Lambda expression is used to check the validity. When you need to create complicated validation for the user’s data, Fluent Validation comes in handy.
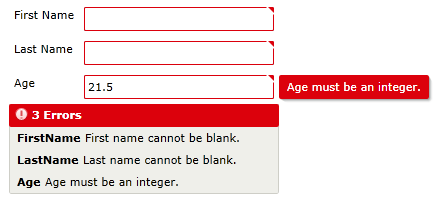
Benefits of Fluent Validation:
- Open-source: It’s a free and open-source library for making and maintaining clear validations. It also works with third-party models, which you cannot modify. This provides much-needed clarity and readability to the model classes.
- Code Quality: By eliminating the requirement for data annotations during the development of a dedicated public class for testing your models, FluentValidation helps you produce code that is clearer, more manageable, and of higher quality.
Let’s See How to Use Fluent Validation Library:
Step 1: Create Employee class:
public class Employee { public Guid Id {get; set; }= Guid.NewGuid(); public string Name { get; set; } public string Email { get; set; } public DateTime CreatedDate { get; set; } DateTime.Now; } |
Step 2: Validated using a EmployeeDtoValidator. These classes are defined as follows:
public class EmployeeDtoValidator: AbstractValidator<Employee> { public EmployeeDtoValidator() { RuleSet("name", () => { RuleFor(x => x.Name). NotNull().WithMessage("name could not be null"); }); RuleSet("email", () => { RuleFor(x => x.Email).NotNull().WithMessage("email could not be null"); }); } } |
Step 3: Configure validator class in program file.
builder.Services.AddScoped<IValidator<Employee>, EmployeeDtoValidator>(); builder.Services.AddValidatorsFromAssemblyContaining<EmployeeDtoValidator>(); |
Step 4: With the manual validation approach, you’ll inject the validator into your controller and invoke it against the model.
For example, you might have a controller that looks like this
public class EmployeeController: Controller { private IValidator _validator; public EmployeeController(IValidator validator) { //Inject our validator and also a DB context for storing our Employee object. _validator = validator; } public ActionResult Create() { return View(); } [HttpPost] public async Task Create(Employee employee) { var result= await _validator.ValidateAsync(employee); if (!result.IsValid) { //re-render the view when validation failed. return View("Create", employee); } TempData["notice"] = "Employee successfully created"; return RedirectToAction("Index"); } } } |
1.10 SignalR
A key goal of today’s app development is to give users live information. Whether you’re making a game, a dashboard, or anything else, you may benefit from real-time features. The ability of your server-side code to rapidly transmit material to related clients talks about real-time capability. In this case, we require SignalR in .Net Core. It also streamlines the process significantly. Like a chat room, SignalR automatically manages connections and allows you to send messages to all users at once.
You can integrate SignalR hubs, written in C#, into your .NET Core project alongside your pages and APIs. In addition, the fundamental programming approach naturally incorporates additional NET advanced features like dependency injection, authentication, authorization, and extensibility.
SignalR is one of the best libraries to build real-time apps with .NET.
— Milan Jovanović (@mjovanovictech) July 8, 2023
It allows server-side code to send asynchronous messages to connected clients.
Here are a few good use cases for SignalR:
– Notifications
– Chat/messaging
– Real-time data streaminghttps://t.co/o0AbOLa619
SignalR is a library that can be used to develop real-time web applications in ASP.NET Core. SignalR contains an API that allows server-side code to send messages to connected client browsers.
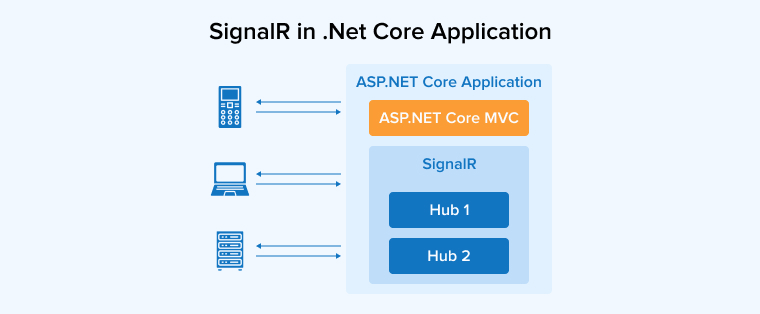
Benefits of SignalR:
- Real-time Functionality: SignalR is a free and open-source library that makes it easier to implement real-time features on the web. With the help of server-side programming, materials can be transmitted to clients in real-time thanks to real-time web capability.
- Networking: Able to broadcast the message to all clients in the network at once. It facilitates communication with clients both personally and in groups as well as it is also able to accommodate more users.
Let’s See How to Use SignalR Library:
Step 1: Install SignalR NuGet package: Integrate the SignalR library into your project to enable real-time communication.
Step 2: Register services and CORS policy
builder.Services.AddSignalR(); builder.Services.AddCors(options => { options.AddPolicy("CORSPolicy", builder=> builder.AllowAnyMethod().AllowAnyHeader().AllowCredentials().SetIsOriginAllowed((hosts) => true)); }}; |
Step 3: Define IMessageHubClient interface: Create an interface inside the “Hub” folder, outlining the methods for sending offers to users.
public interface IMessageHubClient { Task SendOffersToUser(List message); } |
Implement MessageHubClient class: Develop the actual SignalR hub that extends the IMessageHubClient interface. This class manages to send offers to clients.
public class MessageHubClient: Hub { public async Task SendOffersToUser(List message) { await Clients.All.SendOffersToUser(message); } } |
Step 4: Design a controller responsible for handling offers. Inject the IHubContext to enable sending messages via SignalR.
public class OfferController: Controller { private IHubContext messageHub; public OfferController(IHubContext_messageHub) { messageHub = messageHub; } [HttpPost] [Route("electronicoffers")] public string Get() { List offers = new List(); offers.Add("28% Off on IPhone 12"); offers.Add("15% Off on HP Pavillion"); offers.Add("25% Off on Samsung Smart TV"); messageHub.Clients.All.SendOffersToUser(offers); return "Offers sent successfully to all users!"; } } |
1.11 MailKit
MailKit is one of the top Net standard libraries. It is the pinnacle of MimeKit, this .net core library used for e-mail clients that work cross-platform. The goal of this work is to provide robust, feature-rich, and RFC-compliant SMTP, POP3, and IMAP client network implementations.
New #EnCase #EnScript sample – sending email messages using the .NET MailKit library.https://t.co/ATeE29Xz1E#DFIR #InfoSec #CaseAutomation #CustomWorkflows
— Simon Key (@SimonDCKey) September 15, 2021
@OpenTextSecure pic.twitter.com/FcYBomWq5v
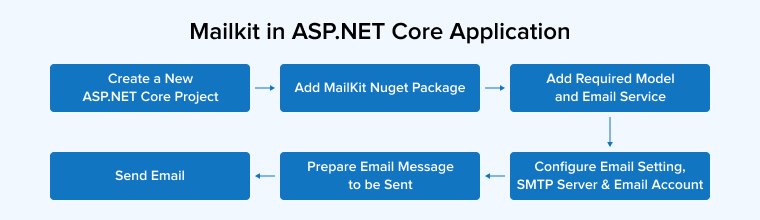
Benefits of MailKit:
- Security: Simple Authentication and Security Layer (SASL) Authentication.
- Proxy Support: Proxy support for SOCKS4/4a, SOCKS, and HTTP.
Let’s See How to Use MailKit Library:
Step 1: To send an email we need to provide some SMTP server details along with the account we would like to send emails from. The application should not hardcode these details because they might change over time and require updates. It also makes it easier to maintain for other developers. Below is the implementation in appsettings.json.
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft.AspNetCore": "Warning" } }, "AllowedHosts": "*", "MailSettings": { "DisplayName": "Developer Test", "From": "[email protected]", "Host": "smtp.test.email", "Password": "upPftsKAqgcFKKmXBW", "Port": 587, "UserName": "[email protected]", "UseSSL": false, "UseStartTls": true } |
Step 2: Create MailSettings object file like below.
public class MailSettings { public string? DisplayName { get; set; } public string? From { get; set; } public string? UserName { get; set; } public string? Password { get; set; } public string? Host { get; set; } public int Port { get; set; } public bool UseSSL { get; set; } public string UseStartTls (get; set; } } |
Step 3: Create a new file named MailData.cs with the following properties inside.
public class MailData { // Receiver public List To { get; } public List Bcc { get; } public List Cc { get; } // Sender public string? From { get; } public string? DisplayName { get; } public string? ReplyTo ( get; } public string? ReplyToName { get; } // Content public string Subject { get; } public string? Body { get; } public MailData(List to, string subject, string? body = null, string? from = null, string? displayName = null, string? replyTo = null, string? replyToName = null, List? bcc = null, List? cc = null) { // Receiver To = to; Bcc=bcc?? new List(); Cc = cc ?? new List(); // Sender From = from; DisplayName = displayName; ReplyToName = replyToName; ReplyTo = replyTo; // Content Subject = subject; Body = body; } } |
Step 4: Create a new folder named Services at the root of your project along with two new files inside named ImailService.cs and MailService.cs.
public interface IMailService { Task SendAsync(MailData mailData, CancellationToken ct); } |
public class MailService: IMailService { private readonly MailSettings _settings; public MailService(IOptions<MailSettings> settings) { _settings = settings.Value; } public async Task<bool> SendAsync(MailData mailData, CancellationToken ct = default) { try { // Initialize a new instance of the Minekit. MinaMessage class var nail = new MineMessage(); #region Sender / Receiver // sender mail.From.Add(new MailboxAddress(_settings.DisplayName, mailData. From ?? _settings From)); mail.Sender = new MailboxAddress(mailData.DisplayName ?? _settings.DisplayName, mailData .From ?? _settings.From); // Receiver foreach (string mailAddress in mailData.To) mail.To.Add(MailboxAddress, Parse(mailAddress)); // Set Reply to if specified in mail data if(!string.IsNullOrEmpty(mailData.ReplyTo) mail. ReplyTo.Add(new MailboxAddress(mailData.ReplyToNane, mailData.ReplyTo)); // BCC // Check if a BCC was supplied in the request if (mailData.Bcc != null) { // get only addresses where value is not null or with whitespace. x value of address foreach (string mailAddress in mailData.Bcc. Where(x=>!string.IsNullOrWhiteSpace(x))) mail.Bcc.Add(MailboxAddress.Parse(mailAddress.Trim())); } // Check if a CC address was supplied in the request if (mailData.Cc != null) { foreach (string mailAddress in mailData.Cc.Where(x=> !string.IsNullOrWhiteSpace(x))) mail.Cc.Add(MailboxAddress.Parse(mailAddress.Trim())); } #endregion |
#region Content // Add Content to Mine Message var body=new BodyBuilder(); mail.Subject mailData.Subject; body.HtmlBody = mailData.Body; mail. Body = body.ToMessageBody(); #endregion #regian Send Mail using var smtp = new SmtpClient(); if (_settings.UseSSL) { await smtp.ConnectAsync(_settings.Host, _settings.Port, SecureSocketOptions.SsLOnConnect, ct); else if (_settings.UseStartTls) { await smtp.ConnectAsync(_settings.Host, _settings.Port, SecureSocketOptions.StartTls, ct); } await smtp.AuthenticateAsync(_settings. UserNane, _settings.Password, ct); await smtp. SendAsync(mail, ct); await smtp.DisconnectAsync(true, ct); #endregion return true; } catch (Exception) { return false; } } } |
Step 5: Configure MailSettings and MailService in the program.cs
builder.Services.Configure(builder.Configuration.GetSection(nameof(MailSettings))); builder.Services.AddTransient(); |
Step 6: Add a new MailController to send emails
public class MailController: Controller { private readonly MailService _mail; public MailController(IMailService mail) { _mail = mail; } [HttpPost("sendmail")] public async Task SendMailAsync(MailData mailData) { bool result = await _mail.SendAsync(mailData, new CancellationToken()); if (result) { return StatusCode(StatusCodes.Status2000K, "Mail has successfully been sent."); else { return StatusCode(StatusCodes.Status500Internal ServerError, "An error occurred. The Mail could not be sent."); } } } |
1.12 Autocomplete
Autocomplete is a ASP.NET core library that allows desktop, online, and cloud applications to automatically complete features like text fields and domains.
Benefits of Autocomplete:
- Autofill search: The autofill search option in the ASP.NET Core, AutoComplete control allows the user to quickly and simply search for objects by completing the term they are typing based on the recommendation text.
- UI customization: Using templates, you may change how each recommendation looks when it’s shown.
- Responsive UI: Adaptive user interface (UI) created specifically for mobile devices that respond to touch.
Let’s See How to Use Autocomplete Library:
The jQuery AutoComplete plugin has been applied to the TextBox and the URL of the plugin is set to the AutoComplete Action method.
$(function (){ $("#txtEmployee").autocomplete({ source: function (request, response) { $.ajax({ url: ‘/Employee/GetEmployees/’, data: { "prefix": request.tern ), type: "POST", success: function (data){ response($.map(data, function (item) { return item; })) }, error: function (response) { alert(response.responseText); }, failure: function (response) { alert(response.responseText); } }); select: function (e, i) { $("#hfEmployee").val(i.item.val); }, minLength: 1 }); }); @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <br /><br /> <br /><br /> @ViewBag.Message } |
2. Conclusion
This article mentions the .Net libraries list as a resource for you to use while working on your next project. There are many .NET development firms available that are capable of delivering high-quality .NET core services. Looking for one? Contact us today!
FAQs
How to Install a .NET library?
To install a .NET library, one will have to download the installer and follow the steps given below-
- Run the installer.
- Provide a security key during the installation process.
- Configure the library.
- Restart the .NET application to start the tracing.
How to Create a Dotnet Library?
To create a .NET library, one needs to follow the below-given points –
- Run dotnet new classlib -o StringLibrary command in the terminal.
- To add the library project, run dotnet sln add StringLibrary/StringLibrary.csproj command.
- Now check whether the library target is .NET 7 or not. And for that go to Explorer and open StringLibrary/StringLibrary.csproj.
- Now, open the class.cs file and replace the code.
- Save the file
- Run the dotnet build command to build the solution.
Comments
Leave a message...